React Developer Tools
Use React Developer Tools to inspect React components , edit props and state , and identify performance problems.

You will learn
- How to install React Developer Tools
Browser extension
The easiest way to debug websites built with React is to install the React Developer Tools browser extension. It is available for several popular browsers:
- Install for Chrome
- Install for Firefox
- Install for Edge
Now, if you visit a website built with React, you will see the Components and Profiler panels.
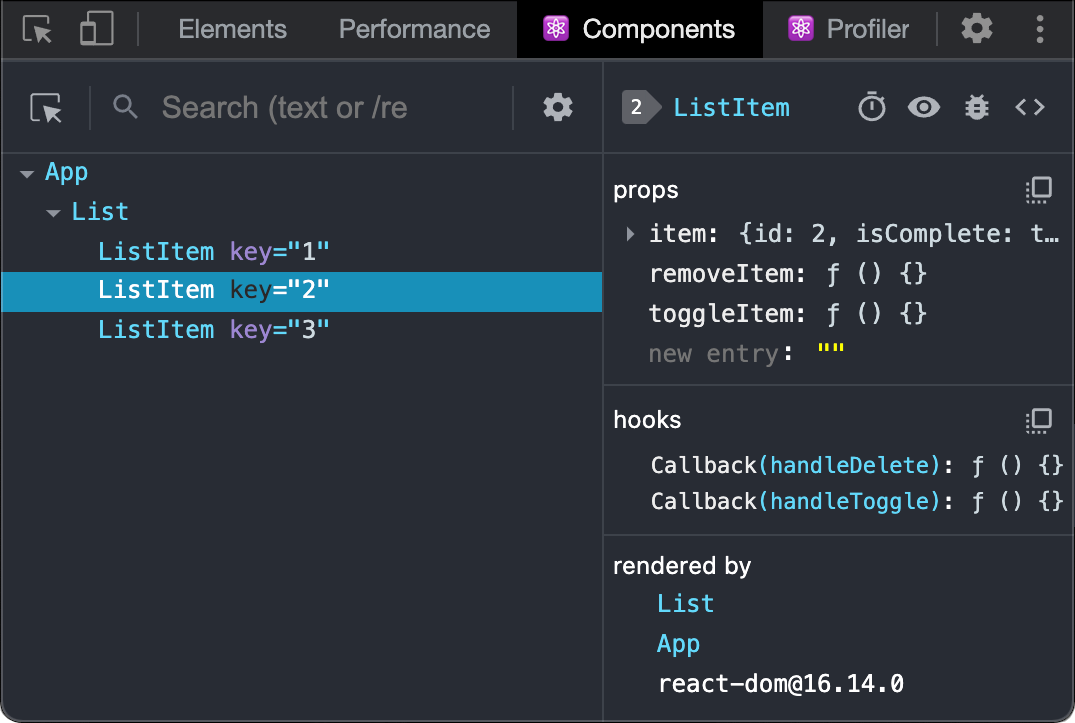
Safari and other browsers
For other browsers (for example, Safari), install the react-devtools npm package:
Next open the developer tools from the terminal:
Then connect your website by adding the following <script> tag to the beginning of your website’s <head> :
Reload your website in the browser now to view it in developer tools.
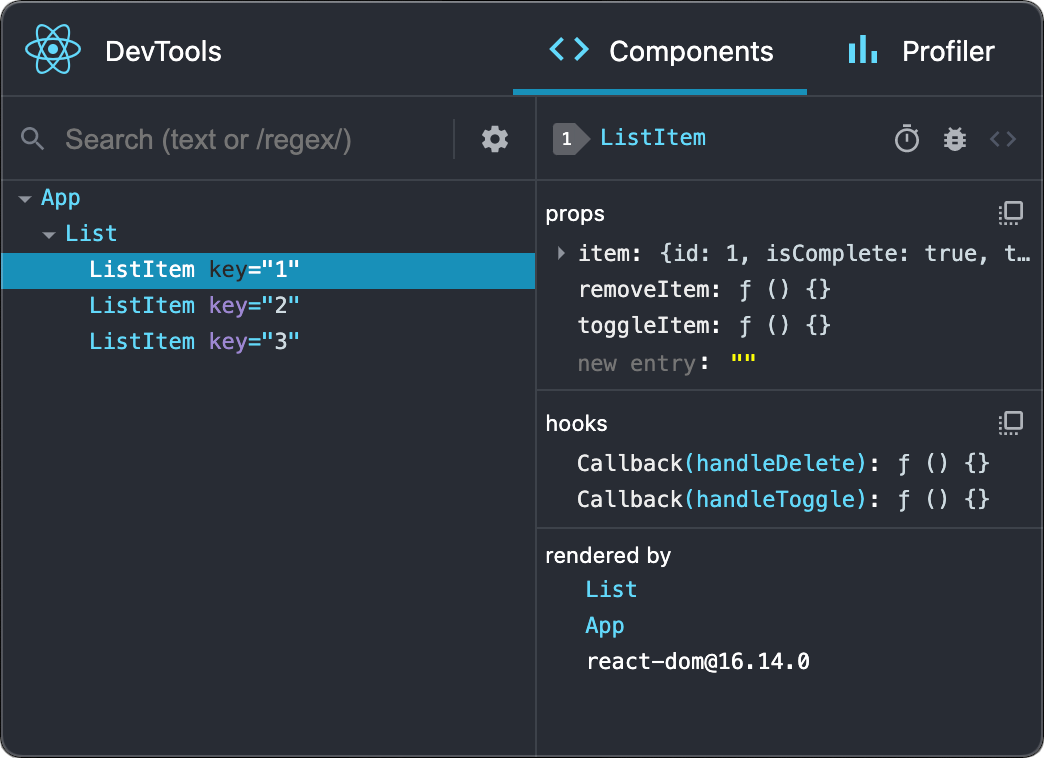
Mobile (React Native)
React Developer Tools can be used to inspect apps built with React Native as well.
The easiest way to use React Developer Tools is to install it globally:
Next open the developer tools from the terminal.
It should connect to any local React Native app that’s running.
Try reloading the app if developer tools doesn’t connect after a few seconds.
Learn more about debugging React Native.
How do you like these docs?
react-devtools
- 6 Dependencies
- 33 Dependents
- 150 Versions
This package can be used to debug non-browser-based React applications (e.g. React Native, mobile browser or embedded webview, Safari).
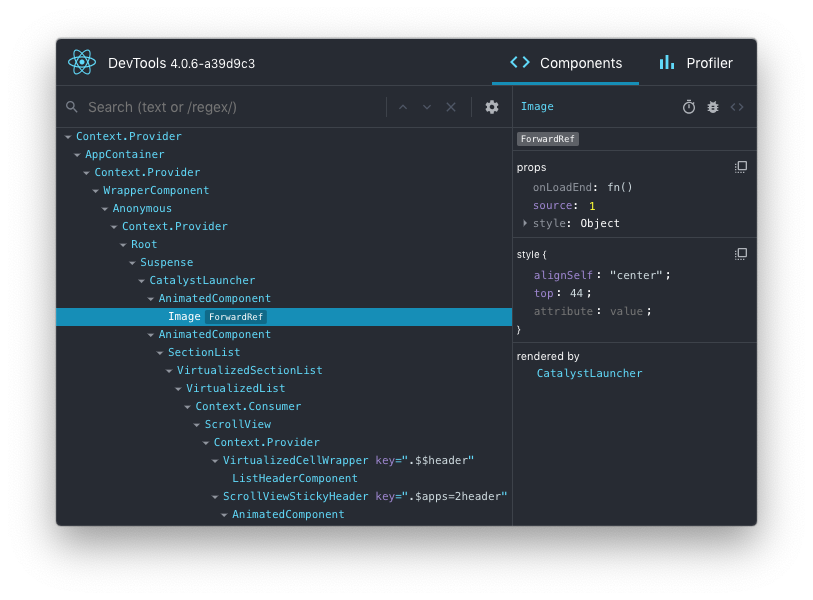
Installation
Install the react-devtools package. Because this is a development tool, a global install is often the most convenient:
If you prefer to avoid global installations, you can add react-devtools as a project dependency. With Yarn, you can do this by running:
With NPM you can just use NPX :
Usage with React Native
Run react-devtools from the terminal to launch the standalone DevTools app:
If you're not using a local simulator, you'll also need to forward ports used by React DevTools:
If you're using React Native 0.43 or higher, it should connect to your simulator within a few seconds. (If this doesn't happen automatically, try reloading the React Native app.)
Integration with React Native Inspector
You can open the in-app developer menu and choose "Show Inspector". It will bring up an overlay that lets you tap on any UI element and see information about it:
However, when react-devtools is running, Inspector will enter a special collapsed mode, and instead use the DevTools as primary UI. In this mode, clicking on something in the simulator will bring up the relevant components in the DevTools:
You can choose "Hide Inspector" in the same menu to exit this mode.
Inspecting Component Instances
When debugging JavaScript in Chrome, you can inspect the props and state of the React components in the browser console.
First, follow the instructions for debugging in Chrome to open the Chrome console.
Make sure that the dropdown in the top left corner of the Chrome console says debuggerWorker.js . This step is essential.
Then select a React component in React DevTools. There is a search box at the top that helps you find one by name. As soon as you select it, it will be available as $r in the Chrome console, letting you inspect its props, state, and instance properties.
Usage with React DOM
The standalone shell can also be useful with React DOM (e.g. to debug apps in Safari or inside of an iframe).
Add <script src="http://localhost:8097"></script> as the very first <script> tag in the <head> of your page when developing:
This will ensure the developer tools are connected. Don’t forget to remove it before deploying to production!
If you install react-devtools as a project dependency, you may also replace the <script> suggested above with a JavaScript import ( import 'react-devtools' ). It is important that this import comes before any other imports in your app (especially before react-dom ). Make sure to remove the import before deploying to production, as it carries a large DevTools client with it. If you use Webpack and have control over its configuration, you could alternatively add 'react-devtools' as the first item in the entry array of the development-only configuration, and then you wouldn’t need to deal either with <script> tags or import statements.
By default DevTools listen to port 8097 on localhost . If you need to customize host, port, or other settings, see the react-devtools-core package instead.
The React Tab Doesn't Show Up
If you are running your app from a local file:// URL , don't forget to check "Allow access to file URLs" on the Chrome Extensions settings page. You can find it by opening Settings > Extensions:
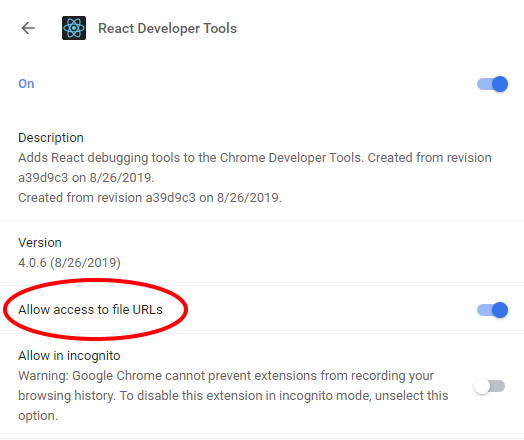
Or you could develop with a local HTTP server like serve .
The React tab won't show up if the site doesn't use React , or if React can't communicate with the devtools. When the page loads, the devtools sets a global named __REACT_DEVTOOLS_GLOBAL_HOOK__ , then React communicates with that hook during initialization. You can test this on the React website or by inspecting Facebook .
If your app is inside of CodePen , make sure you are registered. Then press Fork (if it's not your pen), and then choose Change View > Debug. The Debug view is inspectable with DevTools because it doesn't use an iframe.
If your app is inside an iframe, a Chrome extension, React Native, or in another unusual environment , try the standalone version instead . Chrome apps are currently not inspectable.
If your Components tab is empty, refer to "The React tab shows no components" section below .
If you still have issues please report them . Don't forget to specify your OS, browser version, extension version, and the exact instructions to reproduce the issue with a screenshot.
The React tab shows no components
The issue with chrome v101 and earlier.
As we migrate to a Chrome Extension Manifest V3, we start to use a new method to hook the DevTools with the inspected page. This new method is more secure, but relies on a new API that's only supported in Chrome v102+. For Chrome v101 or earlier, we use a fallback method, which can cause malfunctions (e.g. failure to load React Elements in the Components tab) if the JS resources on your page is loaded from cache. Please upgrade to Chrome v102+ to avoid this issue.
Service Worker malfunction
Go to chrome://extensions. If you see "service worker (inactive)" in the React Developer Tools extension, try disabling and re-enabling the extension. This will restart the service worker. Then go to the page you want to inspect, close the DevTools, and reload the page. Open the DevTools again and the React components tab should be working.
Local development
The standalone DevTools app can be built and tested from source following the instructions below.
Prerequisite steps
DevTools depends on local versions of several NPM packages 1 also in this workspace. You'll need to either build or download those packages first.
1 Note that at this time, an experimental build is required because DevTools depends on the createRoot API.
Build from source
To build dependencies from source, run the following command from the root of the repository:
Download from CI
To use the latest build from CI, run the following command from the root of the repository:
Build steps
You can test the standalone DevTools by running the following:
- First, complete the prerequisite steps above! If you don't do it, none of the steps below will work.
- Then, run yarn start:backend and yarn start:standalone in packages/react-devtools-core
- Run yarn start in packages/react-devtools
- Refresh the app after it has recompiled a change
- For React Native, copy react-devtools-core to its node_modules to test your changes.
Package Sidebar
npm i react-devtools
Git github.com/facebook/react
github.com/facebook/react#readme
Downloads Weekly Downloads
Unpacked size, total files, last publish.
13 days ago
Collaborators
- Software/ IT Staffing
- Project Management
- Technology Architecture
- Web Development
- Mobile Development
- App Development
- UI/ UX Design
- MVP Development
- SaaS Development
- API Development
- QA & Testing
- LabVIEW icon LabVIEW
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
Debug React apps with React Developer Tools
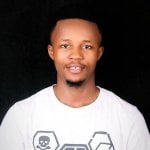
Editor’s Note: This article on debugging React applications with React Developer Tools was last revised on 8 February 2023 to reflect recent updates to React Developer Tools and include React Hooks-related features. To learn more about React Hooks check out our cheat sheet here .
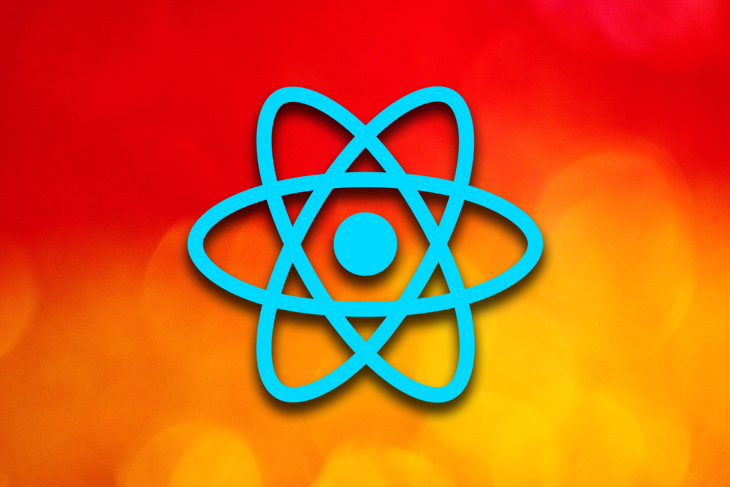
Debugging is one of the most useful skills a developer can possess. It allows you to navigate properly, spot errors in your code, and apply fixes quickly and efficiently to deliver production-ready, error-free, high-quality software systems. In the modern web development industry, this is made possible by using various tools and techniques.
React is one of the fastest-growing frontend development libraries. It makes creating complex and interactive UIs painless by offering a component-oriented UI development approach. Like other UI development frameworks and libraries, it has React Developer Tools, a debugging tool.
In this tutorial, I will explain how to use Rect Developer Tools’ latest features which came with the v4 .x (and later) releases, to debug your React applications with practical examples.
Jump ahead:
Introduction to React Developer Tools
React developer tools feature summary, how to install react developer tools, setting up our test application, react developer tools performance improvements, filtering components with react developer tools.
- Inline props in React DeveloperTools are now a thing of the past
Debugging unexpected prop values and component elements
Indented component view with no horizontal scrolling, improved searching, improved hooks support, restoring selection between reloads, higher-order components support, suspense toggle, reload and profile, component renders list, support for react developer tools.
React Developer Tools is a solution to inspect and analyze React components. It lets you edit component props and the state for debugging purposes. It also offers an inbuilt profiler for performance analysis. It comes as an official browser extension and an Electron-based standalone desktop app. The browser extension is available for Chrome, Firefox, and Edge. For Safari and other web browsers, the React development team offers the standalone Electron app via the r eact-devtools package.
React Developer Tools extends the browser’s native DevTools environment with several React-related debugging tabs. Similarly, the standalone React Developer Tools app offers the same browser-extension-like debugging experience.
Since the Developer Tools solution’s inception, there have been many releases from the core team. In this tutorial, I’ll highlight the notable features and demonstrate ways you can use its features to better debug your React apps productively.
Why should you use React Developer Tools?
Every modern web browser offers an inbuilt developer tools solution for developers to inspect and fix bugs in web apps. For example, you can add breakpoints and use the console to debug any JavaScript-based app. Also, you can inspect native DOM elements and attributes easily with the browser’s DevTools. React uses a virtual DOM with component instances and renders the real DOM during component rendering phases, so we can’t use inbuilt browser developer tools to debug React-component-specific things.
React Developer Tools offers a solution to debug your React apps by letting you inspect/manipulate React component internals, such as props and state. So, using React Developer Tools boosts your React app debugging tasks without using console.log browser-native workarounds.
Now, you know how the React Developer Tools solution helps debug React apps and why the web browser’s built-in debugger is inadequate for productively debugging React apps. Look at the following highlighted features that React Developer Tools offer:
- Inspecting the React component tree and seeing the reflection in the browser
- Searching and filtering components in complex apps
- Inspecting and editing props and state real-time to see how components behave
- Ability to find component owners (aka parents and ancestor components) in unfamiliar apps
- Offers a way to suspend a React component and test the fallback component
- Offers a fully-featured profiler that comes with the flame graph and ranked graph to implement performance enhancements
- Supports exporting and importing profiler results for collaborative debugging requirements (No need to share profiler screenshots/recodings with teammates)
React Developer Tools is available as an extension for modern popular browsers. Install the extension using the following links:
- Google Chrome
- Mozilla Firefox
- Microsoft Edge
If you have already installed the extension, it should update automatically whenever the React team publishes a new version. If you use React Native, Safari, or another web browser, you can install the standalone version from npm :
Let’s create a test app to get started with React Developer Tools debugging. I created a starter project for easy setup and to reduce overhead, ensuring the article focuses on debugging. The skeleton of the application has already been set up. It includes a few components, styling, and project structure.
Run the following command to clone the repository if you want to experiment with me:
Then, open the folder and install the project’s dependencies by running the following command:
To start the React app development server, run the npm start or yarn start command in the root folder of your project. Open your browser to localhost:3000, and you will see the project live! Before checking newly introduced React Developer Tools features, try the basics you already know to get familiarized with our test app. For example, React elements tree is as follows:
According to the React Developer Tools internal design documentation , the legacy DevTools solution rendered the component tree and internal properties in a less-efficient strategy that involves many JSON serialization events, so debugging complex React apps had performance bottlenecks from the DevTools perspective.
The new DevTools solution offers significant performance gains and improved navigation and inspection experience by transporting optimized component tree data and props on demand. These performance enhancements motivate developers to productively use React Developer Tools in larger apps.
In previous versions of DevTools, navigating through large component trees has been a bit tedious. But now, DevTools provides a way to filter components so that you can hide ones you’re not interested in. To access this feature, let’s filter through the three components in our sample application. Open your DevTools, and you will see our three components listed:
Click the setting icon below the components tab to filter out a component and focus on the one we are interested in. You’ll be presented with a popup that consists of several tabs. Click the components tab and choose your preferred filtering option.
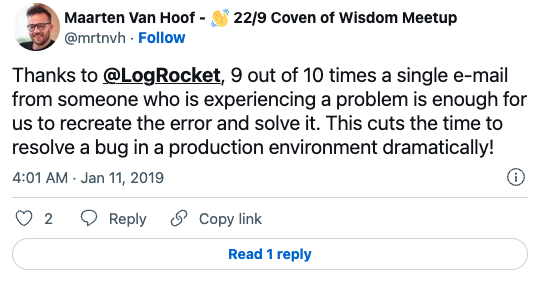
Over 200k developers use LogRocket to create better digital experiences

By default, the component tree shows all React components. You can add a filter via the component tab and hide components that match Pe regular expression to exclude Persons and People components from the tree:
After we filter out components, they become hidden by default but will become visible after disabling the filter. This feature will come in handy when you work on a project with many components and fast sorting becomes a real need. What’s even more interesting about this feature is that filter preferences are remembered between sessions, so you don’t need to re-add your favorite component filters during each debugging session.
You can add multiple filters and exclude elements based on component name, type, location, and higher-order components (HOC) . Also, you can use advanced regular expressions. For example, the following filter hides all heading DOM elements ( <h1> ) and Contacts React components:
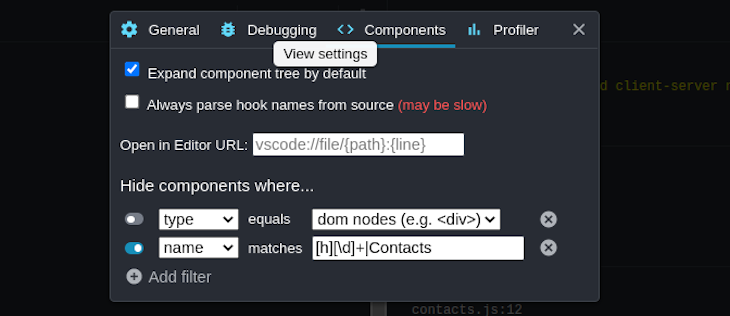
Inline props in React Developer Tools are now a thing of the past
To make larger component trees easier to browse and to make DevTools faster, components in the tree no longer show inline props . To see this feature in action, all you need to do is select a component and all the components’ props, state, and Hooks will be displayed on the right-hand side of the React Developer Tools components tab.
In our sample application, we only pass props to our Contacts component. Clicking it will reveal the value of the props passed to it, and clicking the other components will reveal that no props were passed to them. Look at the following preview:
Although viewing props from DevTools may not be so useful for smaller React projects since props are easily inspectable through the code, it will come in handy when working with larger React projects that consist of complex component trees and props. However, the props and state editing feature helps debug even small-scale apps productively:
In React component handling, a component’s owner refers to the component that renders it. On the other hand, parent refers to the upper-level component of the component tree. The parent component is not always the owner. Let’s understand it with an example. Rename the App component to App_original and add the following code:
Container is the parent of Panel , but App is the owner of the component, and only owners can send down props. In the newer version of React Dev tools, you can quickly debug an unexpected prop value by skipping over the parents and finding the owner that initiated the unexpected prop value. DevTools v4 adds a rendered by list in the right-hand pane that allows you to quickly step through the list of owners to speed up your debugging process.
By clicking the Panel component from the component tree, you can see where the color prop came from by browsing the rendered by list:
This is very useful when tracing back a particular prop’s origin. It also comes with an inverse function called owners tree . It is the list of things rendered by a particular component — the things it owns. This view is kind of like looking at the source of the component’s render method and can be a helpful way to explore large, unfamiliar React applications. The owners tree typically appears before the component tree UI.
To use this feature to debug our application, double-click a component to view the owners’ tree and click the x button to return to the full component tree. You can also move around in the tree to see all the children of the component. Rename App_original to App , get our contacts app back, and check the owners tree as follows:
Visual improvements in React Developer Tools
Carefully designed UI/UX concepts directly affect the quality of software systems. As a result, every software development team typically hires at least one UI/UX developer or more. UI/UX principles-based visual improvements may not always come with the first release of a particular app — the UI/UX team typically research, experiment, analyze, and implement new visual improvements to boost app users’ productivity and improve the app’s usability aspects.
Before v4 of React Developer Tools, some UI/UX-related issues affected debugging productivity and overall debugger’s usability (especially while debugging large React apps). So, in v4, the React development team introduced the following developer-friendly visual improvements.
In the previous versions of React Developer Tools, deeply nested components require both vertical and horizontal scrolling to see, which makes tracking large component trees difficult. DevTools now dynamically adjust nesting indentation to eliminate horizontal scrolling.
To use this feature in our app, click the components tab, then click any component . All its children will automatically appear below it with an automatic indentation from the next component. It’s impossible to demonstrate this with our sample contacts app since it’s a simple app that doesn’t contain deeply nested elements. However, you can see the dynamic indentation if you open React Developer Tools for the o fficial React documentation :
Previously, when searching in DevTools, the result is often a filtered components tree showing matching nodes as roots. Other components are hidden, and the search match now displays as root elements. This made the overall structure of the application harder to reason about because it displayed ancestors as siblings. Now, you can easily search through your components with inline results similar to the browser’s find-in-page search:
Functional improvements in React Developer Tools
As mentioned before, visual improvements increases React developers’ productivity during debugging. A good UI/UX isn’t the only fact that makes a software system great — app functionality (or features) also increases a particular software product’s quality. Early React Developer Tools versions weren’t fully-featured and didn’t align properly with modern React library features, for example, Suspense, Hooks, and more. In v4, the React team introduced the following functionalities to debug React apps that use the latest, advanced React library concepts.
Hook-based React projects can be debugged faster and better because Hooks in v4 now have the same level of support as props and state. Values can be edited, and arrays and objects can be drilled into. Open the sample app, select the App component in DevTools, look at the Hooks area, and try to edit state, as shown in the following preview:
While debugging, if you hit reload , DevTools now attempts to restore the last selected element. Let’s assume we were sorting through the Persons component in our sample application before a page refresh occurs, DevTools will resume with the Persons component automatically selected:
Experienced React developers often tend to use advanced development techniques while developing apps. Higher-order components (aka HOC) is an advanced React component composition technique that implements a function to take a component and return a modified component. React Developer Tools v4 displays HOC badges as it displays forwardRef , Memo -like badges. Let’s check HOC support in DevTools.
First, add the following function to your App.js file:
The withMoreProps HOC adds x and y props to the input component. Modify the Persons component with withMoreProps HOC as follows:
Check DevTools. Now you can see the HOC label and newly added props:
React’s Suspense API lets components wait or do something before rendering. <Suspense> components can be used to specify loading states when components deeper in the tree are waiting to render. DevTools lets you test these loading states with this toggle:
Profiler changes in React Developer Tools
In the programming field, a profiler refers to a software program that analyzes another software program’s source dynamically. The dynamic analysis process helps developers identify performance issues in their apps. For example, if a web app works slowly in certain conditions, web developers can use a profiler to identify performance bottlenecks. React Developer Tools offers the profiler program in a separate tab in the browser extension and the standalone app.
React Developer Tools Profiler is a powerful tool for performance-tuning React components. Legacy DevTools supported profiling but only after it detected a profiling-capable version of React. Because of this, there was no way to profile an application’s initial mount (one of the most performance-sensitive parts). This feature is now supported with a reload and profile action:
The profiler displays a list of each time the selected component is rendered during a profiling session, along with the duration of each render. This list can be used to quickly jump between commits when analyzing the performance of specific components. For example, profile the sample app and click a component to see how many times a particular component was rendered as follows:
Only the following versions of React are supported:
- v0-14.x : Not supported
- v15.x: Supported (except for the new component filters feature)
- v16.x and newer versions: Supported
React Native
- v0-0.61 : Not supported
- v0.62 and newer versions: Supported
So if certain features don’t work on a particular project, check the React version you are using. It’s always good to use the latest React version for new projects or upgrade React version in existing projects to use the latest library features and receive performance or security enhancements.
In this tutorial, we have talked about debugging React applications with DevTools. We looked at some additions and improvements that came with it. We also looked at how they make debugging your code easier.
Try debugging your React apps with the features we’ve discussed in this article. You can check this live playground app to learn how to debug React apps with DevTools without installing the browser extension or the standalone app. Here is the summary that you need to remember before debugging React apps:
- Use the element tree (the component tab) to browse components, edit props, and state
- Use the profiler to identify performance issues and analyze for performance enhancements (for example, time to interactive ( TTI ) optimizations for SEO and usability)
If you have any questions, comments, or additions, be sure to drop a comment. Happy coding!
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
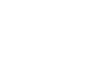
Stop guessing about your digital experience with LogRocket
Recent posts:.
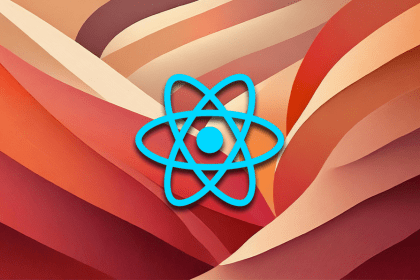
Comparing React state tools: Mutative vs. Immer vs. reducers
Mutative processes data with better performance than both Immer and native reducers. Let’s compare these data handling options in React.
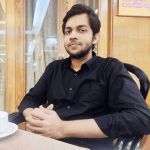
Radix UI adoption guide: Overview, examples, and alternatives
Radix UI is quickly rising in popularity and has become an excellent go-to solution for building modern design systems and websites.
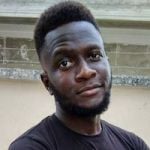
Understanding the CSS revert-layer keyword
In this article, we’ll explore CSS cascade layers — and, specifically, the revert-layer keyword — to help you refine your styling strategy.
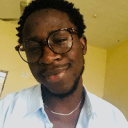
Exploring Nushell, a Rust-powered, cross-platform shell
Nushell is a modern, performant, extensible shell built with Rust. Explore its pros, cons, and how to install and get started with it.
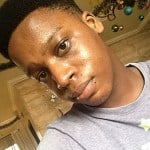
2 Replies to "Debug React apps with React Developer Tools"
Great article, well done.
Great article! When a component is clicked in “rendered by” section on right, is there a way to go back to the previous component ?
Leave a Reply Cancel reply
React DevTools 101: Master Debugging with This Essential Tool
Introduction to react devtools.
React DevTools provides invaluable debugging and profiling capabilities for React developers. With React DevTools, you can inspect React components right in the browser DevTools panel. This allows tracking down bugs faster and optimizing performance. This guide will overview the key features of React DevTools and demonstrate how it helps debug React apps through real-world examples. By the end, you'll have the skills to master debugging your own React apps with DevTools.
Let's start by installing React DevTools and accessing it through the browser.
Installing and Accessing React DevTools
React DevTools is available as a browser extension for Chrome and Firefox. You can install it from the Chrome Web Store or Firefox Add-ons site.
Once installed, a new "React" tab will appear in your browser's Developer Tools panel. To open DevTools, use the keyboard shortcut Ctrl + Shift + C (Cmd + Shift + C on Mac). The React tab is where you'll access all the React debugging features.
For example, I installed the React DevTools extension in Chrome. When I inspect my example React app and open the Chrome DevTools, I see the React tab alongside the other panels like Elements and Network. This is where I can start inspecting and debugging my React components.
Inspecting React Components
The Components panel in React DevTools shows the component tree for your React application. You can click on any component to view additional details like props, state, and hooks.
The highlighting tool is great for visualizing which components are actually rendering. I can hover over a component in the tree view and DevTools will highlight it on the page. This helps trace whether components are rendering as expected.
Filtering components is also handy. I can search for a specific component by name or filter the tree to only show components rendering under a certain folder path. When debugging a large app, this keeps the view focused.
The timeline tool in React DevTools is invaluable for inspecting component updates. It shows detailed information on which components re-rendered during a given timeframe. I can replay renders to watch component updates live. This helps pinpoint components that may be re-rendering too frequently.
For example, while profiling my app I noticed the Navbar component was re-rendering constantly on scroll events even when its props weren't changing. The timeline tool helped me identify this performance bottleneck.
Examining State and Props
React DevTools makes it easy to inspect the props and state values for any component.
I can select a component in DevTools and view its props and state in the panel sidebar. Interactively editing these values is an easy way to test different scenarios. My changes even persist across page reloads, which accelerates development.
The props and state history navigator allows "time travel" debugging. I can view previous values and step through changes turn-by-turn. This is invaluable when tracking down bugs related to state.
I also leverage the search feature frequently. I can search for components that contain specific props or state values. This comes in handy when trying to locate the source of stale state bugs.
Pinning components persists their state which is handy for important debugging scenarios. For example, I'll pin my Redux store component to keep monitoring state changes.
Debugging with React DevTools
React DevTools unlocks powerful JavaScript debugging techniques tailored for React.
Setting breakpoints allows pausing execution to inspect component values in the middle of rendering. I can also step through the code line-by-line to isolate issues.
The call stack tool provides insightful stack traces. I can view the chain of function calls leading up to errors or slow renders. This context helps narrow down the source of bugs.
Logging custom debug messages to the console from my React components makes debugging straightforward. I can track variables, function calls, and more.
There are also React-specific tips like checking for unnecessary re-renders due to parent components passing new props references unnecessarily. React DevTools points out these optimizations.
For example, I had a bug where state was getting overwritten unexpectedly. By setting a breakpoint in componentDidUpdate and examining the call stack, I was able to pinpoint where the incorrect state update was occurring. React DevTools cut down hours of debugging time.
Profiling with the Profiler
Optimizing performance is crucial for React apps. The Profiler provides detailed performance metrics to identify optimization opportunities.
The Profiler measures how long each render phase takes for components. I can record traces to visualize components that are slowing things down.
Flame charts help highlight expensive operations like excessive state updates. I can dig into specific components to understand what’s causing sluggish renders.
The Profiler integrates nicely with the Chrome Performance tab for low-level profiling. I can compare performance across app versions to validate optimizations.
For example, I noticed my app animation was janky during scroll events. The Profiler showed that an expensive DOM measurement was happening on each scroll. By memoizing the measurement into a variable, I reduced the time spent rendering substantially.
<a href="https://devhunt.org" style="font-weight:bold;color:blue;">Check out DevHunt to discover more innovative developer tools like React DevTools →</a>
Advanced Usage of React DevTools
React DevTools offers advanced capabilities for power users. Let's explore a few highlights.
Customizing Components in DevTools
For rapid testing, I can modify components directly in React DevTools. Changes like swapping prop values or state persist across page reloads.
Overriding CSS styles comes in handy for quickly mocking layouts and themes. I can tweak margins, colors, and more.
For Redux apps, overriding the Redux state from the DevTools panel accelerates development. Mocking API response data avoids roundtrips while testing.
These customizations streamline development by removing friction. I save hours not having to recompile code to test every change.
Integrating with React Native
Debugging React Native apps works similarly to web apps with a few extra steps.
I can open the DevTools overlay by shaking the device or pressing the menu button. Here I can inspect views, profile performance, and debug JS code.
Setting up remote debugging allows full integration with my machine's Chrome DevTools. There is some initial setup, but the workflow is smooth.
Being able to access the full React DevTools capabilities for React Native apps is invaluable. The integration may take more work, but unlocks next-level debugging powers.
Mastering React DevTools is a gamechanger for React developers. The ability to inspect components, view state, debug code, and profile performance unlocks next-level app development, debugging, and optimization.
While it takes time to learn all of React DevTools' capabilities, integrating it into your workflow is worth the effort. The tools feel like they were made specifically for React.
Hopefully this overview has shown that React DevTools lives up to its tagline as an “essential” tool for React. Master it, and you’ll be able to debug even the most complex React applications with confidence.
Debugging Tests
There are various ways to setup a debugger for your Jest tests. We cover debugging in Chrome and Visual Studio Code .
Debugging Tests in Chrome
Add the following to the scripts section in your project's package.json
Place debugger; statements in any test and run:
This will start running your Jest tests, but pause before executing to allow a debugger to attach to the process.
Open the following in Chrome
After opening that link, the Chrome Developer Tools will be displayed. Select inspect on your process and a breakpoint will be set at the first line of the react script (this is done to give you time to open the developer tools and to prevent Jest from executing before you have time to do so). Click the button that looks like a "play" button in the upper right hand side of the screen to continue execution. When Jest executes the test that contains the debugger statement, execution will pause and you can examine the current scope and call stack.
Note: the --runInBand cli option makes sure Jest runs test in the same process rather than spawning processes for individual tests. Normally Jest parallelizes test runs across processes but it is hard to debug many processes at the same time.
Debugging Tests in Visual Studio Code
Debugging Jest tests is supported out of the box for Visual Studio Code .
Use the following launch.json configuration file:
- Debugging Tests in Chrome
- Debugging Tests in Visual Studio Code
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
react-devtools for Safari #67
oveddan commented Feb 4, 2015
- 👍 150 reactions
- 😄 4 reactions
- 🎉 3 reactions
- ❤️ 23 reactions
adbl commented Feb 9, 2015
Sorry, something went wrong.
nmn commented Feb 23, 2015
- 👍 2 reactions

michaeltroy commented Mar 2, 2015
ikurennyi commented May 18, 2015
sophiebits commented May 18, 2015
- 👎 72 reactions
- 😕 28 reactions
jaredly commented Oct 15, 2015
- 👍 1 reaction
TylerBrock commented Dec 31, 2015
- 👍 112 reactions
iamdustan commented Dec 31, 2015
rustanacexd commented Feb 17, 2016
kassens commented Feb 17, 2016
freddyrangel commented Sep 15, 2016
- 👍 35 reactions
voxelshaper commented Dec 10, 2016
taime commented Jan 24, 2017
DomVinyard commented Apr 28, 2017
sunlee-newyork commented May 7, 2017
joshhepworth commented May 11, 2017 • edited
- 👍 12 reactions
ericdfields commented Jun 26, 2017
rensftw commented Aug 4, 2017
Domvinyard commented aug 16, 2017.
kristaponis commented Oct 28, 2017
jhessin commented Nov 12, 2017
TheTourer commented Dec 9, 2017
raphael-thibierge commented Dec 11, 2017
Aarbel commented Dec 27, 2017
- 👍 8 reactions
zcaceres commented Jan 15, 2018
cristiandan commented Jan 26, 2018
gastonmorixe commented Jan 28, 2018
mdavies-solsys commented Feb 21, 2018
ezfe commented Feb 24, 2018
ghost commented Feb 27, 2018
jasonwoodland commented Mar 2, 2018
giangle-datadive commented Apr 1, 2018
marciotoze commented Apr 12, 2018
daniel-gato commented Apr 30, 2018
dascheaten commented May 3, 2018
hurvajs77 commented May 6, 2018
visuallization commented May 11, 2018
yeyuguo commented Jun 13, 2018
ur92 commented Jun 13, 2018
nisevi commented Aug 12, 2018
maxkohl88 commented Aug 20, 2018
suberg commented Aug 29, 2018
jgabriele commented Sep 6, 2018
J-Liu commented Sep 7, 2018
bupy7 commented Sep 7, 2018
dkassen commented Sep 25, 2018
flyinhigh commented Oct 23, 2018
camilo86 commented Oct 29, 2018
marcoroth commented Nov 16, 2018
seepls commented Jan 15, 2019
superandrew commented Apr 18, 2019
tinegrunau commented Apr 25, 2019
mikelyons commented Jun 12, 2019
aapelismith commented Sep 2, 2019 • edited
No branches or pull requests
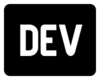
DEV Community

Posted on Oct 12, 2021 • Updated on Nov 4, 2022
How To Debug a React App in VSCode
UPDATE : I released a npm package create-chrome-debugger that creates a Chrome Debugger shortcut to start Chrome in Remote Debugging Mode.
When developing a React app (or any JavaScript app), I heavily use console.log() for debugging purposes if something is not running as expected. Only if it's really tricky, I use the VSCode debugger. It's not that I don't like debuggers, debugging JS is just not as convenient as it is in other programming languages.
Usually, the React app is started with npm run start/yarn start (react-scripts start) and it runs on localhost:3000 and hot reloads when making file changes. A new Chrome tab is opened by React and I just keep this tab open forever. If I need to check the value of a certain variable, I log it to the console and check the output on Chrome Dev Tools.
On the other hand, VSCode offers two debugging options for JS apps: Launching the debugger with a new browser window or attaching the debugger to the already running app on a certain browser window/tab. Until now, I was only using the first option of launching a new window because I wasn't able to get the second option working. Unfortunately, launching a new browser means you have to navigate to the page you actually want to debug and you lose all your state (e.g. form inputs). So effectively you end up with two instances of the same app. And that's the reason I didn't use the debugger in the first place.

However, I didn't want to accept that issue any longer and decided to figure out what I'm missing. Here are my findings!
Start React App with Remote Debugging
In order to debug JS apps, the browser has to be started with remote debugging enabled. For example, Chrome has to be started with the flag --remote-debugging-port=9222 . When you click debug on VSCode it does exactly that: It starts a new browser window with this command line argument. Unfortunately, the default React start script launches a browser without remote debugging (maybe for safety reasons?). However, the advanced configuration allows us to change the browser and how it is launched by setting two environment variables BROWSER and BROWSER_ARGS :
The name of the browser depends on the operating system. For example, Chrome is google chrome on macOS, google-chrome on Linux and chrome on Windows.
You have to close Chrome completely before running this script. If Chrome is already running on your system, this React start script will not create a new window but will create a new tab on your existing Chrome window. My assumption is that you enable remote debugging on process level and if you already started Chrome by clicking on the icon, remote debugging is not enabled by default. Then, when React wants to launch a new window, Chrome internally checks if it can use an existing window or if it has to create a new window. In my case, it re-used the existing Chrome window and didn't enable remote debugging. I guess that's also the reason I was not able to attach the VSCode debugger to my running React app.
Start Chrome Always with Remote Debugging
Another more convenient option is to start Chrome always with remote debugging enabled. On Windows it's straightforward as you just have to right-click on the Chrome shortcut, go to properties and append the above command line argument to the target field like this: C:\Program Files (x86)\Google\Chrome\Application\chrome.exe --remote-debugging-port=9222 -- "%1"
On macOS it's trickier. I've found two options on the Internet that I will include in the following. In both cases you end up with a new Chrome app that you can add to the dock to replace the original app. It's a kind of shortcut that will simply start Chrome with command line arguments.
Create a Custom Chrome App
This answer on StackOverflow describes how to create a custom Chrome app only with a text editor. It also includes a nice debug icon that you can use to distinguish the custom app from the original app.
Using Automator
There's another solution by Dave Shepard on how to use Automator to create a custom Chrome app. Automator is a macOS standard tool to create apps and workflows composed of multiple actions. In my case, I created an app that runs a shell script to start Chrome with remote debugging enabled. Please refer to the original blog post for detailed instructions.
Debugging in VSCode
Now that we have Chrome up and running with remote debugging enabled, we can head back to VSCode for debugging the React app. VSCode manages its debug configurations in the file .vscode/launch.json :
There are two debug configurations. The first will create a new Chrome window with remote debugging enabled on the url http://localhost:3000 , while the second will attach the debugger to an existing Chrome window. The important things I'd like to mention here are the port and the urlFilter properties. The port number must match the remote debugging port from the command line argument above (it's not the port on which the development server runs, e.g. 3000). The url filter expression will search for a page with this url.
In VSCode we can simply put a breakpoint in our component which is currently being rendered in the browser and then click debug Attach to Chrome . The breakpoint will break on the next re-render of the component and we don't have to navigate on a new browser window.
Top comments (5)
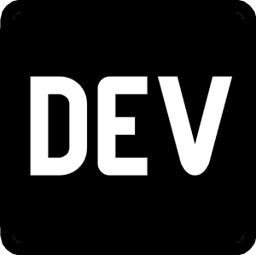
Templates let you quickly answer FAQs or store snippets for re-use.

- Joined Jul 12, 2018
how to debug in safari on macOS?

- Work Co-Founder at Flyweight.io
- Joined May 16, 2021
Unfortunately, I couldn't find any information if it is possible to start Safari in remote debugging mode (Safari on iOS seems to be possible).
thanks for your time, finally I had to install edge for this
Any reason you don't use Chrome? I made a short video that shows how to create a custom Chrome shortcut that automates the whole remote debugging setup. It's basically just a npm package.

- Joined Sep 23, 2020
This eloquent explanation was missing! Thank you :)
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
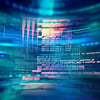
Learn React Hydration in 5 minutes
LordCodex - Apr 22
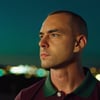
How to install React 19 Beta
Gonçalo Alves - Apr 26
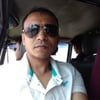
1289. Minimum Falling Path Sum II
MD ARIFUL HAQUE - Apr 26
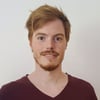
Path To A Clean(er) React Architecture - API Layer & Fetch Functions
Johannes Kettmann - Apr 26
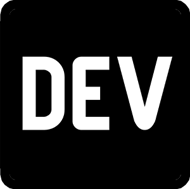
We're a place where coders share, stay up-to-date and grow their careers.
Boost Your Debugging Productivity How To Debug React Apps With VS Code
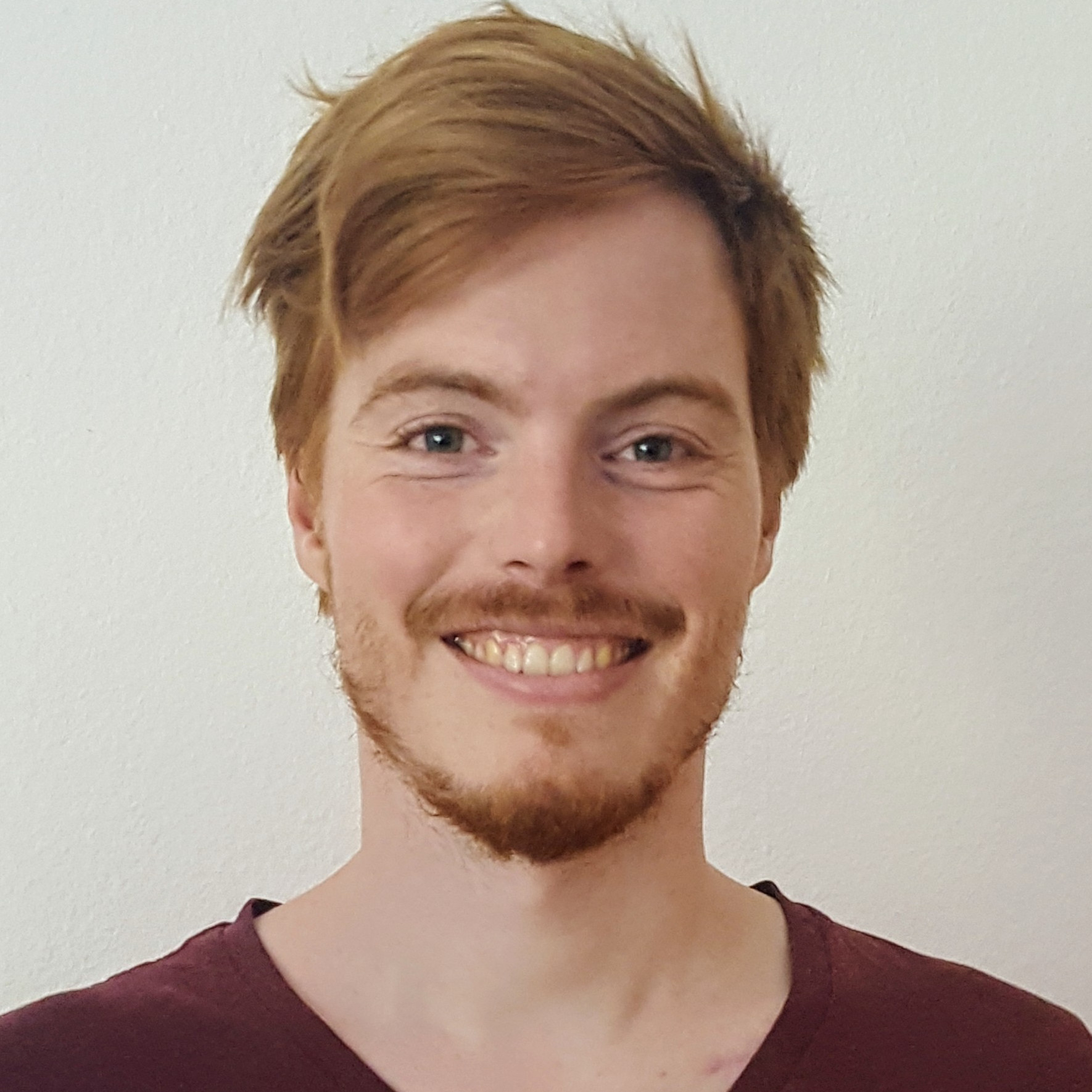
Debugging a React app can be a slow and painful process. Adding a console.log() statement here and there until you’re finally in the right spot. Or a more advanced version: Jumping around between setting breakpoints in the Chrome dev tools and editing code in your IDE until the bug is fixed.
But with the right tools and a strategic approach debugging can become much easier. Maybe even fun?!
Turns out much beloved VS Code makes it very simple to debug a React app directly from the IDE. The result: Super easy setup and a more productive debugging workflow.
On this page, you can see how to set up VS Code as a debugger for your React app and see it in action . We’ll debug a small problem with a Next.js application and use (conditional) breakpoints, step into functions, and inspect and edit variables directly from the VS Code. All of this paired with a structured debugging approach and the bug is fixed in no time.
Here’s a short video showing how to set up and use the VS Code debugger. Alternatively, you can find a detailed step-by-step tutorial with screenshots on this page.
Launch Chrome via VS Code
Starting to debug your React app with the VS Code debugger is surprisingly simple. You let VS Code create a launch.json config for you and slightly adjust it. You can find the file in the .vsocde folder in your repository.
Depending on your app you need to
- adjust the url field (here I change the port to 3000)
- adjust the webRoot entry (e.g. if your code is in the src folder like create-react-app apps you change ${workspaceFolder} to ${workspaceFolder}/src )
Now you can hit the play button ▶️ to start a Chrome browser in debug mode. The VS Code debugger is automatically attached to this browser.
Note: You could run Chrome in debug mode manually and use the “attach” launch config to attach the VS Code debugger. But I don’t see a reason to overcomplicate things.
If you already have a launch.json file or want to add another config to it you can simply use Intellisense (e.g. by pressing cmd + space on Mac) and get suggestions.
Here is my launch.json file:
Using The VS Code Debugger
Two use the debugger you need two things:
- Run your React app (e.g. by running npm start ).
- Start Chrome via VS Code by pressing the play button ▶️.
You can now use the debugger as you would in the Chrome dev tools. If you’re not familiar with that you can see an example below.
The VS Code Debugger In Action
Let’s imagine the following situation: We’re a developer working on a Next.js app that is in production. Real users, nothing should break.
One of the most important pages used to look like this ( you can see a deployed version of it here ):
But suddenly we get reports that something is broken ( here a deployed version ):
Damn, how did this bug make it into production? Our customers are upset. They’re paying good money and rely on our app. Obviously, our managers are going crazy as well.
But we as the developers stay calm. With the right debugging approach we can probably fix this bug in no time.
The error message in production doesn’t tell us a lot. So first we run the app on our local machine.
Since we know that we need to debug the code we use the VS Code debugger to start a Chrome instance.
This opens the browser at localhost:3000 as defined in launch.json.
Now when we navigate to the problematic page we see this error.
- a production-grade code base
- realistic tasks & workflows
- high-end tooling setup
- professional designs.
Using Breakpoints in VS Code
This error is much better than what we see on the production website. It provides us with two important pieces of information:
- The error message “Cannot read properties of null”.
- The name of the file and the line where the error occurred: line 49 in issue-row.tsx .
Opening the file in VS Code is really simple. The file path in the error message is in fact a link:
When we click it, the file issue-row.tsx opens in VS Code. Magic.
The error message tells us that the issue prop is likely null at some point. Let’s verify that by adding a breakpoint. Simply click inside the empty space next to the line number.
Once you press the green “Restart” button ↻ the page refreshes.
To speed up the process you can also use a key combination (Cmd + Shift + F5 in my case). You can hover over the button to find out yours.
The code execution stops at the breakpoint. At the same time, the website freezes in its loading state.
As you can see in the screenshot above the issue prop is defined during the first render.
So we press the “Continue” button ⏯️ or F5 a couple of times. The problem is that there are quite a few IssueRow components being rendered. So hitting “Continue” until we find the right issue becomes quickly annoying.
Conditional Breakpoints To The Rescue
Instead of hitting “Continue” all the time, we’d like to skip all the issues that are defined and only stop at the nullish one.
The easiest way to do that is by adding a condition to the breakpoint. Right-click on the breakpoint and select “Edit Breakpoint”.
Now we can enter a JavaScript expression. In our case, we want to stop at issue === null .
We hit enter and continue code execution. And voila we can confirm that at least one issue is null .
To be honest, this isn’t exactly news to us as we already knew this from the error message. But at least we were able to confirm the problem.
Finding The Root Cause
So let’s dig a bit deeper. In a simple JavaScript program, we could just follow the call stack or press the “Step Out” button (⬆️) to find the root cause of the problem.
But with React (and other frameworks) it’s not that easy:
Except the IssueRow we can’t see any of our code files in the “Call Stack” panel. Everything else is internal React files.
This doesn’t help us much.
So, unfortunately, we need to find out where the IssueRow component is rendered manually. The global search function of VS Code is our best friend here.
We open the file and add another breakpoint just before the return statement of the component.
Then hit the refresh button ↻ in the debug controls or F5.
Great, now we see that one item in the items array is indeed null .
Inspect And Edit Variables In The Debugger
Our assumption is that this null value causes the bug. But before we start messing around with our code we can easily verify this assumption by editing the variable inside the debugger.
When we continue the code execution now we can see that the error disappears on the website. The 7th and 8th items in the issue list are now duplicates as expected.
This verifies our assumption that the null value in the data array is the problem.
That makes the solution to our bug simple: we can just filter out all null values from the data to fix the bug. Of course, we could implement the filter function in the component. But maybe a place closer to the data source would be more suitable. This way other (future) components could potentially benefit from the same fix.
Step Into A Function
To dig a bit deeper we have a look at the beginning of the component. The items array with the null value comes from the issuePage variable. And that one comes from a hook.
So let’s set another breakpoint there and hit the refresh button again. Code execution stops at the breakpoint.
Now we can use the “Step Into” button ⬇️ to investigate that hook. The first file that opens is again some internal React file.
But this time we’re better off. After hitting the “Step Into” button a couple of times we end up in the useIssues hook.
Having a closer look the getIssues function seems like a good candidate to filter the data.
Unfortunately, for some reason, we neither can step into the getIssues function nor does code execution stop at a breakpoint inside. If you have a bit of debugging experience you know that there are inconveniences like this from time to time.
Anyway, let’s not allow that to hold us back. We add a bit of code to filter the issues.
Once we hit return we can confirm that the bug is fixed.
Commit, push, deploy. Everyone’s happy.

IMAGES
VIDEO
COMMENTS
The easiest way to debug websites built with React is to install the React Developer Tools browser extension. It is available for several popular browsers: ... Install for Edge; Now, if you visit a website built with React, you will see the Components and Profiler panels. Safari and other browsers . For other browsers (for example, Safari ...
4. Starting with MacOS 13.0 Ventura and Safari 16.1, developers can now build and distribute Extensions for Safari Web Inspector, just like they do with Safari as a whole through the App Store. It is now hopefully a matter of time before Facebook or someone else builds and distributes React Dev Tools for Safari.
Learn how to use React DevTools in Safari! In this tutorial, we'll show how to set up the standalone version of React DevTools to debug your React app in non...
Open your terminal and run the following command: > npm install -g react-devtools 2. After the installation is complete, execute the following command in your terminal: > react-devtools 3. A pop ...
This package can be used to debug non-browser-based React applications (e.g. React Native, mobile browser or embedded webview, Safari). ... The standalone shell can also be useful with React DOM (e.g. to debug apps in Safari or inside of an iframe). Run react-devtools from the terminal to launch the standalone DevTools app:
React Developer Tools is an extension available for Chromium and Firefox browsers that provide accompanying tools to developers for debugging React applications. The key features of the extension include access to a live view of the components attached to each node in the component tree, an inspection of component props and state, an analysis ...
Editor's Note: This article on debugging React applications with React Developer Tools was last revised on 8 February 2023 to reflect recent updates to React Developer Tools and include React Hooks-related features. To learn more about React Hooks check out our cheat sheet here.. Debugging is one of the most useful skills a developer can possess. It allows you to navigate properly, spot ...
A React development environment set up with Create React App. To set this up, follow Step 1 — Creating an Empty Project of the How To Manage State on React Class Components tutorial, which will remove the non-essential boilerplate. This tutorial will use debug-tutorial as the project name.
Breakpoints and the debugger. When debugging a React app, I often find breakpoints to be very helpful. There are two main ways in which we can use them: By writing the debugger statement in our source code. By clicking on a specific line of the code in the Chrome web browser (or Firefox, Edge, etc.) Developer Tools.
Section 6: Conclusion (Emojis: 🎉🚀) Summarize the key takeaways from the guide. Encourage continuous learning and practice for mastering debugging skills in React. Offer resources and further reading for readers interested in diving deeper into React debugging. Remember to include code examples, screenshots, and practical demonstrations to ...
️ This is not only work for React, it also work for any local web application as long as we follow the fundamental steps. Running local React app on Iphone. We will use create-react-app. npx create-react-app demo-app. It might take some time to create an React app, after create one we can access demo-app folder and run up the React app. cd demo-app npm start
Open Safari Technology Preview (since of this writing it is the only version with Safari 16). a. In the Menu Bar enable: Develop -> Allow Unsigned Permissions; Develop -> Experimental Features -> Web Inspector Extensions; b. Open Preferences and navigate to Extensions. React Developer Tools will be shown in the list given the process worked ...
Debugging with React DevTools. React DevTools unlocks powerful JavaScript debugging techniques tailored for React. Setting breakpoints allows pausing execution to inspect component values in the middle of rendering. I can also step through the code line-by-line to isolate issues. The call stack tool provides insightful stack traces.
An extension that allows inspection of React component hierarchy in the Chrome and Firefox Developer Tools. - facebook/react-devtools
Add the following to the scripts section in your project's package.json. "scripts": {. "test:debug": "react-scripts --inspect-brk test --runInBand --no-cache". } Place debugger; statements in any test and run: $ npm run test:debug. This will start running your Jest tests, but pause before executing to allow a debugger to attach to the process.
The only way to currently debug those is using the Safari developer tools. It would be great if there was a port of the react-devtools for Safari. The text was updated successfully, but these errors were encountered:
Visual Studio Code includes a built-in debugger for Edge and Chrome. There are a couple ways to get started with it. Use the Open Link command to debug a URL. Clicking a link in the JavaScript debug terminal. Use a launch config to launch a browser with your app. We also have more detailed walkthroughs to get started with React, Angular, and ...
By default, Create React App will open the default system browser, favoring Chrome on macOS. Specify a browser to override this behavior, or set it to none to disable it completely. If you need to customize the way the browser is launched, you can specify a node script instead.
Start React App with Remote Debugging. In order to debug JS apps, the browser has to be started with remote debugging enabled. For example, Chrome has to be started with the flag --remote-debugging-port=9222. When you click debug on VSCode it does exactly that: It starts a new browser window with this command line argument.
2. We have a reactJs application which works fine on all other browsers except safari on macOS and ios. The app works perfectly on Chrome on macOs or windows and ios as well. Also, once the app freezes, we cannot open dev console in safari and if it is open, most of the things don't work like pausing script execution.
Starting to debug your React app with the VS Code debugger is surprisingly simple. You let VS Code create a launch.json config for you and slightly adjust it. You can find the file in the .vsocde folder in your repository. Depending on your app you need to. adjust the url field (here I change the port to 3000)