- Sign In / Suggest an Article
Current ISO C++ status
Upcoming ISO C++ meetings
Upcoming C++ conferences
Compiler conformance status
ISO C++ committee meeting
March 18-23, Tokyo, Japan
April 17-20, Bristol, UK
using std::cpp 2024
April 24-26, Leganes, Spain
Pure Virtual C++ 2024
April 30, Online
C++ Now 2024
May 7-12, Aspen, CO, USA
June 24-29, St. Louis, MO, USA
July 2-5, Folkestone, Kent, UK

A Tour of C++
by Bjarne Stroustrup
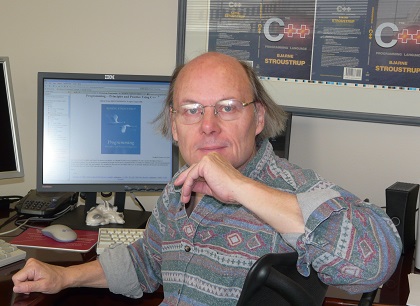
This poses challenges: How do you present C++? What techniques do you recommend? What language features and libraries do you emphasize? Presenting C++11 as a layer on top of C++98 would be as bad as representing C++98 as a layer on top of C. C++ must be presented as a whole, as the powerful tool for design and implementation that it is, rather than a set of independent features.
The Fourth Edition of The C++ Programming Language attempts that and should become available in a few months. To help people get started with C++11 and with TC++PL4, I summarize C++11 in four chapters, collectively named A Tour of C++. Addison-Wesley graciously allowed me to post drafts of these chapters. I will do so over the next months.
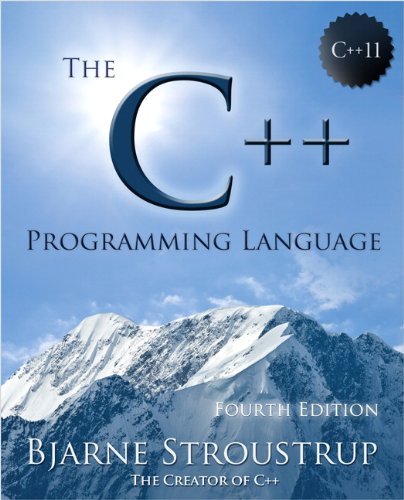
The “Tour” is a tour of ISO Standard C++, rather than a tour of what’s new in C++11 or a tour of your favorite C++ implementation. Also, the tour assumes some familiarity with programming; it is not written for complete novices.
Constructive feedback is most welcome.
- Bjarne Stroustrup (November 2012)
P.S. For a list of new features in C++11, see my C++11 FAQ . Of course, TC++PL4 has such a list, but it is not part of the tour.
Take the Tour
- Part 1: The Basics
- Part 2: Abstraction Mechanisms
- Part 3: Containers and Algorithms
- Part 4: Concurrency and Utilities
The Tour is now also available in book form ; see cover image at right.
Preface to The C++ Programming Language, 4th Ed.
[Added March 2013: The Preface to Stroustrup's TC++PL4e mentioned above is now available and reproduced here with permission.]
All problems in computer science can be solved by another level of indirection, except for the problem of too many layers of indirection.
— David J. Wheeler
C++ feels like a new language. That is, I can express my ideas more clearly, more simply, and more directly in C++11 than I could in C++98. Furthermore, the resulting programs are better checked by the compiler and run faster.
In this book, I aim for completeness. I describe every language feature and standard-library component that a professional programmer is likely to need. For each, I provide:
- Rationale: What kinds of problems is it designed to help solve? What principles underlie the design? What are the fundamental limitations?
- Specification: What is its definition? The level of detail is chosen for the expert programmer; the aspiring language lawyer can follow the many references to the ISO standard.
- Examples: How can it be used well by itself and in combination with other features? What are the key techniques and idioms? What are the implications for maintainability and performance?
The use of C++ has changed dramatically over the years and so has the language itself. From the point of view of a programmer, most of the changes have been improvements. The current ISO standard C++ (ISO/IEC 14882:2011, usually called C++11) is simply a far better tool for writing quality software than were previous versions. How is it a better tool? What kinds of programming styles and techniques does modern C++ support? What language and standard-library features support those techniques? What are the basic building blocks of elegant, correct, maintainable, and efficient C++ code? Those are the key questions answered by this book. Many answers are not the same as you would find with 1985, 1995, or 2005 vintage C++: progress happens.
C++ is a general-purpose programming language emphasizing the design and use of type-rich, lightweight abstractions. It is particularly suited for resource-constrained applications, such as those found in software infrastructures. C++ rewards the programmer who takes the time to master techniques for writing quality code. C++ is a language for someone who takes the task of programming seriously. Our civilization depends critically on software; it had better be quality software.
There are billions of lines of C++ deployed. This puts a premium on stability, so 1985 and 1995 C++ code still works and will continue to work for decades. However, for all applications, you can do better with modern C++; if you stick to older styles, you will be writing lower-quality and worse-performing code. The emphasis on stability also implies that standards-conforming code you write today will still work a couple of decades from now. All code in this book conforms to the 2011 ISO C++ standard.
This book is aimed at three audiences:
- C++ programmers who want to know what the latest ISO C++ standard has to offer,
- C programmers who wonder what C++ provides beyond C, and
- People with a background in application languages, such as Java, C#, Python, and Ruby, looking for something “closer to the machine” -- something more flexible, something offering better compile-time checking, or something offering better performance.
Naturally, these three groups are not disjoint -- a professional software developer masters more than just one programming language.
This book assumes that its readers are programmers. If you ask, “What’s a for-loop?” or “What’s a compiler?” then this book is not (yet) for you; instead, I recommend my Programming: Principles and Practice Using C++ to get started with programming and C++. Furthermore, I assume that readers have some maturity as software developers. If you ask “Why bother testing?” or say, “All languages are basically the same; just show me the syntax” or are confident that there is a single language that is ideal for every task, this is not the book for you.
What features does C++11 offer over and above C++98? A machine model suitable for modern computers with lots of concurrency. Language and standard-library facilities for doing systemslevel concurrent programming (e.g., using multicores). Regular expression handling, resource management pointers, random numbers, improved containers (including, hash tables), and more. General and uniform initialization, a simpler for -statement, move semantics, basic Unicode support, lambdas, general constant expressions, control over class defaults, variadic templates, user-defined literals, and more. Please remember that those libraries and language features exist to support programming techniques for developing quality software. They are meant to be used in combination -- as bricks in a building set -- rather than to be used individually in relative isolation to solve a specific problem. A computer is a universal machine, and C++ serves it in that capacity. In particular, C++’s design aims to be sufficiently flexible and general to cope with future problems undreamed of by its designers.
Acknowledgments
In addition to the people mentioned in the acknowledgment sections of the previous editions, I would like to thank Pete Becker, Hans-J. Boehm, Marshall Clow, Jonathan Coe, Lawrence Crowl, Walter Daugherty, J. Daniel Garcia, Robert Harle, Greg Hickman, Howard Hinnant, Brian Kernighan, Daniel Krügler, Nevin Liber, Michel Michaud, Gary Powell, Jan Christiaan van Winkel, and Leor Zolman. Without their help this book would have been much poorer.
Thanks to Howard Hinnant for answering many questions about the standard library.
Andrew Sutton is the author of the Origin library, which was the testbed for much of the discussion of emulating concepts in the template chapters, and of the matrix library that is the topic of Chapter 29. The Origin library is open source and can be found by searching the Web for “Origin” and “Andrew Sutton.”
Thanks to my graduate design class for finding more problems with the “tour chapters” than anyone else.
Had I been able to follow every piece of advice of my reviewers, the book would undoubtedly have been much improved, but it would also have been hundreds of pages longer. Every expert reviewer suggested adding technical details, advanced examples, and many useful development conventions; every novice reviewer (or educator) suggested adding examples; and most reviewers observed (correctly) that the book may be too long.
Thanks to Princeton University’s Computer Science Department, and especially Prof. Brian Kernighan, for hosting me for part of the sabbatical that gave me time to write this book.
Thanks to Cambridge University’s Computer Lab, and especially Prof. Andy Hopper, for hosting me for part of the sabbatical that gave me time to write this book.
A Tour of C++: Edition 3
About this ebook, ratings and reviews, about the author.
Bjarne Stroustrup is the designer and original implementer of C++ and the author of Programming: Principles and Practice Using C++, Second Edition, and The C++ Programming Language, Fourth Edition, among others. Currently a professor at Columbia University, he has previously worked at AT&T Bell Labs, Texas A&M University, and Morgan Stanley. He is the recipient of numerous honors, including The National Academy of Engineering's Charles Stark Draper Prize for Engineering "for conceptualizing and developing the C++ programming language." Dr. Stroustrup is a member of the National Academy of Engineering, a fellow of IEEE, ACM, CHM, and Churchill College Cambridge.
Rate this ebook
Reading information, more by bjarne stroustrup.
Similar ebooks
Get full access to A Tour of C++, 3rd Edition and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.
A Tour of C++, 3rd Edition
Read it now on the O’Reilly learning platform with a 10-day free trial.
O’Reilly members get unlimited access to books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
Book description
In A Tour of C++, Third Edition, Bjarne Stroustrup provides an overview of ISO C++, C++20, that aims to give experienced programmers a clear understanding of what constitutes modern C++. Featuring carefully crafted examples and practical help in getting started, this revised and updated edition concisely covers most major language features and the major standard-library components needed for effective use.
Stroustrup presents C++ features in the context of the programming styles they support, such as object-oriented and generic programming. His tour is remarkably comprehensive. Coverage begins with the basics, then ranges widely through more advanced topics, emphasizing newer language features. This edition covers many features that are new in C++20 as implemented by major C++ suppliers, including modules, concepts, coroutines, and ranges. It even introduces some library components in current use that are not scheduled for inclusion in the standard until C++23.
This authoritative guide does not aim to teach you how to program (for that, see Stroustrup's Programming: Principles and Practice Using C++, Second Edition ), nor will it be the only resource you'll need for C++ mastery (for that, see Stroustrup's The C++ Programming Language, Fourth Edition, and recommended online sources). If, however, you are a C or C++ programmer wanting greater familiarity with the current C++ language, or a programmer versed in another language wishing to gain an accurate picture of the nature and benefits of modern C++, you won't find a shorter or simpler introduction.
Table of contents
- About This eBook
- Halftitle Page
- Copyright Page
- Acknowledgments
- 1.1 Introduction
- 1.2 Programs
- 1.3 Functions
- 1.4 Types, Variables, and Arithmetic
- 1.5 Scope and Lifetime
- 1.6 Constants
- 1.7 Pointers, Arrays, and References
- 1.9 Mapping to Hardware
- 1.10 Advice
- 2.1 Introduction
- 2.2 Structures
- 2.3 Classes
- 2.4 Enumerations
- 3.1 Introduction
- 3.2 Separate Compilation
- 3.3 Namespaces
- 3.4 Function Arguments and Return Values
- 4.1 Introduction
- 4.2 Exceptions
- 4.3 Invariants
- 4.4 Error-Handling Alternatives
- 4.5 Assertions
- 5.1 Introduction
- 5.2 Concrete Types
- 5.3 Abstract Types
- 5.4 Virtual Functions
- 5.5 Class Hierarchies
- 6.1 Introduction
- 6.2 Copy and Move
- 6.3 Resource Management
- 6.4 Operator Overloading
- 6.5 Conventional Operations
- 6.6 User-Defined Literals
- 7.1 Introduction
- 7.2 Parameterized Types
- 7.3 Parameterized Operations
- 7.4 Template Mechanisms
- 8.1 Introduction
- 8.2 Concepts
- 8.3 Generic Programming
- 8.4 Variadic Templates
- 8.5 Template Compilation Model
- 9.1 Introduction
- 9.2 Standard-Library Components
- 9.3 Standard-Library Organization
- 10.1 Introduction
- 10.2 Strings
- 10.3 String Views
- 10.4 Regular Expressions
- 10.5 Advice
- 11.1 Introduction
- 11.2 Output
- 11.4 I/O State
- 11.5 I/O of User-Defined Types
- 11.6 Output Formatting
- 11.7 Streams
- 11.8 C-style I/O
- 11.9 File System
- 11.10 Advice
- 12.1 Introduction
- 12.2 vector
- 12.4 forward_list
- 12.6 unordered_map
- 12.7 Allocators
- 12.8 Container Overview
- 12.9 Advice
- 13.1 Introduction
- 13.2 Use of Iterators
- 13.3 Iterator Types
- 13.4 Use of Predicates
- 13.5 Algorithm Overview
- 13.6 Parallel Algorithms
- 13.7 Advice
- 14.1 Introduction
- 14.3 Generators
- 14.4 Pipelines
- 14.5 Concepts Overview
- 14.6 Advice
- 15.1 Introduction
- 15.2 Pointers
- 15.3 Containers
- 15.4 Alternatives
- 15.5 Advice
- 16.1 Introduction
- 16.3 Function Adaption
- 16.4 Type Functions
- 16.5 source_location
- 16.6 move() and forward()
- 16.7 Bit Manipulation
- 16.8 Exiting a Program
- 16.9 Advice
- 17.1 Introduction
- 17.2 Mathematical Functions
- 17.3 Numerical Algorithms
- 17.4 Complex Numbers
- 17.5 Random Numbers
- 17.6 Vector Arithmetic
- 17.7 Numeric Limits
- 17.8 Type Aliases
- 17.9 Mathematical Constants
- 17.10 Advice
- 18.1 Introduction
- 18.2 Tasks and threads
- 18.3 Sharing Data
- 18.4 Waiting for Events
- 18.5 Communicating Tasks
- 18.6 Coroutines
- 18.7 Advice
- 19.1 History
- 19.2 C++ Feature Evolution
- 19.3 C/C++ Compatibility
- 19.4 Bibliography
- 19.5 Advice
- A.1 Introduction
- A.2 Use What Your Implementation Offers
- A.3 Use Headers
- A.4 Make Your Own module std
- Code Snippets
Product information
- Title: A Tour of C++, 3rd Edition
- Author(s): Bjarne Stroustrup
- Release date: September 2022
- Publisher(s): Addison-Wesley Professional
- ISBN: 9780136823575
You might also like
A tour of c++, 2nd edition.
by Bjarne Stroustrup
In Bjarne Stroustrup, the creator of C++, describes what constitutes modern C++. This concise, self-contained guide …
Effective C++, Third Edition
by Scott Meyers
“Every C++ professional needs a copy of Effective C++. It is an absolute must-read for anyone …
The C++ Programming Language, 4th Edition
The new C++11 standard allows programmers to express ideas more clearly, simply, and directly, and to …
Discovering Modern C++, 2nd Edition
by Peter Gottschling
Write Powerful, Modern C++ Code for Scientific, Engineering, and Embedded Applications Discovering Modern C++, Second Edition, …
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
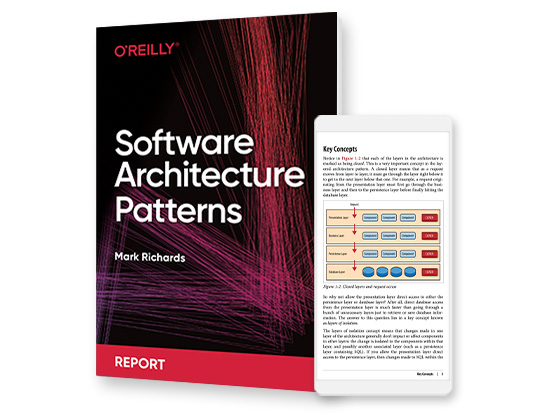
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
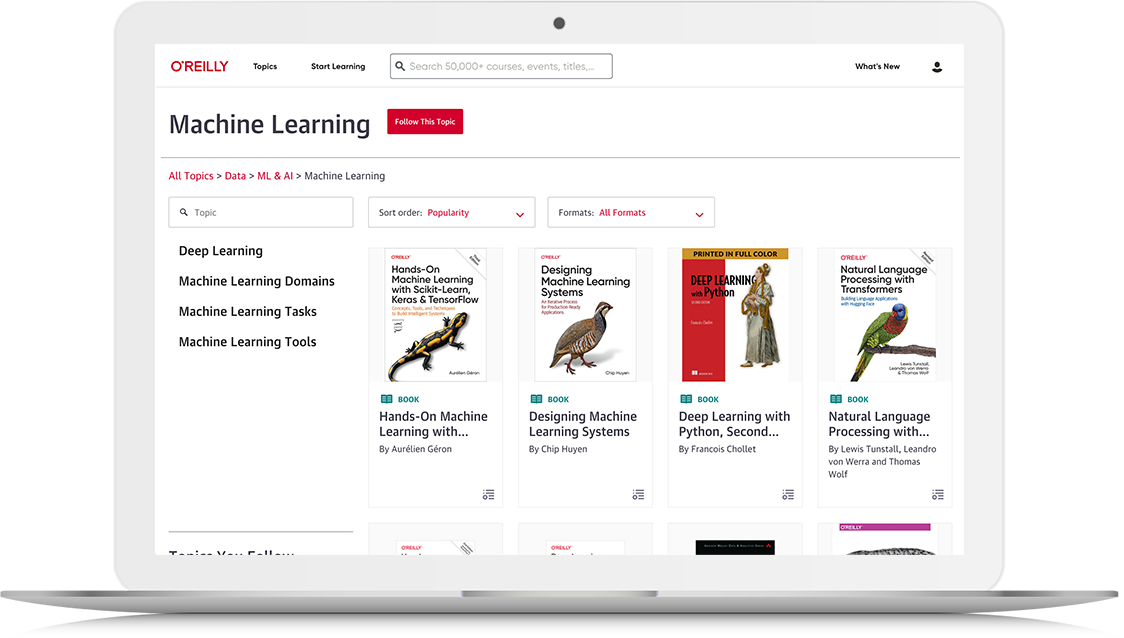
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
A tour of the C# language
- 17 contributors
The C# language is the most popular language for the .NET platform , a free, cross-platform, open source development environment. C# programs can run on many different devices, from Internet of Things (IoT) devices to the cloud and everywhere in between. You can write apps for phone, desktop, and laptop computers and servers.
C# is a cross-platform general purpose language that makes developers productive while writing highly performant code. With millions of developers, C# is the most popular .NET language. C# has broad support in the ecosystem and all .NET workloads . Based on object-oriented principles, it incorporates many features from other paradigms, not least functional programming. Low-level features support high-efficiency scenarios without writing unsafe code. Most of the .NET runtime and libraries are written in C#, and advances in C# often benefit all .NET developers.
Hello world
The "Hello, World" program is traditionally used to introduce a programming language. Here it is in C#:
The line starting with // is a single line comment . C# single line comments start with // and continue to the end of the current line. C# also supports multi-line comments . Multi-line comments start with /* and end with */ . The WriteLine method of the Console class, which is in the System namespace, produces the output of the program. This class is provided by the standard class libraries, which, by default, are automatically referenced in every C# program.
The preceding example shows one form of a "Hello, World" program, using top-level statements . Earlier versions of C# required you to define the program's entry point in a method. This format is still valid, and you'll see it in many existing C# samples. You should be familiar with this form as well, as shown in the following example:
This version shows the building blocks you use in your programs. The "Hello, World" program starts with a using directive that references the System namespace. Namespaces provide a hierarchical means of organizing C# programs and libraries. Namespaces contain types and other namespaces—for example, the System namespace contains many types, such as the Console class referenced in the program, and many other namespaces, such as IO and Collections . A using directive that references a given namespace enables unqualified use of the types that are members of that namespace. Because of the using directive, the program can use Console.WriteLine as shorthand for System.Console.WriteLine . In the earlier example, that namespace was implicitly included.
The Hello class declared by the "Hello, World" program has a single member, the method named Main . The Main method is declared with the static modifier. While instance methods can reference a particular enclosing object instance using the keyword this , static methods operate without reference to a particular object. By convention, when there are no top-level statements a static method named Main serves as the entry point of a C# program.
Both entry point forms produce equivalent code. When you use top-level statements, the compiler synthesizes the containing class and method for the program's entry point.
The examples in this article give you a first look at C# code. Some samples may show elements of C# that you're not familiar with. When you're ready to learn C#, start with our beginner tutorials , or dive into the links in each section. If you're experienced in Java , JavaScript , TypeScript or Python , read our tips to help you find the information you need to quickly learn C#.
Familiar C# features
C# is approachable for beginners yet offers advanced features for experienced developers writing specialized applications. You can be productive quickly. You can learn more specialized techniques as you need them for your applications.
C# apps benefit from the .NET Runtime's automatic memory management . C# apps also use the extensive runtime libraries provided by the .NET SDK. Some components are platform independent, like file system libraries, data collections, and math libraries. Others are specific to a single workload, like the ASP.NET Core web libraries, or the .NET MAUI UI library. A rich Open Source ecosystem on NuGet augments the libraries that are part of the runtime. These libraries provide even more components you can use.
C# is in the C family of languages. C# syntax is familiar if you've used C, C++, JavaScript, or Java. Like all languages in the C family, semi-colons ( ; ) define the end of statements. C# identifiers are case-sensitive. C# has the same use of braces, { and } , control statements like if , else and switch , and looping constructs like for , and while . C# also has a foreach statement for any collection type.
C# is a strongly typed language. Every variable you declare has a type known at compile time. The compiler, or editing tools tell you if you're using that type incorrectly. You can fix those errors before you ever run your program. Fundamental data types are built into the language and runtime: value types like int , double , char , reference types like string , arrays, and other collections. As you write your programs, you create your own types. Those types can be struct types for values, or class types that define object-oriented behavior. You can add the record modifier to either struct or class types so the compiler synthesizes code for equality comparisons. You can also create interface definitions, which define a contract, or a set of members, that a type implementing that interface must provide. You can also define generic types and methods. Generics use type parameters to provide a placeholder for an actual type when used.
As you write code, you define functions, also called methods , as members of struct and class types. These methods define the behavior of your types. Methods can be overloaded, with different number or types of parameters. Methods can optionally return a value. In addition to methods, C# types can have properties , which are data elements backed by functions called accessors . C# types can define events , which allow a type to notify subscribers of important actions. C# supports object oriented techniques such as inheritance and polymorphism for class types.
C# apps use exceptions to report and handle errors. You'll be familiar with this practice if you've used C++ or Java. Your code throws an exception when it can't do what was intended. Other code, no matter how many levels up the call stack, can optionally recover by using a try - catch block.
Distinctive C# features
Some elements of C# might be less familiar. Language integrated query (LINQ) provides a common pattern-based syntax to query or transform any collection of data. LINQ unifies the syntax for querying in-memory collections, structured data like XML or JSON, database storage, and even cloud based data APIs. You learn one set of syntax and you can search and manipulate data regardless of its storage. The following query finds all students whose grade point average is greater than 3.5:
The preceding query works for many storage types represented by Students . It could be a collection of objects, a database table, a cloud storage blob, or an XML structure. The same query syntax works for all storage types.
The Task based asynchronous programming model enables you to write code that reads as though it runs synchronously, even though it runs asynchronously. It utilizes the async and await keywords to describe methods that are asynchronous, and when an expression evaluates asynchronously. The following sample awaits an asynchronous web request. When the asynchronous operation completes, the method returns the length of the response:
C# also supports an await foreach statement to iterate a collection backed by an asynchronous operation, like a GraphQL paging API. The following sample reads data in chunks, returning an iterator that provides access to each element when it's available:
Callers can iterate the collection using an await foreach statement:
C# provides pattern matching . Those expressions enable you to inspect data and make decisions based on its characteristics. Pattern matching provides a great syntax for control flow based on data. The following code shows how methods for the boolean and , or , and xor operations could be expressed using pattern matching syntax:
Pattern matching expressions can be simplified using _ as a catch all for any value. The following example shows how you can simplify the and method:
Finally, as part of the .NET ecosystem, you can use Visual Studio , or Visual Studio Code with the C# DevKit . These tools provide rich understanding of C#, including the code you write. They also provide debugging capabilities.
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
A Tour of C++ (Second edition)
Addison-wesley. isbn 978-0-13-499783-4. july 2018..
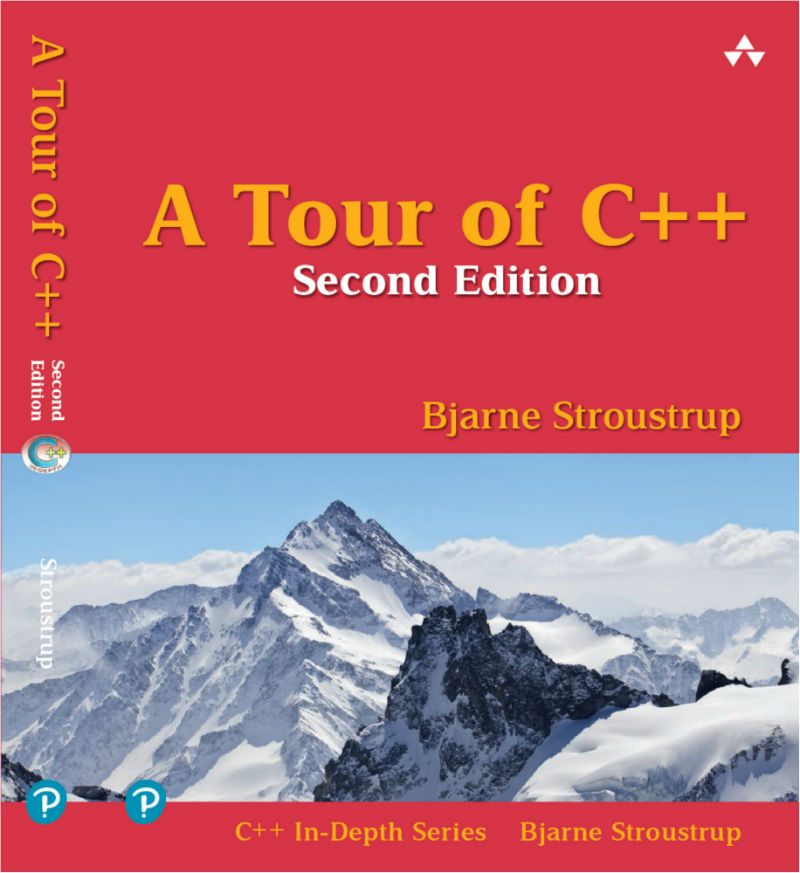
Translations
Prop Farm: Look for Nick Taylor, Adam Hadwin to improve on last year’s Zurich Classic of New Orleans runner-up
Golfbet News
Change Text Size
After 16 straight weeks on TOUR of standard stroke-play tournaments, the Zurich Classic of New Orleans features a twist. Eighty teams of two players each will compete this week at TPC Louisiana with the top 33 teams and ties making the cut after 36 holes. Thursday and Saturday, the two-person teams will play Four-Balls (best ball) and on Friday and Sunday, they will play Foursomes (alternate shot).
It is hard enough to handicap a field of individuals and try to find some winning wagers but when you turn a single player into a two-headed dynamic, the challenges multiply. For any team event, I like to look at current form. If both team members come into the event playing well, that can't be a bad thing. I also like to look at complementary skill sets. Maybe Player A is a great ball-striker and Player B is an excellent putter. And finally, team chemistry. The hard part is much of this is guess work and not truly quantifiable - especially when you pair the two players and we grade their results as one unit.
"This tournament traditionally takes less handle with the team format, which I think either doesn’t resonate with bettors or they just don’t know how to approach handicapping it," said Thomas Gable, director of race and sports at The Borgata in New Jersey.
Senior manager at Mandalay Bay Race and Sportsbook, Tristan Davis, offered his theory. "The team event with alternate formats probably throws a few curveballs into some models, so I'm not expecting a lot from the sharp guys this week," he said.
Personally, I don't believe there is a connection between the betting enthusiasm being down this week and trying to sort through just exactly what Scottie Scheffler has been doing on TOUR for the last couple of months. But I wonder if the interest from the casual fan or recreational bettor would be accelerated to a much greater degree had this been Jordan Spieth or Rory McIlroy on this type of historic run, or Tiger Woods for example.
"(Scheffler's) character is not polarizing, so the story seems nonchalant,” said Las Vegas oddsmaker Jeff Sherman. "Although, they are betting on him to complete the Grand Slam this season and our price has come down."
Davis chimed in, saying, "I think with making big headlines of Scheffler's winning streak, people have to be tuning in. I think there are grounds to say that he would even be attracting new bettors. In the Tiger days, sure, he would make more noise but still I think Scottie and Nelly Korda, for the same reasons, are attracting new bettors. I would be more than happy if Scottie were to play on TOUR every week."
After winning four times in his last five starts, Scheffler is not in this week's field as he continues to eagerly await the birth of his first child - and we eagerly await his return to action. Interestingly enough, Scheffler often pairs with Sam Burns in team formats. Burns, the Louisiana native who attended LSU and has experienced success in this event in the past, is also not in this week's field - as he too is expecting the birth of his first child any day now. But while the activity at the window may be lighter, there are still some bets being made this week in the Big Easy.
"Punters are betting into Theegala/Zalatoris (+800) like they have already won. They have been by far the most popular duo as of now with over 25% of handle," said Davis, Mandalay Bay's veteran bookmaker. "The favorites for the tournament, Cantlay/Schauffele (+450), have also had good support and further down the page the Canadians, Nick Taylor and Adam Hadwin, have also had a good following."
Hadwin and Taylor's interview after Round 4 at Zurich Classic
"Team Theegala/Zalatoris has taken some money for First Round Leader at +1800,” said Gable about what he is seeing at The Borgata. "The McIlroy/Lowry team at +750, however, has attracted the most action for overall winner."
Bogdanovich noted that has seen one small move this week. "Jhonattan Vegas/Bronson Burgoon (+12500) drew a little sharp action at a big number."
Drew O'Dell, senior data analyst at BetMGM, told me their most-bet pairs are among the shortest favorites: Theegala/Zalatoris, Cantlay/Schauffele and McIlroy/Lowry. But one difference surfaces when it comes to biggest liabilities.
"Theegala/Zalatoris is our biggest liability and Cantlay/Schauffele is our third largest,” O’Dell said, “but the team of Nick Taylor and Adam Hadwin show up here as our second-biggest liability."
Theegala/Zalatoris is a great-looking team, perhaps enhanced by their appearances together on TV commercials as a couple of wild-west gunslingers. The Irish duo of McIlroy and Lowry is certainly appealing, and this is McIlroy's first-ever appearance at the Zurich Classic of New Orleans. Schauffele and Cantlay won this tournament in 2022.
Shane Lowry on practicing with Rory McIlroy
The betting volume for myself was down a bit this week compared to traditional weeks on TOUR, but I’m keeping my focus on the outright market. I jumped on the Canadian duo of Taylor and Hadwin (+2500) as they look to build some Presidents Cup momentum together. Other pairs to make my outright card included Morikawa/Kitayama (+1200), Hoge/McNealy (+2500), Smalley/Schmid (+5500) and Echavarria/Greyserman (+8000).
It's a unique week on TOUR, and one that brings with it extra variables – but there are still options to cash a few tickets.
For resources to overcome a gambling problem, call or text 1-800-GAMBLER today.
McIlroy ready to return to PGA Tour policy board, and eager to enjoy his first trip to New Orleans
AVONDALE, La. — For this week, at least, Rory McIlroy is focused on “fun” during his first visit to New Orleans for the PGA Tour’s lone team event.
McIlroy and teammate Shane Lowry have their restaurant reservations booked in this city renowned for its dining scene. A stroll down Bourbon Street also is on McIlroy’s agenda, so he can “say I’ve been there and I’ve got the T-shirt and then move on. I don’t think I want to spend too much time down there.”
Soon, however, the No. 2-ranked golfer in the world will be ready to resume exerting his considerable influence over serious matters surrounding the fractured state of men’s professional golf.
McIlroy said Wednesday that he is interested in returning to the PGA Tour’s policy board, from which he resigned abruptly last November.
“I don’t think there’s been much progress made in the last eight months, and I was hopeful that there would be,” McIlroy said, alluding his goal of seeing a formalized unification of the PGA Tour and upstart, Saudi Arabia-funded LIV Golf.
“I think I could be helpful to the process,” McIlroy said. “But only if people want me involved.”
The PGA Tour and LIV are in merger talks, but they have been protracted, with no clear end in sight. Both tours have continued to operate independently, keeping many of the top names in golf from competing against one another for most of the golf calendar — major tournaments (Masters, US Open, British Open and PGA Championship) excepted.
Meanwhile, the PGA Tour has taken on Strategic Sports Group as a minority investor in a deal that could be worth as much as $3 billion.
Webb Simpson, one of the six player directors on the PGA Tour board and PGA Tour Enterprises board, has submitted a letter saying that he wants to resign as a player director, but only if McIlroy replaces him , according to a person who has seen the letter.
The person spoke to The Associated Press on condition of anonymity Tuesday because the letter was not made public.
After his pro-am round at the Zurich Classic on Wednesday, McIlroy said he started thinking about returning to the board when Simpson approached him about it.
“I said, ‘Look, if it was something that other people wanted, I would gladly take that seat,’ and that was the conversation that we had,” McIlroy said. “I feel like I care a lot, and I have some pretty good experience and good connections within the game and sort of around the wider sort of ecosystem and everything that’s going on.
“But at the end of the day, it’s not quite up to me to just come back on the board,” he added. “There’s a process that has to be followed.”
The other board members are Patrick Cantlay, Peter Malnati, Adam Scott, Jordan Spieth and Tiger Woods.
McIlroy said he sees a completed merger of the PGA and LIV tours as “the only way forward for the game of golf.”
He said he aims to promote compromise while also trying “to help people see the benefits of what unification could do for the game and what it could do for this tour in particular.”
“We obviously realize the game is not unified right now for a reason, and there’s still some hard feelings and things that need to be addressed,” McIlroy said. “But I think at this point, for the good of the game, we all need to put those feelings aside and all move forward together.”
In the meantime, the 34-year-old McIlroy, of Northern Ireland, sounds eager to participate in what, for him, is a new event while taking in one of American’s more culturally distinctive cities.
“This is my 17th year as a professional golfer, and to be able to still do things for the first time like play in this event and experience something like this is pretty cool,” McIlroy said.
McIlroy and Shane Lowry, a 37-yearold Irishman, have been teammates before in the Ryder Cup. So, their partnership at the Zurich Classic is not entirely unfamiliar to them.
“We thought it would be fun to team up together again in something like this,” McIlroy said. “Just really excited to spend the week with Shane.
“To sort of relax and play under maybe not the amount of pressure or the stress that we’ve both been under the last couple of weeks I think is a nice thing,” McIlroy said. “It’s nice to be able to rely on a teammate every now and again and bail you out of trouble or know that you don’t have to play perfect golf because you’ve got someone right there beside you.”
Lowry, meanwhile, sounded no less excited to be able to lean on McIlroy’s game at the Pete Dye-designed TPC Louisiana, where the winning team will earn $2.57 million (about $1.29 million each).
“Rory is probably like No. 1 on people’s lists to come play here with,” Lowry said. “We’ll be good for each other on the course. We’ll enjoy doing it, which is a big part of it as well.”
McIlroy and Lowry are one of several high-profile pairs among the 80 teams at the Zurich.
Others include: 2022 champions Xander Schauffele and Patrick Cantlay; Matt Fitzpatrick and younger brother Alex; Collin Morikawa and Kurt Kitayama; Billy Horschel and Tyson Alexander; Sahith Theegala and Will Zalatoris; Fancesco Molinari and Luke Donald; and defending champions Nick Hardy and Davis Riley.
AP golf: https://apnews.com/hub/golf


- Kindle Store
- Kindle eBooks
- Computers & Technology
Promotions apply when you purchase
These promotions will be applied to this item:
Some promotions may be combined; others are not eligible to be combined with other offers. For details, please see the Terms & Conditions associated with these promotions.
- Highlight, take notes, and search in the book
- In this edition, page numbers are just like the physical edition
Buy for others
Buying and sending ebooks to others.
- Select quantity
- Buy and send eBooks
- Recipients can read on any device
These ebooks can only be redeemed by recipients in the US. Redemption links and eBooks cannot be resold.

Download the free Kindle app and start reading Kindle books instantly on your smartphone, tablet, or computer - no Kindle device required .
Read instantly on your browser with Kindle for Web.
Using your mobile phone camera - scan the code below and download the Kindle app.

Image Unavailable

- To view this video download Flash Player
Follow the author

Tour of C++, A (C++ In-Depth Series) 3rd Edition, Kindle Edition
- ISBN-13 978-0136816485
- Edition 3rd
- Sticky notes On Kindle Scribe
- Publisher Addison-Wesley Professional
- Publication date August 31, 2022
- Language English
- File size 37695 KB
- See all details
- Kindle (5th Generation)
- Kindle Keyboard
- Kindle (2nd Generation)
- Kindle (1st Generation)
- Kindle Paperwhite
- Kindle Paperwhite (5th Generation)
- Kindle Touch
- Kindle Voyage
- Kindle Oasis
- Kindle Scribe (1st Generation)
- Kindle Fire HDX 8.9''
- Kindle Fire HDX
- Kindle Fire HD (3rd Generation)
- Fire HDX 8.9 Tablet
- Fire HD 7 Tablet
- Fire HD 6 Tablet
- Kindle Fire HD 8.9"
- Kindle Fire HD(1st Generation)
- Kindle Fire(2nd Generation)
- Kindle Fire(1st Generation)
- Kindle for Windows 8
- Kindle for Windows Phone
- Kindle for BlackBerry
- Kindle for Android Phones
- Kindle for Android Tablets
- Kindle for iPhone
- Kindle for iPod Touch
- Kindle for iPad
- Kindle for Mac
- Kindle for PC
- Kindle Cloud Reader
Customers who bought this item also bought

From the Publisher


From the Preface
"C++ feels like a a new language. That is, I can express my ideas more clearly, more simply, and more directly today than I could in C++98 or C++11. Furthermore, the resulting programs are better checked by the compiler and run faster.
Like other modern languages, C++ is large and there are a large number of libraries needed for effective use. This thin book aims to give an experienced programmer an idea of what constitutes modern C++. It covers most major language features and the major standard-library components.
...the aim here is not mastery, but to give an overview, to give key examples, and to help a programmer get started [with C++ 20]."
— Bjarne Stroustrup, designer and original implementer of the C++ language
Editorial Reviews
About the author.
Bjarne Stroustrup is the designer and original implementer of C++ and the author of Programming: Principles and Practice Using C++, Second Edition , and The C++ Programming Language, Fourth Edition , among others. Currently a professor at Columbia University, he has previously worked at AT&T Bell Labs, Texas A&M University, and Morgan Stanley. He is the recipient of numerous honors, including The National Academy of Engineering's Charles Stark Draper Prize for Engineering "for conceptualizing and developing the C++ programming language." Dr. Stroustrup is a member of the National Academy of Engineering, a fellow of IEEE, ACM, CHM, and Churchill College Cambridge.
Product details
- ASIN : B0B8S35JWV
- Publisher : Addison-Wesley Professional; 3rd edition (August 31, 2022)
- Publication date : August 31, 2022
- Language : English
- File size : 37695 KB
- Simultaneous device usage : Up to 5 simultaneous devices, per publisher limits
- Text-to-Speech : Enabled
- Screen Reader : Supported
- Enhanced typesetting : Enabled
- X-Ray : Not Enabled
- Word Wise : Not Enabled
- Sticky notes : On Kindle Scribe
- Print length : 320 pages
- #6 in C++ Programming
- #53 in C++ Programming Language
About the author
Bjarne stroustrup.
Bjarne Stroustrup is the designer and original implementer of C++.
He is a founding member of the ISO C++ standards committee and a major contributor to modern C++.
He worked at Bell Labs and is now a professor at Columbia University and a fellow of Churchill College, Cambridge.
He is a member of the USA National Academy of Engineering, an ACM, IEEE, and CHM Fellow.
He is a recipient of the Draper Prize.
His publication list is as long as your arm. For details, see his home pages (www.stroustrup.com)
Customer reviews
Customer Reviews, including Product Star Ratings help customers to learn more about the product and decide whether it is the right product for them.
To calculate the overall star rating and percentage breakdown by star, we don’t use a simple average. Instead, our system considers things like how recent a review is and if the reviewer bought the item on Amazon. It also analyzed reviews to verify trustworthiness.
- Sort reviews by Top reviews Most recent Top reviews
Top reviews from the United States
There was a problem filtering reviews right now. please try again later..

Top reviews from other countries

- Amazon Newsletter
- About Amazon
- Accessibility
- Sustainability
- Press Center
- Investor Relations
- Amazon Devices
- Amazon Science
- Sell on Amazon
- Sell apps on Amazon
- Supply to Amazon
- Protect & Build Your Brand
- Become an Affiliate
- Become a Delivery Driver
- Start a Package Delivery Business
- Advertise Your Products
- Self-Publish with Us
- Become an Amazon Hub Partner
- › See More Ways to Make Money
- Amazon Visa
- Amazon Store Card
- Amazon Secured Card
- Amazon Business Card
- Shop with Points
- Credit Card Marketplace
- Reload Your Balance
- Amazon Currency Converter
- Your Account
- Your Orders
- Shipping Rates & Policies
- Amazon Prime
- Returns & Replacements
- Manage Your Content and Devices
- Recalls and Product Safety Alerts
- Conditions of Use
- Privacy Notice
- Consumer Health Data Privacy Disclosure
- Your Ads Privacy Choices
- Election 2024
- Entertainment
- Newsletters
- Photography
- Personal Finance
- AP Investigations
- AP Buyline Personal Finance
- AP Buyline Shopping
- Press Releases
- Israel-Hamas War
- Russia-Ukraine War
- Global elections
- Asia Pacific
- Latin America
- Middle East
- Election Results
- Delegate Tracker
- AP & Elections
- Auto Racing
- 2024 Paris Olympic Games
- Movie reviews
- Book reviews
- Personal finance
- Financial Markets
- Business Highlights
- Financial wellness
- Artificial Intelligence
- Social Media
PGA Tour players learn how much loyalty is worth in new equity program
Tiger Woods waves after his final round at the Masters golf tournament at Augusta National Golf Club Sunday, April 14, 2024, in Augusta, Ga. (AP Photo/David J. Phillip)
Rory McIlroy, of Northern Ireland, waves after making a putt on the sixth hole during the second round of the RBC Heritage golf tournament, Friday, April 19, 2024, in Hilton Head Island, S.C. (AP Photo/Chris Carlson)
- Copy Link copied

Players who stayed loyal to the PGA Tour amid lucrative recruitment by Saudi-funded LIV Golf are starting to find out how much that loyalty could be worth.
The PGA Tour on Wednesday began contacting the 193 players eligible for the $930 million from a “Player Equity Program” under the new PGA Tour Enterprises .
The bulk of that money — $750 million — went to 36 players based on their career performance, the last five years and how they fared in a recent program that measured their star power.
How much they received was not immediately known. Emails were going out Wednesday afternoon and Thursday informing players of what they would get. One person who saw a list of how the equity shares were doled out said the names had been redacted. The person spoke to The Associated Press on condition of anonymity because many details of the program were not made public.
The Telegraph reported Tiger Woods was to receive $100 million in equity and Rory McIlroy could get $50 million, without saying how it came up with those numbers.
Commissioner Jay Monahan outlined the first-of-its-kind equity ownership program in a Feb. 7 memo to players, a week after Strategic Sports Group became a minority investor in the new commercial PGA Tour Enterprises.
The private equity group, a consortium of professional sports owners led by the Fenway Sports Group, made an initial investment of $1.5 billion that could be worth $3 billion. The tour is still negotiating with the Public Investment Fund of Saudi Arabia — the financial muscle behind the rival LIV Golf league — as an investor.
Any deal with PIF would most certainly increase the value of the equity shares.
Another person with knowledge of the Player Equity Program, speaking on condition of anonymity because of the private nature of the dealings, said the equity money is not part of the SSG investment. That money was geared toward growth capital.
Golf.com received a series of informational videos on the Player Equity Program that was sent to players and reported only 50% of the equity would vest after four years, 25% more after six years and the rest of it after eight years.
It also reported how the 36 players from the top tier were judged on “career points,” such as how long they were full members, victories, how often they reached the Tour Championship and extra points for significant victories.
Jason Gore, the tour’s chief player officer, said in one of the videos, “It’s really about making sure that our players know the PGA Tour is the best place to compete and showing them how much the Tour appreciates them being loyal.”
Emails also were sent to 64 players who would share $75 million in aggregate equity based on the past three years, and $30 million to 57 players who are PGA Tour members. Also, $75 million in equity shares was set aside for 36 past players instrumental in building the tour.
The program has an additional $600 million in equity grants that are recurring for future PGA Tour players. Those would be awarded in amounts of $100 million annually started in 2025.
Players only get equity shares from one of the four tiers now, although everyone would be eligible for the recurring grants.
Even with equity ownership geared toward making the PGA Tour better, the concern was players questioning who got how much and whether they received their fair share.
LIV Golf lured away seven major champions dating to 2018 since it launched in 2022, all with guaranteed contracts and most of them believed to have topped $100 million.
McIlroy, playing this week in the Zurich Classic of New Orleans, was asked how much would make players feel validated for their decision to stay with the PGA Tour.
“I think the one thing we’ve learned in golf over the last two years is there’s never enough,” McIlroy replied.
AP golf: https://apnews.com/hub/golf
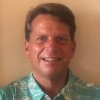
Looking for cheaper Eras Tour tickets? See Taylor Swift at these 10 international cities.

Swifties who want to snag tickets to Taylor Swift’s colossal Eras Tour know the experience won’t come cheap.
This year, the billionaire will only visit three U.S. cities – Miami, New Orleans and Indianapolis – and prices for the in-demand shows are astronomical . For the Oct. 18 show in Miami, for example, cost of a single ticket ranged between $1,615 and $8,524 on StubHub.com as of Wednesday afternoon.
So, how else can fans see one of the world’s biggest singers? Go abroad .
In the past 30 days, searches for ‘Taylor Swift Europe tour 2024 tickets’ have increased by 300% in the U.S., indicating that some people are ready to take a long-haul flight to see the wildly popular artist live, according to travel website Islands.com .
Concert-goers who head to Europe for their Swift experience may actually save more money in the long-run . Not only are ticket prices across the pond cheaper, but many European cities, like Warsaw and Lisbon, are also budget-friendly, offering inexpensive food and lodging.
Learn more: Best travel insurance
Was the travel worth it? Beyoncé and Taylor Swift's summer concerts drew fans from afar
To determine the most affordable Eras Tour stops, Islands.com researchers compared the cost of a two-night trip, including one cheap ticket on StubHub.com, two nights at a four-star hotel, six inexpensive meals, two five-mile taxi rides, and one domestic beer. However, researchers didn’t account for airline tickets or concert merchandise.
With safety at the top of travelers' minds, the researchers also factored in the city’s safety rating out of 100.
10 cheapest destinations for the Eras Tour in 2024
10. Vienna, Austria - total average cost: $1,089, safety score: 69.72
9. Cardiff, U.K. - total average cost: $1,061, safety score: 61.83
8. Lyon, France - total average cost: $1,047, safety score: 44.3
7. Lisbon, Portugal - total average cost: $1,028, safety score: 70.15
6. Munich, Germany - total average cost: $1,000, safety score: 78.88
5. Paris, France - total average cost: $971, safety score: 41.83
4. Stockholm, Sweden - total average cost: $935, safety score: 53.86
3. Hamburg, Germany - total average cost: $903, safety score: 57.51
2. Gelsenkirchen, Germany - total average cost: $749, safety score: 51.33
1. Warsaw, Poland - total average cost: $712, safety score: 72.98
site categories
Megan thee stallion & roc nation sued over unpaid wages & alleged backseat sex action by ‘mean girls’ actress & another woman, idina menzel to launch career-spanning north american tour this summer.
By Greg Evans
NY & Broadway Editor
More Stories By Greg
- Broadway Spring 2024: ‘Uncle Vanya’ & All Of Deadline’s Reviews
- Mary Todd Lincoln Comedy ‘Oh, Mary!’ Sets Broadway Transfer For Summer
- ‘Barbie’ Producer LuckyChap To Make New York Stage Debut With New Work By ‘Titaníque’ Co-Writer & Star Marla Mindelle

Tony winner Idina Menzel will launch a North American tour this summer featuring signature songs from her stage career – including Wicked and Rent – and albums including her latest, Drama Queen .
Menzel’s Take Me Or Leave Me Tour will kick off July 19 in Seattle and run through mid-August, with more than 20 stops including Los Angeles, Dallas, Chicago and New York. See the full itinerary below.
Related Stories

Idina Menzel Commemorates John Travolta's Oscars Blunder By Wishing Adele Dazeem A Happy Birthday

'You Are So Not Invited To My Bat Mitzvah' Review: It's An Adam Sandler Family Affair, But The Kids Steal This Funny And Poignant Coming-Of-Age Teen Comedy
General on-sale for tickets for the tour begins Friday.
Menzel rose to fame for her role as Maureen in the popular Broadway musical Rent , and her career took off when she won a Tony Award for her role as Elphaba, the Wicked Witch of the West, in the smash musical Wicked . Her voice can be heard as Elsa in Disney’s Frozen (the film’s song “Let It Go”, voiced by Menzel, won the Oscar for Best Original Song).
The Take Me or Leave Me Tour – North America 2024 dates are as follows:
July 19: Seattle, WA July 21: Oakland, CA July 23: Los Angeles, CA July 25: Mesa, AZ July 26: Highland, CA July 27: Las Vegas, NV July 30: Austin, TX July 31: Dallas, TX August 2: Atlanta, GA August 3: Charleston, SC August 4: Orlando, FL August 6: Auburn, AL August 7: Greenville, SC August 9: Chicago, IL August 10: Pittsburgh, PA August 11: Detroit, MI August 13: Toronto, ON August 15: New York, NY August 16: Hershey, PA August 17: Washington, DC August 18: Greensboro, NC
Must Read Stories
Comcast edges q1 estimates; ‘dune 2’ leads imax to north american high.

Comer, Taylor-Johnson & Fiennes Set To Lead First Pic In ‘28 Years Later’ Trilogy
Hollywood contraction hits star tv packages: “a head-scratcher”, actors access is latest target in class-action suit over pay-to-play service.
Subscribe to Deadline Breaking News Alerts and keep your inbox happy.
Read More About:
Deadline is a part of Penske Media Corporation. © 2024 Deadline Hollywood, LLC. All Rights Reserved.
Breaking News
Kid Cudi cancels tour: Ankle broken at Coachella is ‘much more serious,’ needs surgery
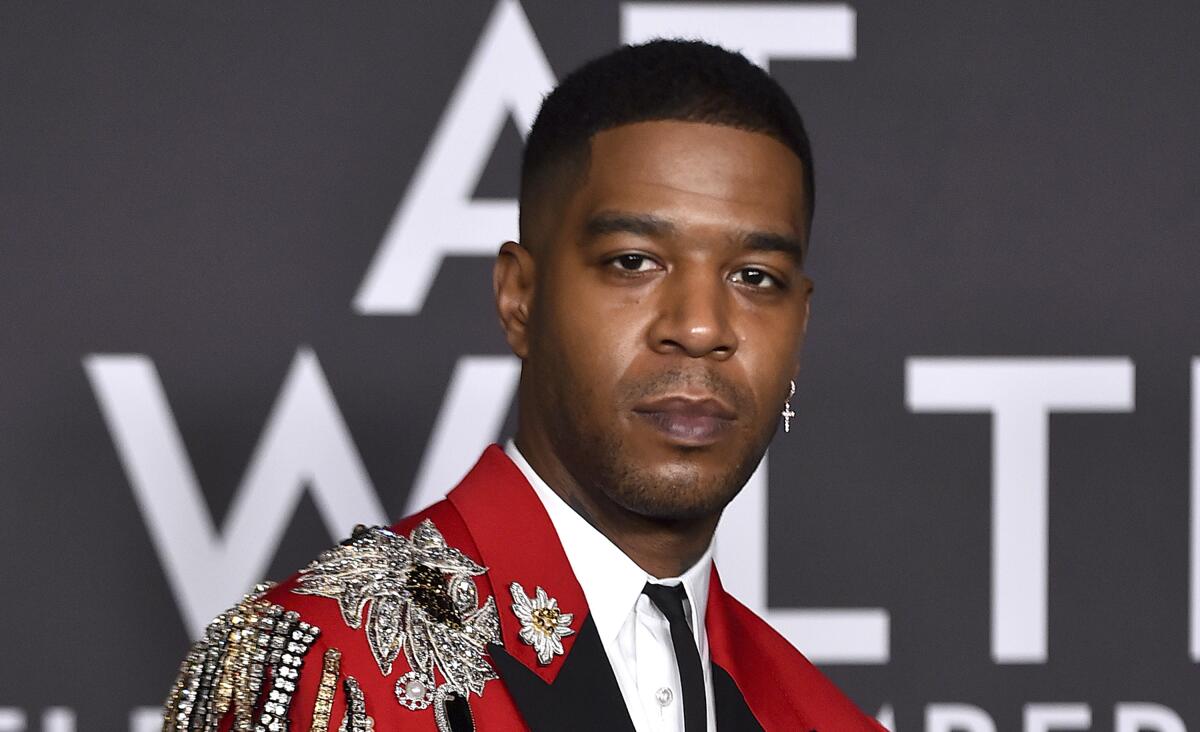
- Show more sharing options
- Copy Link URL Copied!
Kid Cudi is canceling his upcoming Insano: Engage the Rage World Tour after finding out his broken ankle is “much more serious” than he thought.
“Guys, so, I have a broken calcaneus,” Cudi posted Wednesday on social media. “Im headed to surgery now and there’s gonna be a long recovery time. We have to cancel the tour so I can focus on getting better to be out there in top shape to rage with you all.”
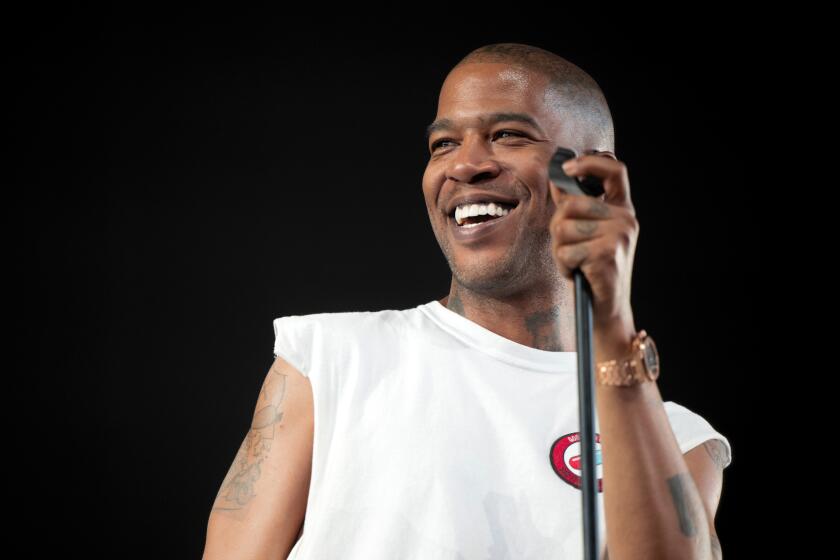
Kid Cudi is ‘hoping’ his Coachella broken ankle will be healed in time for Insano tour
Kid Cudi offers an update on the ankle he broke jumping off the Sahara stage on the last day of Coachella 2024. He seems upbeat, albeit a little uncertain.
April 23, 2024
The calcaneus is more commonly known as the heel bone. While some ankle fractures can heal in weeks, a broken calcaneus has a longer recovery time. “This type of fracture commonly occurs during a high-energy event — such as a car crash or a fall from a ladder — when the heel is crushed under the weight of the body,” the American Academy of Orthopedic Surgeons says on its website . Surgery is often required to prevent heel deformity.
This, friends, is why you don’t jump off one of the tallest stages at Coachella.
“There’s just no way I can bounce back in time to give 100%,” Cudi said. “The injury is much more serious than I thought.”
The U.S. leg of the Insano tour was supposed to start June 28 in Austin, Texas, and conclude with a show in Los Angeles on Aug. 30. Kid Cudi planned to head to Europe in early 2025; it’s unclear whether that part of the tour is still on.
Drake removed from lawsuit over Astroworld crowd crush that left 10 fans dead
A judge removes Drake, who performed with Travis Scott at the 2021 Astroworld concert, from a suit filed by families of the 10 fans who died in a crowd crush.
April 12, 2024
The rapper said that ticket holders will be contacted by email and receive a full refund. Also, new tour dates will be announced as soon as possible.
In a video posted earlier this week and captured by TMZ , Kid Cudi said with a chuckle, “This is what happens when a 40-year-old man tries to prance around offstage like he’s 26, like he used to do back in the day.” He added that he was “hoping” to be healed up in time to hit the road.
So much for that idea.
“I’m so sorry fam and I love you all so much, thanks for the endless love and support. Im really disappointed as im sure you guys are too, but I will be back. Thats a promise,” the rapper wrote Wednesday.
“Im ok, just a lil soreness, but im in good spirits.”
View this post on Instagram A post shared by Willis (@kidcudi)
More to Read
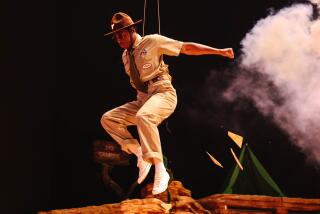
Coachella 2024: Tyler, the Creator wreaks late-night havoc as headliner on Day 2
April 14, 2024
Taylor Swift at Coachella? These are the 3 sets where our experts think she could appear
April 10, 2024
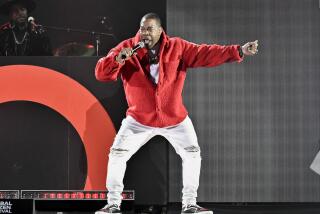
Busta Rhymes cancels 2024 Blockbusta tour less than a week before launch
March 8, 2024
The biggest entertainment stories
Get our big stories about Hollywood, film, television, music, arts, culture and more right in your inbox as soon as they publish.
You may occasionally receive promotional content from the Los Angeles Times.
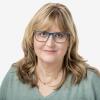
Christie D’Zurilla is an assistant editor for entertainment news on the Fast Break team. A graduate of USC, she joined the Los Angeles Times in 2003 as a copy editor, started writing about celebrities in 2009 and has more than 34 years of journalism experience in Southern California.
More From the Los Angeles Times
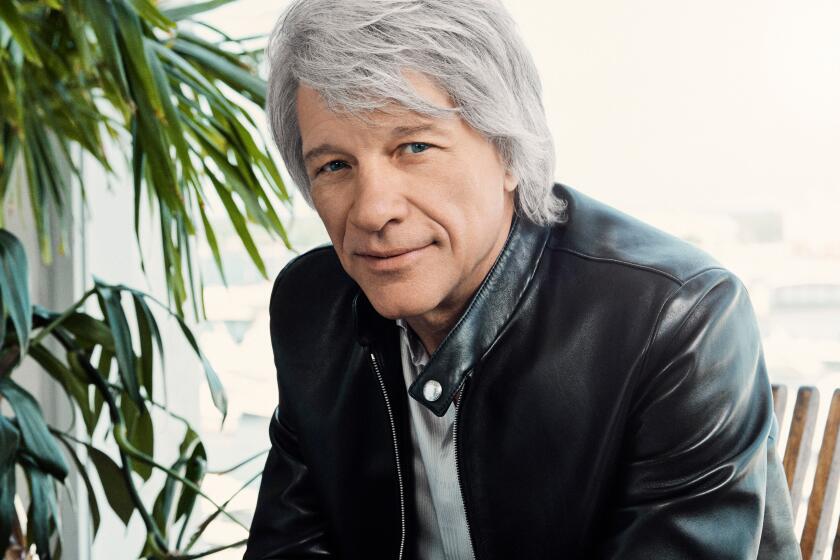
Jon Bon Jovi on Hollywood, Biden and getting ‘punched in the nose’ by a new docuseries
April 25, 2024
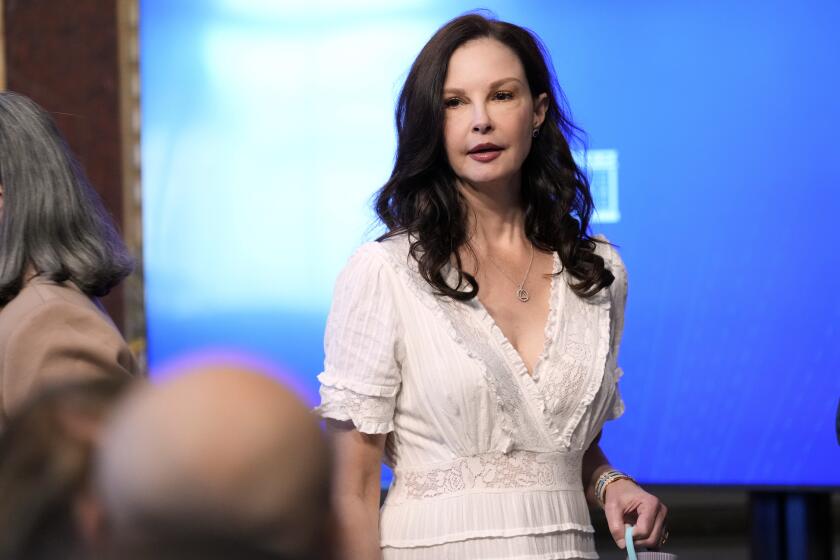
Entertainment & Arts
Ashley Judd, Aloe Blacc open up about deaths of Naomi Judd, Avicii in White House visit
April 24, 2024
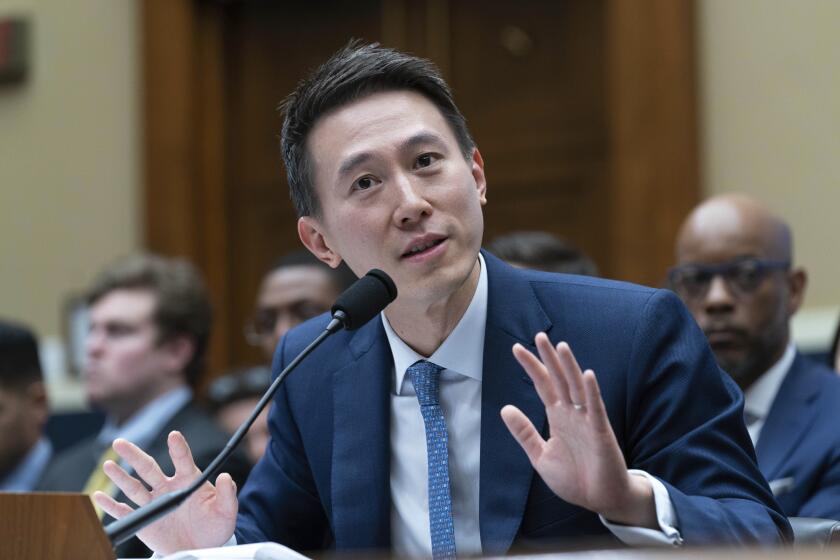
Legislation banning TikTok is coming this week. How will it affect the music industry?
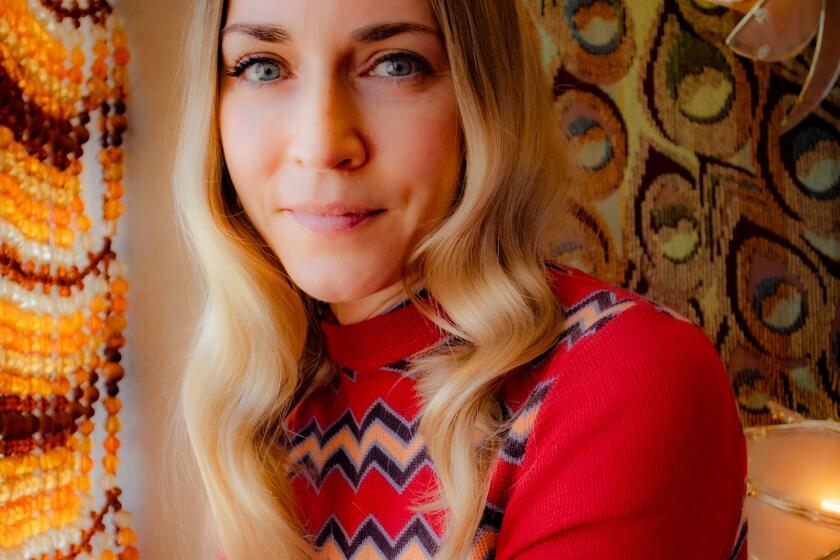
Stagecoach 2024: Goldenvoice’s Stacy Vee on country music’s moment in the sun
Howard Jones, ABC and Haircut 100 Team Up for New Wave Extravaganza North American Tour
By Jem Aswad
Executive Editor, Music
- Peso Pluma Electrifies at Private Club Gig in New York for Sony Electronics 22 hours ago
- Prince’s ‘Musicology’ at 20: A Look at the Album, Tour and Year That Saved His Career 23 hours ago
- MTV’s VMAs to Return to New York in September 24 hours ago

A new wave one-stop shop will tour North America this summer when Howard Jones and ABC will be hitting stages across North America with special guest Haircut 100. The full itinerary appears below.
“I’m very excited to be hitting the road with my friends ABC and Haircut 100,” Jones enthused. “Our audiences can look forward to some seriously top tunes night after night! Bring your celebratory selves to the party.”
Popular on Variety
Of course, the evening will play out like an evening filled with hits from the early days of MTV, when the so-called “Second British Invasion” dominated the channel. Jones’ hits “New Song” and “What Is Love,” and “Things Can Only Get Better” probably segued into or out of ABC’s “Look of Love,” “Poison Arrow” and “Be Near Me” and Haircut 100’s “Love Plus One” and “Fantastic Day.”
All three acts have gone on to other successes, but this tour brings together the songs and sounds that first brought them to fame.
14 Redmond, WA Marymoor Live
17 Sandy City, UT Sandy Amphitheater
18 Las Vegas, NV The Theater At Virgin Hotels
20 Inglewood, CA YouTube Theater
21 Del Mar, CA The Sound
24 Oklahoma City, OK The Zoo Amphitheatre
28 Columbus, OH TempleLive At Columbus Athenaeum
29 Windsor, ON Caesars Windsor – The Colosseum
30 Niagara Falls, ON Fallsview Casino Resort
4 Montclair, NJ The Wellmont
5 Port Chester, NY The Capitol Theatre
6 Huntington, NY The Paramount
More From Our Brands
Nicki nicole reminds an ex what he’s lost on ‘ojos verdes’, cher’s revamped former beverly hills home hits the market for $4.1 million, get ready for the ai-ified olympic games, be tough on dirt but gentle on your body with the best soaps for sensitive skin, ahs: delicate finale delivers ominous, abrupt ending — grade it, verify it's you, please log in.

IMAGES
VIDEO
COMMENTS
In A Tour of C++, Third Edition, Bjarne Stroustrup provides an overview of ISO C++, C++20, that aims to give experienced programmers a clear understanding of what constitutes modern C++. Featuring carefully crafted examples and practical help in getting started, this revised and updated edition concisely covers most major language features and the major standard-library components needed for ...
ISBN-10: -13-681648-7. September 2022. The ``tour'' is a quick (254 pages + index, historical information, etc.) tutorial overview of all of standard C++ (language and standard library) at a moderately high level for people who already know C++ or at least are experienced programmers. It covers C++20 plus a few likely features of C++23.
In A Tour of C++, Second Edition, Bjarne Stroustrup, the creator of C++, describes what constitutes modern C++. This concise, self-contained guide covers most major language features and the major standard-library components―not, of course, in great depth, but to a level that gives programmers a meaningful overview of the language, some key examples, and practical help in getting started.
c ch ha ar r / /character, for example, 'a', 'z', and '9' i in nt t / /integer, for example, 1, 42, and 1216 d do ou ub bl le e / /double-precision floating-point number, for example, 3.14 and 299793.0 A c ch ha ar r variable is of the natural size to hold a character on a given machine (typically a byte), and
In A Tour of C++, Third Edition, Bjarne Stroustrup provides an overview of ISO C++, C++20, that aims to give experienced programmers a clear understanding of what constitutes modern C++. Featuring carefully crafted examples and practical help in getting started, this revised and updated edition concisely covers most major language features and the major standard-library components needed for ...
In A Tour of C++, Second Edition, Bjarne Stroustrup, the creator of C++, describes what constitutes modern C++. This concise, self-contained guide covers most major language features and the major standard-library components—not, of course, in great depth, but to a level that gives programmers a meaningful overview of the language, some key examples, and practical help in getting started.
Stroustrup presents the C++ features in the context of the programming styles they support, such as object-oriented and generic programming. His tour is remarkably comprehensive. Coverage begins with the basics, then ranges widely through more advanced topics, including many that are new in C++11, such as move semantics, uniform initialization ...
Tour A Tour of C++. by Bjarne Stroustrup. C++11 feels like a new language. I write code differently now than I did in C++98. ... Presenting C++11 as a layer on top of C++98 would be as bad as representing C++98 as a layer on top of C. C++ must be presented as a whole, as the powerful tool for design and implementation that it is, rather than a ...
In A Tour of C++, Third Edition, Bjarne Stroustrup provides an overview of ISO C++, C++20, that aims to give experienced programmers a clear understanding of what constitutes modern C++. Featuring carefully crafted examples and practical help in getting started, this revised and updated edition concisely covers most major language features and the major standard-library components needed for ...
In A Tour of C++, Third Edition, Bjarne Stroustrup provides an overview of ISO C++, C++20, that aims to give experienced programmers a clear understanding of what constitutes modern C++. Featuring carefully crafted examples and practical help in getting started, this revised and updated edition concisely covers most major language features and the major standard-library components needed for ...
Tour of C++, A (C++ In-Depth Series) $29.99. (128) In Stock. The C++11 standard allows programmers to express ideas more clearly, simply, and directly, and to write faster, more efficient code. Bjarne Stroustrup, the designer and original implementer of C++, thoroughly covers the details of this language and its use in his definitive reference ...
A Tour of C++. The C++11 standard allows programmers to express ideas more clearly, simply, and directly, and to write faster, more efficient code. Bjarne Stroustrup, the designer and original implementer of C++, thoroughly covers the details of this language and its use in his definitive reference, The C++ Programming Language, Fourth Edition.
Over 7,000 institutions using Bookshelf across 241 countries. Tour of C++, A 3rd Edition is written by Bjarne Stroustrup and published by Addison-Wesley Professional PTG. The Digital and eTextbook ISBNs for Tour of C++, A are 9780136823544, 0136823548 and the print ISBNs are 9780136816485, 0136816487. Save up to 80% versus print by going ...
In A Tour of C++, Third Edition, Bjarne Stroustrup provides an overview of ISO C++, C++20, that aims to give experienced programmers a clear understanding of what constitutes modern C++. Featuring carefully crafted examples and practical help in getting started, this revised and updated edition concisely covers most major language features and the major standard-library components needed for ...
A tour of the C# language. Article 04/09/2024; 17 contributors Feedback. In this article. The C# language is the most popular language for the .NET platform, a free, cross-platform, open source development environment. C# programs can run on many different devices, from Internet of Things (IoT) devices to the cloud and everywhere in between.
ISBN 978--13-499783-4. July 2018. The ``tour'' is a quick (about 240 pages) tutorial overview of all of standard C++ (language and standard library) at a moderately high level for people who already know C++ or at least are experienced programmers. It covers C++17 plus a few likely features of C++20. Available here: The Preface.
This tour presents C++ as an integrated whole, rather than as a layer cake. Consequently, it does not identify language features as present in C, part of C++98, or new in C++11. Such infor-mation can be found in Chapter 14 (History and Compatibility). Acknowledgments
ISBN-13: 978--13-681648-5. In A Tour of C++, Third Edition, Bjarne Stroustrup provides an overview of ISO C++, C++20, that aims to give experienced programmers a clear understanding of what constitutes modern C++. Featuring carefully crafted examples and practical help in getting started, this revised and updated edition concisely covers most ...
Buy now. Sold by InformIT and ebook resellers. ISBN-13: 9780136823544. Tour of C++, A. Published 2022. Need help?
PGA Tour Enterprises is majority owned by the Tour and bolstered by a $1.5 billion investment by Strategic Sports Group, a consortium of sports team owners, the memo said.
After 16 straight weeks on TOUR of standard stroke-play tournaments, the Zurich Classic of New Orleans features a twist. Eighty teams of two players each will c
A Tour of C++. Addison-Wesley. ISBN 978-0321958310. September 2013. Available as eBook and on paper from the publisher. The ``tour'' is a quick (about 180 pages) tutorial overview of all of standard C++ (language and standard library) at a moderately high level for people who already know C++ or at least are experienced programmers.
Rory McIlroy, of Northern Ireland, watches his tee shot on the sixth hole during the second round of the RBC Heritage golf tournament, Friday, April 19, 2024, in Hilton Head Island, S.C. (AP Photo ...
The 43-date Insano world tour was scheduled to kick off on July 28 in Austin, Texas, and featured support from Pusha T, Jaden and Earthgang. From your friend, Scott 🥺🫶🏽 with mad love pic ...
In A Tour of C++, Third Edition, Bjarne Stroustrup provides an overview of ISO C++, C++20, that aims to give experienced programmers a clear understanding of what constitutes modern C++. Featuring carefully crafted examples and practical help in getting started, this revised and updated edition concisely covers most major language features and the major standard-library components needed for ...
The PGA Tour on Wednesday began contacting the 193 players eligible for the $930 million from a "Player Equity Program" under the new PGA Tour Enterprises. The bulk of that money — $750 million — went to 36 players based on their career performance, the last five years and how they fared in a recent program that measured their star power.
Swifties who want to snag tickets to Taylor Swift's colossal Eras Tour know the experience won't come cheap. This year, the billionaire will only visit three U.S. cities - Miami, New Orleans ...
Menzel's Take Me Or Leave Me Tour will kick off July 19 in Seattle and run through mid-August, with more than 20 stops including Los Angeles, Dallas, Chicago and New York. See the full itinerary ...
The U.S. leg of the Insano tour was supposed to start June 28 in Austin, Texas, and conclude with a show in Los Angeles on Aug. 30. Kid Cudi planned to head to Europe in early 2025; it's unclear ...
By Jem Aswad. A new wave one-stop shop will tour North America this summer when Howard Jones and ABC will be hitting stages across North America with special guest Haircut 100. The full itinerary ...