MacOS Safari 11 DOMError "AbortError"
Hi there. When I attempt to play a video on a site I'm working on with 10.13v of MacOs and Safari 11 I'm getting a DOMError - AbortError - The operation was aborted. Does not matter if the video is muted or not. I am not trying to autoplay a video here as this is after our play button is clicked.
- Español – América Latina
- Português – Brasil
- Tiếng Việt
- Chrome for Developers

Abortable fetch
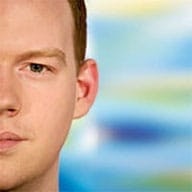
The original GitHub issue for "Aborting a fetch" was opened in 2015. Now, if I take 2015 away from 2017 (the current year), I get 2. This demonstrates a bug in maths, because 2015 was in fact "forever" ago.
2015 was when we first started exploring aborting ongoing fetches, and after 780 GitHub comments, a couple of false starts, and 5 pull requests, we finally have abortable fetch landing in browsers, with the first being Firefox 57.
Update: Noooope, I was wrong. Edge 16 landed with abort support first! Congratulations to the Edge team!
I'll dive into the history later, but first, the API:
The controller + signal manoeuvre
Meet the AbortController and AbortSignal :
The controller only has one method:
When you do this, it notifies the signal:
This API is provided by the DOM standard , and that's the entire API. It's deliberately generic so it can be used by other web standards and JavaScript libraries.
Abort signals and fetch
Fetch can take an AbortSignal . For instance, here's how you'd make a fetch timeout after 5 seconds:
When you abort a fetch, it aborts both the request and response, so any reading of the response body (such as response.text() ) is also aborted.
Here's a demo – At time of writing, the only browser which supports this is Firefox 57. Also, brace yourself, no one with any design skill was involved in creating the demo.
Alternatively, the signal can be given to a request object and later passed to fetch:
This works because request.signal is an AbortSignal .
Reacting to an aborted fetch
When you abort an async operation, the promise rejects with a DOMException named AbortError :
You don't often want to show an error message if the user aborted the operation, as it isn't an "error" if you successfully do what the user asked. To avoid this, use an if-statement such as the one above to handle abort errors specifically.
Here's an example that gives the user a button to load content, and a button to abort. If the fetch errors, an error is shown, unless it's an abort error:
Here's a demo – At time of writing, the only browsers that support this are Edge 16 and Firefox 57.
One signal, many fetches
A single signal can be used to abort many fetches at once:
In the above example, the same signal is used for the initial fetch, and for the parallel chapter fetches. Here's how you'd use fetchStory :
In this case, calling controller.abort() will abort whichever fetches are in-progress.
Other browsers
Edge did a great job to ship this first, and Firefox are hot on their trail. Their engineers implemented from the test suite while the spec was being written. For other browsers, here are the tickets to follow:
In a service worker
I need to finish the spec for the service worker parts , but here's the plan:
As I mentioned before, every Request object has a signal property. Within a service worker, fetchEvent.request.signal will signal abort if the page is no longer interested in the response. As a result, code like this just works:
If the page aborts the fetch, fetchEvent.request.signal signals abort, so the fetch within the service worker also aborts.
If you're fetching something other than event.request , you'll need to pass the signal to your custom fetch(es).
Follow the spec to track this – I'll add links to browser tickets once it's ready for implementation.
The history
Yeah… it took a long time for this relatively simple API to come together. Here's why:
API disagreement
As you can see, the GitHub discussion is pretty long . There a lot of nuance in that thread (and some lack-of-nuance), but the key disagreement is one group wanted the abort method to exist on the object returned by fetch() , whereas the other wanted a separation between getting the response and affecting the response.
These requirements are incompatible, so one group wasn't going to get what they wanted. If that's you, sorry! If it makes you feel better, I was also in that group. But seeing AbortSignal fit the requirements of other APIs makes it seem like the right choice. Also, allowing chained promises to become abortable would become very complicated, if not impossible.
If you wanted to return an object that provides a response, but can also abort, you could create a simple wrapper:
False starts in TC39
There was an effort to make a cancelled action distinct from an error. This included a third promise state to signify "cancelled", and some new syntax to handle cancellation in both sync and async code:
Not real code — proposal was withdrawn
The most common thing to do when an action is cancelled, is nothing. The above proposal separated cancellation from errors so you didn't need to handle abort errors specifically. catch cancel let you hear about cancelled actions, but most of the time you wouldn't need to.
This got to stage 1 in TC39, but consensus wasn't achieved, and the proposal was withdrawn .
Our alternative proposal, AbortController , didn't require any new syntax, so it didn't make sense to spec it within TC39. Everything we needed from JavaScript was already there, so we defined the interfaces within the web platform, specifically the DOM standard . Once we'd made that decision, the rest came together relatively quickly.
Fetch: Abort
As we know, fetch returns a promise. And JavaScript generally has no concept of “aborting” a promise. So how can we cancel an ongoing fetch ? E.g. if the user actions on our site indicate that the fetch isn’t needed any more.
There’s a special built-in object for such purposes: AbortController . It can be used to abort not only fetch , but other asynchronous tasks as well.
The usage is very straightforward:
The AbortController object
Create a controller:
A controller is an extremely simple object.
- It has a single method abort() ,
- And a single property signal that allows to set event listeners on it.
When abort() is called:
- controller.signal emits the "abort" event.
- controller.signal.aborted property becomes true .
Generally, we have two parties in the process:
- The one that performs a cancelable operation, it sets a listener on controller.signal .
- The one that cancels: it calls controller.abort() when needed.
Here’s the full example (without fetch yet):
As we can see, AbortController is just a mean to pass abort events when abort() is called on it.
We could implement the same kind of event listening in our code on our own, without the AbortController object.
But what’s valuable is that fetch knows how to work with the AbortController object. It’s integrated in it.
Using with fetch
To be able to cancel fetch , pass the signal property of an AbortController as a fetch option:
The fetch method knows how to work with AbortController . It will listen to abort events on signal .
Now, to abort, call controller.abort() :
We’re done: fetch gets the event from signal and aborts the request.
When a fetch is aborted, its promise rejects with an error AbortError , so we should handle it, e.g. in try..catch .
Here’s the full example with fetch aborted after 1 second:
AbortController is scalable
AbortController is scalable. It allows to cancel multiple fetches at once.
Here’s a sketch of code that fetches many urls in parallel, and uses a single controller to abort them all:
If we have our own asynchronous tasks, different from fetch , we can use a single AbortController to stop those, together with fetches.
We just need to listen to its abort event in our tasks:
- AbortController is a simple object that generates an abort event on its signal property when the abort() method is called (and also sets signal.aborted to true ).
- fetch integrates with it: we pass the signal property as the option, and then fetch listens to it, so it’s possible to abort the fetch .
- We can use AbortController in our code. The "call abort() " → “listen to abort event” interaction is simple and universal. We can use it even without fetch .
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
More than 3 years have passed since last update.

Safariでvideo.play()したときにAbortErrorと言われたときの対応
※2019年4月22日現在の話です。videoの変更次第ではこの対応では不十分になるかもしれません。 ※音声付きの動画を自動再生したいケースには非対応です。(できるかどうかも未検証です)
iOS12 の Safari で、video.play() した際に、 Unhandled Promise Rejection: AbortError: The operation was aborted. が発生する。 この際、動画が再生されることもあればされないこともある。(多分再生されないのが正しい動き)
参考サイトにあるとおり、 video.muted = true と playsinline を設定する。 また、 autoplay は使わないようにする。
https://lealog.hateblo.jp/entry/2018/03/27/161141 https://github.com/bbc/VideoContext/issues/66
Register as a new user and use Qiita more conveniently
- You get articles that match your needs
- You can efficiently read back useful information
- You can use dark theme
FIX: You receive the error "Operation Aborted" when you use an AJAX-based web application that is built in the .NET Framework 3.5 Service Pack 1
You develop a web application that uses the Asynchronous JavaScript and XML (AJAX) control toolkit in the Microsoft .NET Framework 3.5 Service Pack 1 (SP1). When users connect to the application by using the Windows Internet Explorer over a slow connection, they can receive the following error:
Operation Aborted
Additionally, a blank page is displayed in the Internet Explorer browser. You have to refresh the browser to reload the application. The problem only occurs when there are lots of content or script elements to run after the application calls the Sys.Application.initialize method.
This problem occurs because of an issue that occurs when there is script execution before the webpage's BODY tag is closed.
A supported hotfix is now available from Microsoft. However, it is intended to correct only the problem that is described in this article. Apply it only to systems that are experiencing this specific problem. This hotfix may receive additional testing. Therefore, if you are not severely affected by this problem, we recommend that you wait for the next software update that contains this hotfix. To resolve this problem immediately, contact Microsoft Customer Support Services to obtain the hotfix. For a complete list of Microsoft Customer Support Services telephone numbers and information about support costs, visit the following Microsoft website:
http://support.microsoft.com/contactus/?ws=support Note In special cases, charges that are ordinarily incurred for support calls may be canceled if a Microsoft Support Professional determines that a specific update will resolve your problem. The usual support costs will apply to additional support questions and issues that do not qualify for the specific update in question.
Prerequisites
You must have Microsoft .NET Framework 3.5 Service Pack 1 (SP1) installed to apply this hotfix.
Restart requirements
You do not have to restart the computer after you apply this hotfix.
Hotfix replacement information
This hotfix does not replace a previously released hotfix.
File information
The English (United States) version of this hotfix uses a Microsoft Windows Installer package to install the hotfix. The dates and the times for these files are listed in Coordinated Universal Time (UTC) in the following table. When you view the file information, the date is converted to local time. To find the difference between UTC and local time, use the Time Zone tab in the Date and Time item in Control Panel.
For 32-bit editions of Windows XP, of Windows Server 2003, of Windows Vista, and of Windows Server 2008:
For 64-bit editions of windows xp, of windows server 2003, of windows vista, and of windows server 2008:, for ia-64-bit editions of windows xp, of windows server 2003, of windows vista, and of windows server 2008:.
Microsoft has confirmed that this is a problem in the Microsoft products that are listed in the "Applies to" section.

Need more help?
Want more options.
Explore subscription benefits, browse training courses, learn how to secure your device, and more.
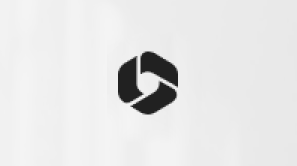
Microsoft 365 subscription benefits
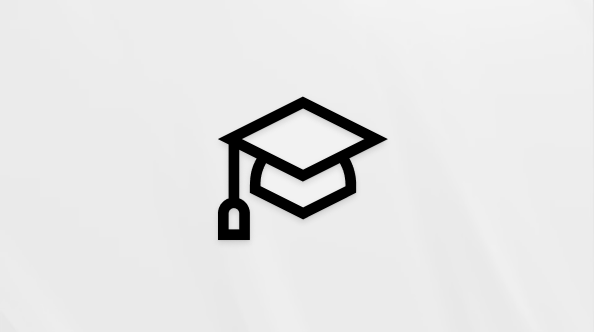
Microsoft 365 training
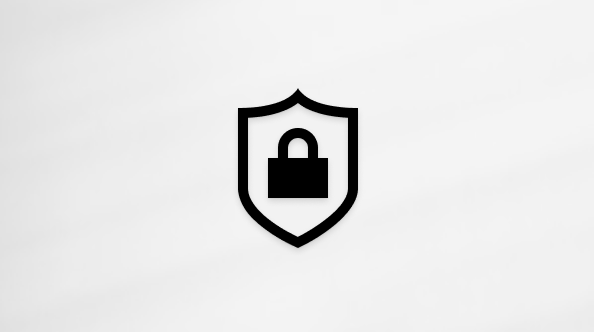
Microsoft security
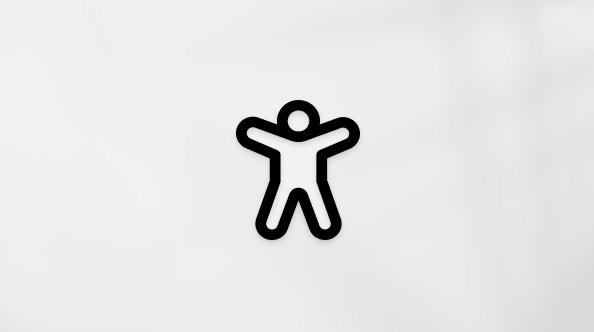
Accessibility center
Communities help you ask and answer questions, give feedback, and hear from experts with rich knowledge.

Ask the Microsoft Community
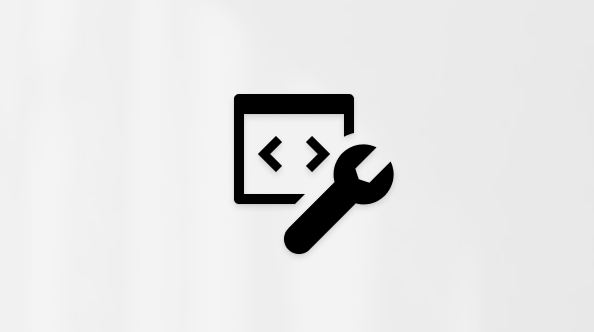
Microsoft Tech Community
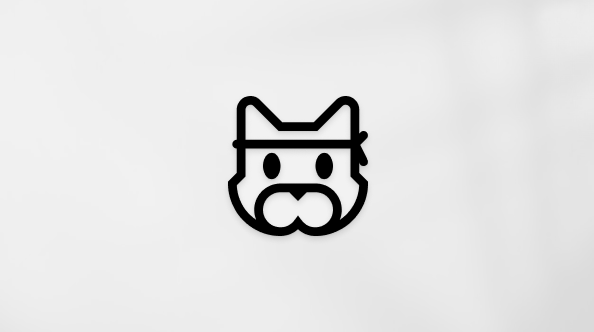
Windows Insiders
Microsoft 365 Insiders
Was this information helpful?
Thank you for your feedback.
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
AbortError: The operation was aborted. #1085
codedmonkey commented Nov 24, 2018 • edited
mohanklein commented Dec 13, 2018
Sorry, something went wrong.
kPanesar commented Jan 27, 2019 • edited
Tehsurfer commented Jan 29, 2019
Kpanesar commented jan 29, 2019.
No branches or pull requests

IMAGES
VIDEO
COMMENTS
Probably related to #14 and prevention of autoplay. Safari 11, both desktop and mobile. DOMError {name: "AbortError", message: "The operation was aborted."} once ...
AbortError: The operation was aborted. Any idea what can be the reason for it? It's occur only on iOS (Chrome & Safari) Thanks Zee. javascript; video; mobile; mobile-safari; http-live-streaming; Share. Improve this question. Follow asked Apr 22, 2019 at 7:18. Zee Zee.
You're now watching this thread. If you've opted in to email or web notifications, you'll be notified when there's activity. Click again to stop watching or visit your profile to manage watched threads and notifications.
signal.addEventListener('abort', => { // Logs true: console.log(signal.aborted); }); This API is provided by the DOM standard , and that's the entire API. It's deliberately generic so it can be used by other web standards and JavaScript libraries.
Summary. AbortController is a simple object that generates an abort event on its signal property when the abort() method is called (and also sets signal.aborted to true).; fetch integrates with it: we pass the signal property as the option, and then fetch listens to it, so it's possible to abort the fetch.; We can use AbortController in our code. The "call abort()" → "listen to abort ...
In JavaScript SDK v3, we added an implementation of WHATWG AbortController interface in @aws-sdk/abort-controller. To use it, you need to send AbortController.signal as abortSignal in the httpOptions parameter when calling .send() operation on the client as follows: abortSignal: abortController.signal, }); // The abortController can be aborted ...
状況. iOS12 の Safari で、video.play () した際に、 Unhandled Promise Rejection: AbortError: The operation was aborted. が発生する。. この際、動画が再生されることもあればされないこともある。. (多分再生されないのが正しい動き).
I can't play my video on iOS and Android Devices. When using Safari Remote Debugging, i get the following issue: Unhandled Promise Rejection: AbortError: The operation was aborted. my html code ...
[Error] Unhandled Promise Rejection: AbortError: The operation was aborted. (anonymous function) rejectPromise. Chrome media internals output. ... Please file a new bug if isSupported returns true and playback with HLS.js is not comparable to Safari's built-in HLS support.
For 64-bit editions of Windows XP, of Windows Server 2003, of Windows Vista, and of Windows Server 2008:
AbortError: The operation was aborted. Is output to console when either: A seek in video element is aborted. The time of a video element is adjusted. Some developers there say that Firefox performs much slower than Chrome or Edge in these scenarios, but I haven't found a way to validate a difference personally.
@ricardodolnl Just extending @damencho comment. In the case of ubuntu, server-id is the default-id if you are running the entire stack on a single VM. But if running JVB on a different VM then server-id is the `private-IP of the instance on which you are running the JVB.. While in Docker even if you are running it on the same/different VM. server-id needs to be the jvb-container-IP in case of ...
After my webpage with Howler working perfectly for a few months, it seems everything has been broken now and I'm getting a lot of AbortError: The operation was aborted. errors, for example when I'm seeking through the audio which only sometimes results in the player not being able to move it's position and it either keeps playing the previous ...