- Practice Backtracking
- Interview Problems on Backtracking
- MCQs on Backtracking
- Tutorial on Backtracking
- Backtracking vs Recursion
- Backtracking vs Branch & Bound
- Print Permutations
- Subset Sum Problem
- N-Queen Problem
- Knight's Tour
- Sudoku Solver
- Rat in Maze
- Hamiltonian Cycle
- Graph Coloring
- Backtracking Algorithm
- Introduction to Backtracking - Data Structure and Algorithm Tutorials
- Difference between Backtracking and Branch-N-Bound technique
- What is the difference between Backtracking and Recursion?

Standard problems on backtracking
- The Knight's tour problem
- Rat in a Maze
- N Queen Problem
- Subset Sum Problem using Backtracking
- M-Coloring Problem
- Algorithm to Solve Sudoku | Sudoku Solver
- Magnet Puzzle
- Remove Invalid Parentheses
- A backtracking approach to generate n bit Gray Codes
- Permutations of given String
Easy Problems on Backtracking
- Print all subsets of a given Set or Array
- Check if a given string is sum-string
- Count all possible Paths between two Vertices
- Find all distinct subsets of a given set using BitMasking Approach
- Find if there is a path of more than k length from a source
- Print all paths from a given source to a destination
- Print all possible strings that can be made by placing spaces
Medium prblems on Backtracking
- 8 queen problem
- Combinational Sum
- Warnsdorff's algorithm for Knight’s tour problem
- Find paths from corner cell to middle cell in maze
- Find Maximum number possible by doing at-most K swaps
- Rat in a Maze with multiple steps or jump allowed
- N Queen in O(n) space
Hard problems on Backtracking
- Power Set in Lexicographic order
- Word Break Problem using Backtracking
- Partition of a set into K subsets with equal sum
- Longest Possible Route in a Matrix with Hurdles
- Find shortest safe route in a path with landmines
- Printing all solutions in N-Queen Problem
- Print all longest common sub-sequences in lexicographical order
- Top 20 Backtracking Algorithm Interview Questions
The Knight’s tour problem
Backtracking is an algorithmic paradigm that tries different solutions until finds a solution that “works”. Problems that are typically solved using the backtracking technique have the following property in common. These problems can only be solved by trying every possible configuration and each configuration is tried only once. A Naive solution for these problems is to try all configurations and output a configuration that follows given problem constraints. Backtracking works incrementally and is an optimization over the Naive solution where all possible configurations are generated and tried. For example, consider the following Knight’s Tour problem.
Problem Statement: Given a N*N board with the Knight placed on the first block of an empty board. Moving according to the rules of chess knight must visit each square exactly once. Print the order of each cell in which they are visited.
The path followed by Knight to cover all the cells Following is a chessboard with 8 x 8 cells. Numbers in cells indicate the move number of Knight.
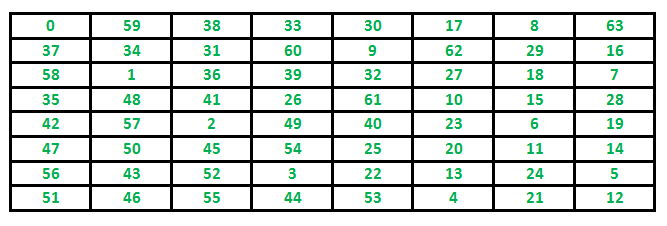
Let us first discuss the Naive algorithm for this problem and then the Backtracking algorithm.
Naive Algorithm for Knight’s tour The Naive Algorithm is to generate all tours one by one and check if the generated tour satisfies the constraints.
Backtracking works in an incremental way to attack problems. Typically, we start from an empty solution vector and one by one add items (Meaning of item varies from problem to problem. In the context of Knight’s tour problem, an item is a Knight’s move). When we add an item, we check if adding the current item violates the problem constraint, if it does then we remove the item and try other alternatives. If none of the alternatives works out then we go to the previous stage and remove the item added in the previous stage. If we reach the initial stage back then we say that no solution exists. If adding an item doesn’t violate constraints then we recursively add items one by one. If the solution vector becomes complete then we print the solution.
Backtracking Algorithm for Knight’s tour
Following is the Backtracking algorithm for Knight’s tour problem.
Following are implementations for Knight’s tour problem. It prints one of the possible solutions in 2D matrix form. Basically, the output is a 2D 8*8 matrix with numbers from 0 to 63 and these numbers show steps made by Knight.
Time Complexity : There are N 2 Cells and for each, we have a maximum of 8 possible moves to choose from, so the worst running time is O(8 N^2 ).
Auxiliary Space: O(N 2 )
Important Note: No order of the xMove, yMove is wrong, but they will affect the running time of the algorithm drastically. For example, think of the case where the 8th choice of the move is the correct one, and before that our code ran 7 different wrong paths. It’s always a good idea a have a heuristic than to try backtracking randomly. Like, in this case, we know the next step would probably be in the south or east direction, then checking the paths which lead their first is a better strategy.
Note that Backtracking is not the best solution for the Knight’s tour problem. See the below article for other better solutions. The purpose of this post is to explain Backtracking with an example. Warnsdorff’s algorithm for Knight’s tour problem
References: http://see.stanford.edu/materials/icspacs106b/H19-RecBacktrackExamples.pdf http://www.cis.upenn.edu/~matuszek/cit594-2009/Lectures/35-backtracking.ppt http://mathworld.wolfram.com/KnightsTour.html http://en.wikipedia.org/wiki/Knight%27s_tour
Please Login to comment...
Similar reads.
- chessboard-problems
- Backtracking
- Mathematical
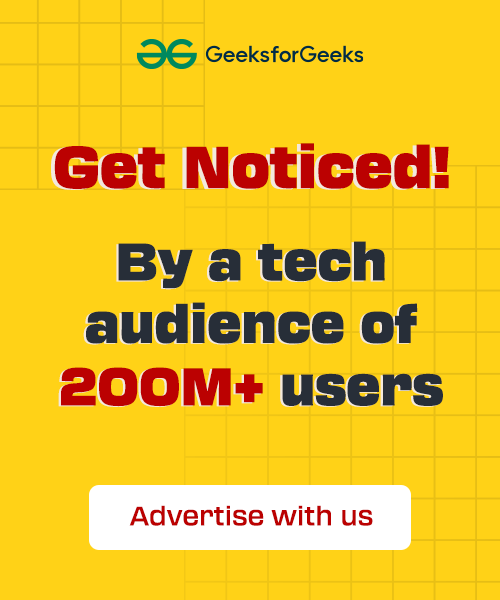
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
Approach: Using Backtracking
In this approach, we start with an empty chessboard and try to place the Knight on each square in turn, backtracking when we reach a point where no further moves are possible. The Backtracking approach is a common algorithmic technique used to solve optimization problems, where we are searching for the best solution among many possible solutions.
- Define a static integer variable N representing the chessboard size (8).
- Define a Method isSafe(x, y, sol) to check if a knight can be placed at position (x, y) on the chessboard. The Method checks if (x, y) is within the board limits and if a knight does not already occupy the position.
- Define a Method print solution (sol) to print the final knight tour solution.
- Define a Method to solve KT () to solve the Knight's tour problem. a. Initialize an empty 2D array (sol) of size NxN, where -1 represents an empty cell. b. Define two arrays (xMove, yMove) to represent all possible knight moves. c. Set the starting position to (0,0) and mark it as visited by setting sol[0][0] to 0. d. Call the recursive Method solveKTUtil() with the starting position, movei=1, and the arrays xMove and yMove. e. If solveKTUtil() returns false, the Knight's tour solution does not exist. If it returns true, print the solution using printSolution() and return true.
- Define a recursive Method solveKTUtil(x, y, movie, sol, xMove, yMove) to solve the Knight's tour problem. a. If movie = N*N, the Knight's tour solution has been found, return true. b. Loop through all possible knight moves (k) using xMove and yMove arrays. c. Calculate the next position (next_x, next_y) using the current position (x, y) and the current knight move (k). d. If the next position is safe (i.e., isSafe(next_x, next_y, sol) returns true), mark it as visited by setting sol[next_x][next_y] = movei, and recursively call solveKTUtil() with the next position (next_x, next_y) and movei+1. e. If solveKTUtil() returns true, the Knight's tour solution has been found, return true. If it returns false, mark the next position as unvisited by setting sol[next_x][next_y] = -1 and continue to the next possible knight move. f. If no move leads to a solution, return false.
- The main method, called solveKT(), is to solve the Knight's tour problem. If a solution exists, it will be printed to the console.
Implementation
Filename: KnightTour.java
Complexity Analysis: The time complexity of the Knight's tour problem using backtracking is O(8^(N^2)), where N is the size of the chessboard. It is because at each move, the Knight has 8 possible moves to choose from, and the total number of moves the Knight can make is N^2.
The space complexity of the program is O(N^2).

- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- PyQt5 ebook
- Tkinter ebook
- SQLite Python
- wxPython ebook
- Windows API ebook
- Java Swing ebook
- Java games ebook
- MySQL Java ebook
Java 2D games
last modified January 10, 2023
This is Java 2D games tutorial. In this tutorial, you will learn the basics of 2D game programming in Java. The Java 2D games tutorial is suitable for beginners and intermediate programmers.
Table of contents
- Moving sprites
- Collision detection
- Space Invaders
- Minesweeper
The tutorial uses Java Swing to create the games.
A unique e-book Java 2D games programming is available on ZetCode. The e-book is in PDF format and has 115 pages.
Related tutorials
You might also want to look at the Java tutorial , Displaying image in Java tutorial , the Java Swing tutorial , the JavaFX tutorial , or the Java 2D tutorial .
Explore your training options in 10 minutes Get Started
- Graduate Stories
- Partner Spotlights
- Bootcamp Prep
- Bootcamp Admissions
- University Bootcamps
- Coding Tools
- Software Engineering
- Web Development
- Data Science
- Tech Guides
- Tech Resources
- Career Advice
- Online Learning
- Internships
- Apprenticeships
- Tech Salaries
- Associate Degree
- Bachelor's Degree
- Master's Degree
- University Admissions
- Best Schools
- Certifications
- Bootcamp Financing
- Higher Ed Financing
- Scholarships
- Financial Aid
- Best Coding Bootcamps
- Best Online Bootcamps
- Best Web Design Bootcamps
- Best Data Science Bootcamps
- Best Technology Sales Bootcamps
- Best Data Analytics Bootcamps
- Best Cybersecurity Bootcamps
- Best Digital Marketing Bootcamps
- Los Angeles
- San Francisco
- Browse All Locations
- Digital Marketing
- Machine Learning
- See All Subjects
- Bootcamps 101
- Full-Stack Development
- Career Changes
- View all Career Discussions
- Mobile App Development
- Cybersecurity
- Product Management
- UX/UI Design
- What is a Coding Bootcamp?
- Are Coding Bootcamps Worth It?
- How to Choose a Coding Bootcamp
- Best Online Coding Bootcamps and Courses
- Best Free Bootcamps and Coding Training
- Coding Bootcamp vs. Community College
- Coding Bootcamp vs. Self-Learning
- Bootcamps vs. Certifications: Compared
- What Is a Coding Bootcamp Job Guarantee?
- How to Pay for Coding Bootcamp
- Ultimate Guide to Coding Bootcamp Loans
- Best Coding Bootcamp Scholarships and Grants
- Education Stipends for Coding Bootcamps
- Get Your Coding Bootcamp Sponsored by Your Employer
- GI Bill and Coding Bootcamps
- Tech Intevriews
- Our Enterprise Solution
- Connect With Us
- Publication
- Reskill America
- Partner With Us
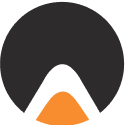
- Resource Center
- Bachelor’s Degree
- Master’s Degree
The Top Coding Games to Help You Learn Java
We all know how vital Java programming and software development skills are to modern businesses. Folks with Java coding chops can write their own ticket—they make great money and get to tackle exciting challenges. The question, then, is how to get the coding skills in the first place? There must be a way to learn Java that offers a little more engagement and excitement than sitting in a classroom or studying a vast, heavy textbook, right?
Your dreams have come true, my friend. There are lots of games you can play that will help you polish your Java skills and keep you entertained at the same time. We’ve gathered a few of the best games in this guide so you can have fun and get your Java coding skills up to speed. These games will give you the essential programming habits and ways of thinking that will be invaluable when you write code.
Find your bootcamp match
Play some robocode.
Who doesn’t love a good tank game? There’s something primal about driving a massive hunk of metal around, dealing death to all your foes through your mighty cannon of doom that is in no way a comment on masculinity whatsoever. And if you can learn Java while you wipe out your tank-clad enemies? Even better! You’ll get all of this and more when you play Robocode , a time-tested way to get your Java skills in shape.
When you play Robocode, you control your tank by developing the AI. To accomplish this, you’ll need to write new code for your craft in Java and other languages. The game is open source and is practically a world heritage site in Internet terms, considering it launched in 2000. Its longevity speaks to its usefulness and effectiveness at teaching Java to generations of gamers. You’ll love it.
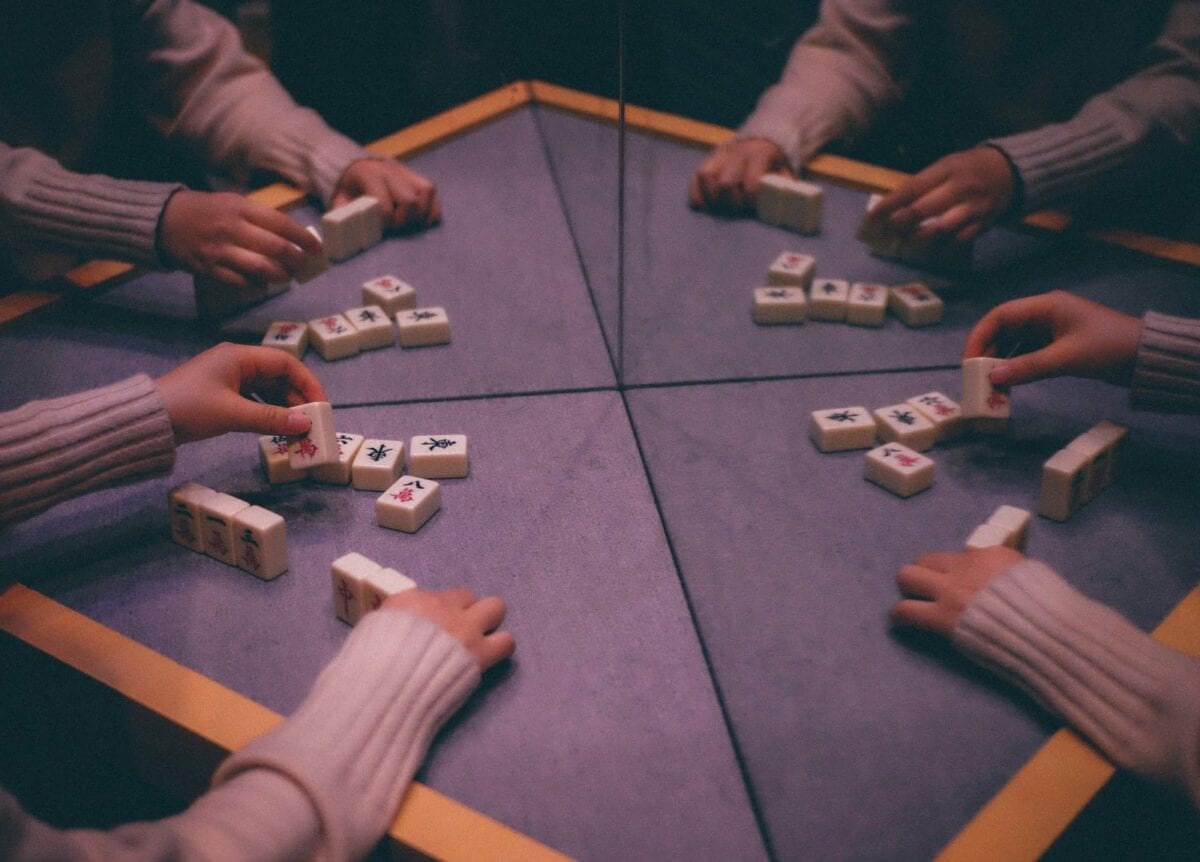
Try Playing Codingame
Maybe tanks aren’t your speed. If you prefer a more brain-twistery sort of game that requires problem- and puzzle-solving skills, you should check out Codingame . Codingame offers multiple challenges and brain teasers that you have to solve to proceed. You’ll need to write code to get through the puzzles, and you can use one of over 25 supported languages, including Java.
The game presents a web browser with a full software development workspace to help you write code and move through the game. The game isn’t just for single players, either—you can set up multiplayer gaming sessions to train yourself and your friends on collaboration and teamwork. Even better, your solutions will be reviewed by other community members, who make recommendations and offer tips to help you get better fast.
And there you go, my buddies and pals. Having programming skills is a must for ambitious employees, but learning those skills can be tedious and frustrating. This guide takes you on a tour of games that will help you learn Java and other coding languages and will help you get your new career off the ground.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication .
What's Next?

Get matched with top bootcamps
Ask a question to our community, take our careers quiz.
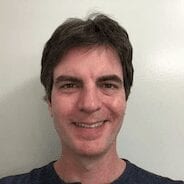
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
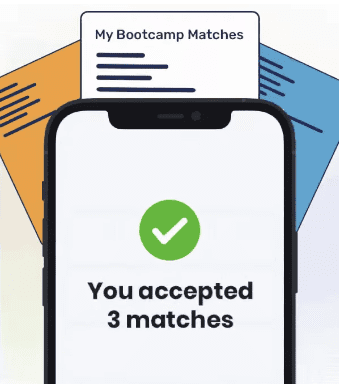
Learn Coding USA
Your first java game: step-by-step coding tutorial.
Last Updated on November 26, 2023
Introduction
Java has become popular for game development due to its versatility and cross-platform compatibility.
In this coding tutorial, we will walk through each step of developing a Java game.
From setting up the development environment to implementing game mechanics, this tutorial will provide a comprehensive guide for beginners.
By the end, readers will have a solid foundation in Java game development.
So let’s dive in and start coding our first Java game!
Setting Up the Development Environment
In order to develop a Java game, you will need a few necessary tools. These include:
- Java Development Kit (JDK) : This is the software platform used to compile and run Java programs.
- Integrated Development Environment (IDE) : An IDE provides a user-friendly interface for writing, testing, and debugging code.
Instructions to install Java Development Kit (JDK) and an Integrated Development Environment (IDE)
To install the JDK and an IDE, follow these step-by-step instructions
- Download the JDK installer from the Oracle website.
- Open the installer and follow the on-screen instructions to install the JDK.
- Once the installation is complete, open the IDE installer.
- Follow the on-screen instructions to install the IDE.
- After the installation is done, open the IDE and create a new Java project.
- Set the JDK path in the IDE to the installed JDK directory.
- Create a new Java class to write your game code.
You are now ready to start coding your first Java game!
Read: Securing Your Java Application: Best Practices
Understanding the Basics
In this section, we will explore the concepts of object-oriented programming and game development, as well as introduce the main components required for developing a Java game.
1. Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm based on the concept of “objects”.
It allows programmers to create modular and reusable code by combining data and behavior into objects.
Java is an object-oriented programming language, making it ideal for game development.
2. Game Development
Game development involves creating interactive experiences, typically for entertainment or educational purposes.
Developing a game requires a combination of programming, design, and creativity.
Game development can be a rewarding process, allowing developers to bring their ideas to life.
3. Required Components for Developing a Java Game
Before diving into the coding aspect, let’s discuss the main components necessary for developing a Java game.
- Graphics: Graphics are an essential component of any game, as they provide visual representation to the players. In Java game development, you can leverage libraries like AWT (Abstract Window Toolkit) or JavaFX for graphics rendering. These libraries provide classes and methods for creating and manipulating graphical elements.
- Input Controls: Input controls allow players to interact with the game through various input devices, such as keyboards or controllers. In Java, you can use the java.awt.event package to handle user input, such as keyboard and mouse events. By detecting and processing input events, you can create responsive and interactive gameplay experiences.
- Game Logic : The game logic determines how the game behaves and responds to player actions. It encompasses rules, calculations, and algorithms that govern the gameplay. In Java game development, you will write code to implement the game logic using object-oriented programming principles.
- Audio: Audio adds another layer of immersion to the game by providing sound effects and music. Java provides libraries like javax.sound.sampled for handling audio playback and manipulation. You can use these libraries to load, play, and control different types of audio files in your Java game.
- Game State Management : Game state management involves keeping track of the current state of the game, such as levels, scores, or player progress. Using object-oriented programming, you can create classes and objects to represent different game states. The game’s logic can then manipulate these objects to maintain and update the game state as the gameplay progresses. By understanding these fundamental components, you are now equipped with the knowledge necessary to start developing your first Java game. In the following section, we will begin the step-by-step coding tutorial.
Read: Java and Big Data: How They Work Together
Creating the Game Window
In this section, we will provide step-by-step instructions on how to create a new Java project and set up the game window using JFrame and JPanel.
We will also demonstrate the implementation of a basic window with the desired dimensions.
Step-by-step Instructions to Create a New Java Project:
- Open your Java Integrated Development Environment (IDE).
- Create a new Java project by selecting “File” -> “New” -> “Java Project”.
- Name your project and select the desired location to save it.
- Click “Finish” to create the project.
Guide for Setting up the Game Window using JFrame and JPanel:
In your newly created Java project, create a new Java class.
- Name the class according to your preference. For example, “GameWindow”.
- Import the necessary libraries: import javax.swing.JFrame; and import javax.swing.JPanel; .
- Create a class that extends JFrame to represent the game window: public class GameWindow extends JFrame { ... } .
- Inside the class, create a constructor and set the desired dimensions of the game window: public GameWindow(int width, int height) { ... } .
- Within the constructor, use setSize() to set the width and height of the window : setSize(width, height); .
- Set the default close operation using setDefaultCloseOperation() and specify EXIT_ON_CLOSE : setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); .
- Create a new instance of a JPanel to be used as the content pane: JPanel panel = new JPanel(); .
- Set the content pane of the JFrame using setContentPane() : setContentPane(panel); .
- Call setVisible(true) to make the window visible: setVisible(true); .
Implementation of a Basic Window with the Desired Dimensions:
Now that we have set up the game window using JFrame and JPanel, let’s implement a basic window with the desired dimensions:
When we run the above code, a new game window with the specified dimensions of 800 pixels in width and 600 pixels in height will be displayed.
In this section, we have learned how to create a new Java project, set up the game window using JFrame and JPanel, and implement a basic window with the desired dimensions.
Next, in Section 4, we will delve into creating the game graphics and handling user input.
Read: Migrating to Java 11: What You Need to Know
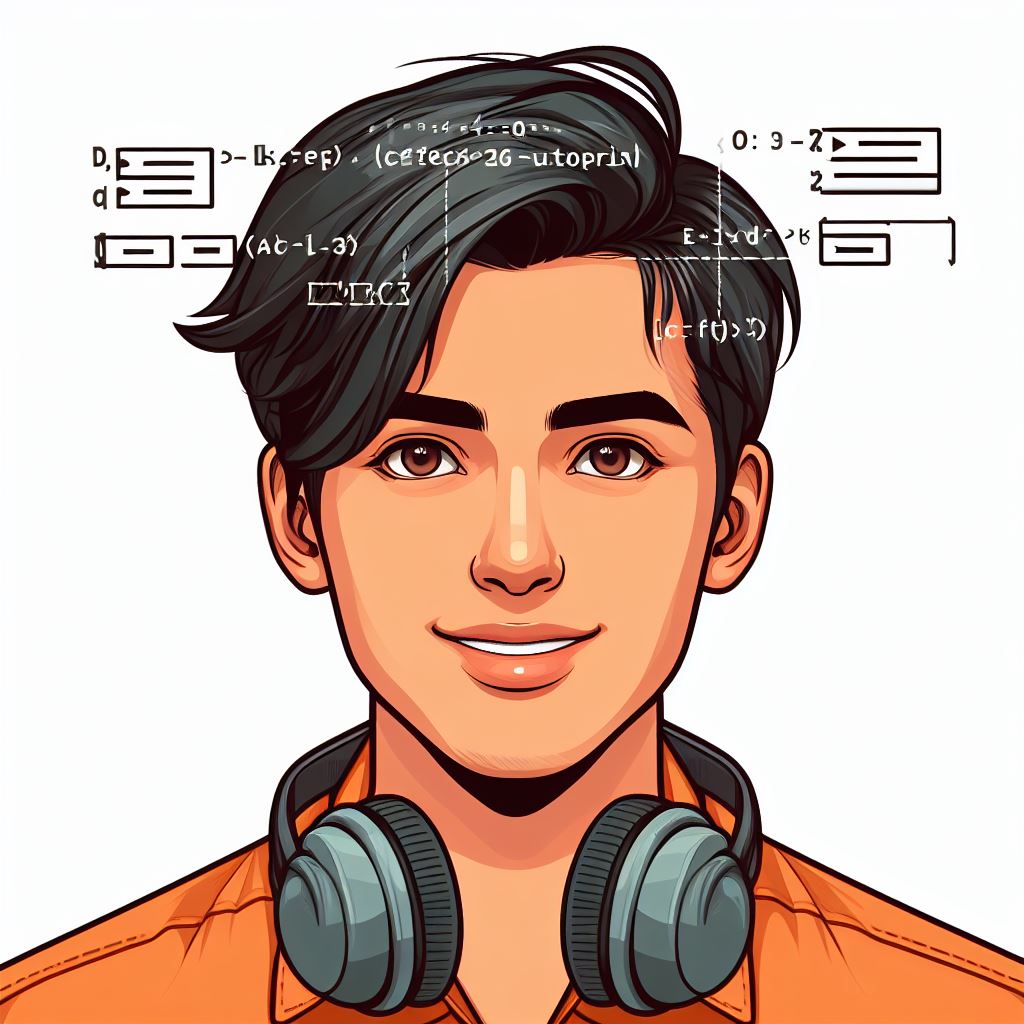
Adding Game Elements
When creating a Java game, it’s important to be able to add game elements such as sprites or images.
This guide will walk you through the process of adding these elements to your game.
Guide for adding sprites or images
First, you’ll need to have the sprites or images that you want to use in your game.
These can be downloaded from various online sources or created by yourself.
Once you have your sprites or images, you need to import them into your Java project.
This can be done by creating a new folder in your project directory and placing the images inside it.
In your Java code, you’ll need to create an instance of the BufferedImage class to hold the image data.
You can do this by using the following code:
Next, you’ll need to create an instance of the Graphics2D class to manipulate the image.
This class extends the Graphics class and provides additional functionality for drawing shapes and images.
To draw the game elements onto the window, you’ll need to override the paintComponent() method of the JPanel class.
Inside this method, you can use the Graphics2D object to draw your images using the drawImage() method.
For example, if you have a BufferedImage called “playerImage”, you can draw it onto the window using the following code:
The “x” and “y” arguments specify the coordinates where you want to draw the image on the window.
You can also use the Graphics2D object to draw other game elements such as shapes and text.
The Graphics2D class provides methods like drawRect(), drawOval(), and drawString() for this purpose.
Remember to call the super.paintComponent() method at the beginning of your overridden paintComponent() method to ensure that the window is properly repainted.
Demonstrating drawing game elements onto the window Let’s demonstrate how to draw game elements onto the window using the example of a simple 2D game where you control a player character.
First, create a new Java project and open the main class containing the main() method.
Import the necessary classes:
Create a class that extends JPanel and override the paintComponent() method:
In the main() method, create an instance of the GamePanel class and add it to a JFrame:
Now you can draw your game elements inside the paintComponent() method of the GamePanel class, using the previously mentioned drawImage() method:
Compile and run your game, and you should see the player character being drawn onto the window!
That’s it! You’ve successfully learned how to add sprites or images to your Java game and draw game elements onto the window.
Have fun creating your own games!
Read: A Guide to Effective Java Exception Handling Techniques
Implementing Game Logic
In this section, we will delve into the important aspects of game loops and their significance in creating a Java game.
Additionally, we will explore the game loop structure using while loops and timers, as well as showcase the integration of user input for controlling game elements.
Introduction to Game Loops and their Importance
Game loops play a crucial role in the execution of a Java game.
They ensure that the game is constantly running and updating at a specific rate, creating the illusion of continuous movement and interaction for the players.
Without a game loop, the game would either freeze or progress too quickly to be enjoyable.
The game loop allows for the separation of game logic and rendering, enabling smooth gameplay even if the rendering process takes longer.
By having a well-structured game loop, developers can control the game’s pace and responsiveness, providing a seamless experience.
Explaining the Game Loop Structure using while Loops and Timers
A widely used approach to implementing game loops in Java is through while loops and timers.
The while loop continuously checks a condition and executes a block of code until the condition becomes false.
In a game loop, the condition might be whether the game is still running or the player has not yet achieved a certain objective.
Here is an example of a game loop structure:
Update game logic
Render game graphics
// Adjust sleep time to maintain a specific frame rate
In this structure, the loop runs until the gameRunning condition is false.
Within the loop, the game logic is updated first, where calculations for game mechanics, collision detection, and AI behavior take place.
After that, the game graphics are rendered, including drawing sprites, backgrounds, and other graphical elements.
The timers, represented by the startTime , endTime , and timeElapsed variables, control the pace of the game loop.
They measure the time taken by each iteration of the loop and adjust the sleep time to maintain a specific frame rate.
The frame rate determines how many times the game logic is updated and the graphics are rendered per second, affecting the smoothness of the gameplay.
Showcasing User Input Integration for Controlling Game Elements
User input is an essential aspect of many games, enabling players to control and interact with game elements.
In Java games, user input can be integrated using various techniques like keyboard and mouse listeners.
For example, to control the movement of a character, the game can listen for key presses related to specific movements and update the character’s position accordingly.
Similarly, mouse listeners can capture events such as clicks or drags, allowing players to interact with objects in the game world.
By integrating user input, Java games become more immersive and engaging, as players have direct control over their in-game actions.
Properly managing user input within the game loop ensures that the game responds to player commands in real-time, enhancing the overall gaming experience.
Therefore, implementing game logic in a Java game involves understanding and implementing game loops, which are crucial for maintaining smooth gameplay.
By using while loops and timers, developers can structure the game loop and control the pace of the game.
Additionally, integrating user input allows players to interact with game elements and adds an extra layer of interactivity to the game.
Mastering these concepts and techniques will enable developers to create captivating and enjoyable Java games.
Collision Detection and Game Over
Collision detection is the process of checking if two objects in a game have collided with each other.
It is a crucial aspect of game development as it enables interactions between different game elements and determines the outcome of these interactions.
Techniques and code examples for implementing collision detection in Java
Implementing collision detection in Java involves various techniques and code examples.
One common technique is the bounding box approach, where each game object is enclosed in a rectangular bounding box.
By checking if the bounding boxes of two objects intersect, we can determine if a collision has occurred.
Here’s an example of how to implement collision detection using bounding boxes in Java:
This code snippet creates bounding boxes for two objects, object1 and object2.
The intersects() method of the Rectangle class checks if the two bounding boxes intersect.
If they do, a collision is detected, and the method returns true; otherwise, it returns false.
Another technique for collision detection is pixel-perfect collision, which involves checking if the pixels of two objects overlap.
This approach provides more precise collision detection but can be computationally expensive.
Handling the game over situation and displaying the appropriate outcome is an essential part of game development.
When a game over condition is met, the player should be informed, and specific actions need to be taken, such as displaying a game over message or resetting the game.
To handle game over situations in Java games, you can use conditional statements and user interface components.
For example, you can have a variable called “gameOver” that is set to true when the game is over.
Based on its value, you can display different outcomes on the screen.
Here’s an example of how to handle game over situations in Java:
In this code snippet, the update() method checks if the gameOver variable is true.
If it is, the displayGameOverMessage() method is called to show a game over message using the JOptionPane class.
The resetGame() method is also called to reset the game state and variables.
Therefore, collision detection is significant in games as it enables interactions between game objects.
Implementing collision detection in Java involves techniques like bounding box and pixel-perfect collision.
Handling game over situations requires displaying appropriate outcomes and taking necessary actions like showing messages or resetting the game.
Adding Sound Effects and Music
Incorporating sound effects and music in games can greatly enhance the gaming experience.
The benefits of adding sound effects and music in games are numerous.
Not only do they make games more engaging and immersive, but they also help to create a more realistic and dynamic environment for players.
One of the main benefits of using sound effects and music in games is that they add an extra layer of immersion.
The sound effects can help to bring the game world to life by creating a sense of realism.
For example, the sound of footsteps can make players feel like they are actually walking in the game world.
Similarly, background music can help to set the mood and atmosphere of the game, whether it’s a fast-paced action game or a calming puzzle game.
Another benefit of incorporating sound effects and music in games is that they can provide important gameplay feedback to players.
For example, sound effects can indicate when an action has been successfully performed or if an enemy is nearby.
This can help players to make decisions and react quickly in the game.
Additionally, music can be used to signal important events or changes in the game, such as reaching a milestone or entering a new level.
To add sound effects and music to a Java game, there are a few steps to follow.
First, you need to have sound files in the desired format, such as WAV or MP3.
These files can be obtained from various sources, including online repositories or by creating your own recordings.
Once you have the sound files, you need to import them into your Java project.
Next, you need to implement audio playback in your Java game.
Java provides a built-in library called javax.sound.sampled for handling audio.
You can use this library to load and play sound files in your game.
To do this, you need to create an instance of the AudioSystem class and use its methods to load and play the sound files.
You can also use the Clip class to control the playback, such as stopping or looping the sound.
Once you have implemented audio playback in your game, you can then play sounds upon specific game events or interactions.
For example, you can play a sound effect when a player collects a power-up or when an enemy is defeated.
This can provide immediate feedback to players and make the game more interactive.
You can also play background music throughout the game to enhance the overall atmosphere.
Therefore, incorporating sound effects and music in games has numerous benefits.
They can enhance immersion, provide gameplay feedback, and create a more dynamic gaming experience.
Adding sound files and implementing audio playback in Java is relatively straightforward using libraries like javax.sound.sampled.
By playing sounds upon specific game events or interactions, developers can further engage players and make the game more enjoyable.
So, don’t underestimate the power of sound in creating captivating Java games.
Testing and Debugging
In the development process of your first Java game, testing and debugging play a crucial role in ensuring the game functions properly.
The Importance of Testing and Debugging Game Code
Testing and debugging your game code is essential to identify and fix any errors or issues that may arise during gameplay.
Without thorough testing, your game may contain bugs or glitches that can significantly impact the user experience.
Debugging allows you to trace and analyze the code’s execution to identify the causes of issues and find effective solutions.
It helps eliminate errors, improve performance, and ensure the game works as intended.
Tips and Techniques for Effective Testing and Debugging in Java
Below are tips and techniques for effective testing and debugging in java
- Utilize println Statements : One simple yet effective technique for debugging is using println statements strategically placed in your code. Print out relevant variables and messages to track the program’s flow and identify any unexpected behavior.
The printed output will provide insights into the value of the variable during runtime, helping you pinpoint potential issues.
- Take Advantage of Debugging Tools: Java offers a variety of debugging tools that can simplify the process of identifying and fixing errors. Integrated Development Environments (IDEs) like Eclipse and IntelliJ provide built-in debuggers, allowing you to step through your code, set breakpoints, and examine variables. By using breakpoints, you can pause the execution at specific points and inspect the state of variables and objects. This technique is invaluable when trying to isolate and fix bugs.
- Test Different Scenarios : Thorough testing involves trying out various scenarios to ensure your game functions correctly in different situations. Test edge cases, input validation, and boundary conditions to detect any unexpected behavior. Consider scenarios like winning or losing the game, playing in different game modes, or entering invalid input to ensure your code handles all scenarios gracefully.
- Use Test-Driven Development (TDD) : Test-Driven Development (TDD) is an effective software development technique that involves creating tests before writing the actual game code. By writing tests first, you can ensure that your code meets the expected functionalities. TDD helps you catch bugs early, avoid regression issues, and maintain a stable codebase. It also encourages modular and easily testable code.
- Learn from Error Messages : Error messages are valuable hints to determine where issues occur and guide you towards potential solutions. Pay close attention to error messages, stack traces, and line numbers provided by the Java compiler or IDE. Understanding these messages allows you to identify the problematic sections and investigate the root cause effectively. Searching for the error online or consulting relevant documentation can often provide insights into potential fixes.
- Collaborate and Seek Feedback: Testing and debugging can be challenging tasks, especially for beginners. Seeking feedback from peers, mentors, or online communities can provide fresh perspectives and help identify issues you might have missed. Collaboration fosters a learning environment and allows you to gain insights from the experience of others. It also helps in finding innovative solutions and improving the overall quality of your game.
Therefore, Testing and debugging are vital steps in game development that ensure your Java game functions optimally.
By applying effective testing techniques and utilizing debugging tools, you can identify, trace, and fix issues efficiently, enhancing the overall gaming experience.
Remember to utilize println statements, leverage debugging tools, test different scenarios, adopt Test-Driven Development, learn from error messages, and seek feedback from others.
Incorporating these practices will not only result in a high-quality game but also enhance your programming skills.
This tutorial covered the step-by-step process of creating a Java game.
We started by setting up the development environment and creating the window for our game.
Next, we added a player character and implemented movement controls using keyboard input.
We also added enemies to our game and implemented collision detection between the player and the enemies.
Then, we added a scoring system and implemented game over conditions.
Now that you have completed this tutorial, I encourage you to experiment and expand upon your game code.
You can add new features such as power-ups, different enemy types, or even additional levels.
By experimenting and adding your own creative ideas, you can make your game unique and more engaging.
To further enhance your Java game development skills, there are several additional resources available.
You can explore online tutorials, books, or even join a Java game development community.
These resources can provide you with more in-depth knowledge and help you take your game development skills to the next level.
Remember, practice is key when it comes to mastering any skill, including coding.
So keep coding, keep experimenting, and keep expanding your game code.
With dedication and persistence, you can become a proficient Java game developer. Good luck!
- Java Memory Management: A Developer’s Guide
- Exploring Java Threads: Concurrency Made Easy
You May Also Like
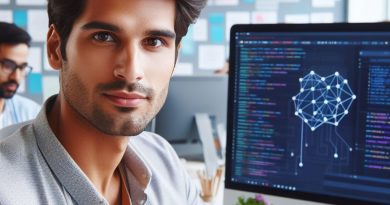
Why Some Professionals Are Learning to Code for Free
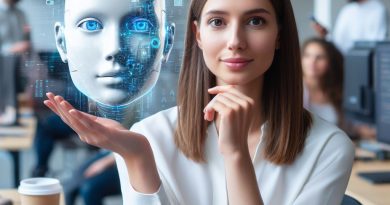
5 Must-Learn Programming Languages for AI Development
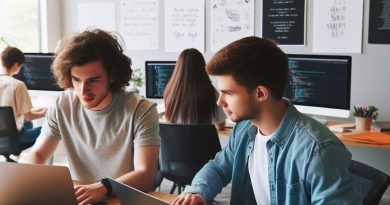
XML Namespaces in SOAP APIs: A Quick Guide
Leave a reply cancel reply.
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- All Articles List
- 18 May 2023
- 88805 views
6 Great Coding Games to Practice Your Java Skills
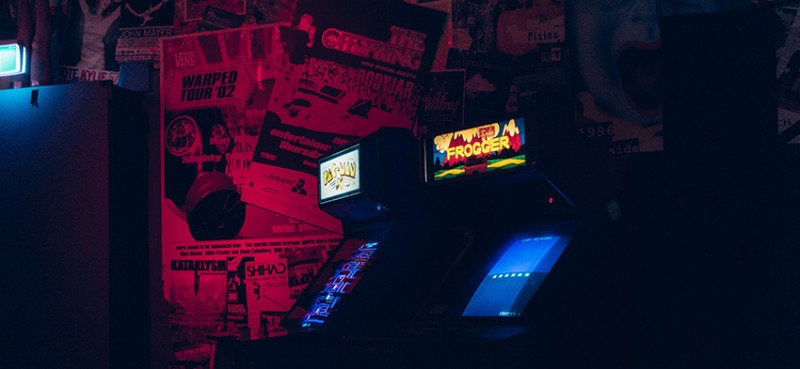
1. Robocode
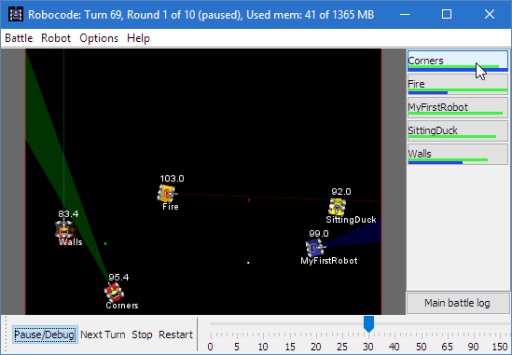
2. Codewars
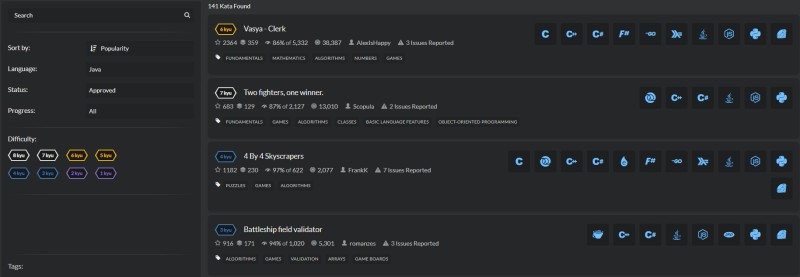
3. CodeMonkey
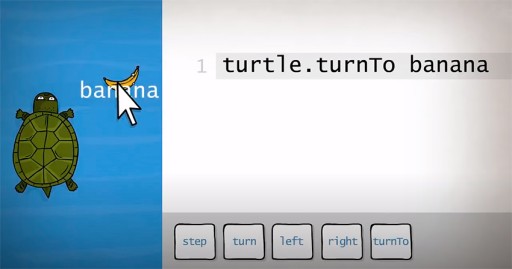
4. Codingame
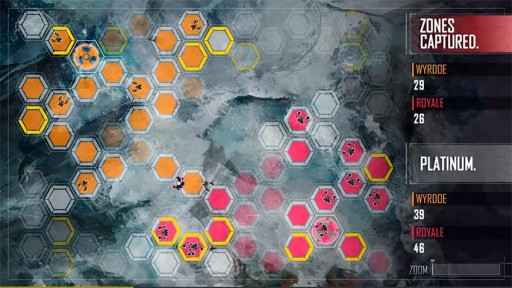
5. Elevator Saga
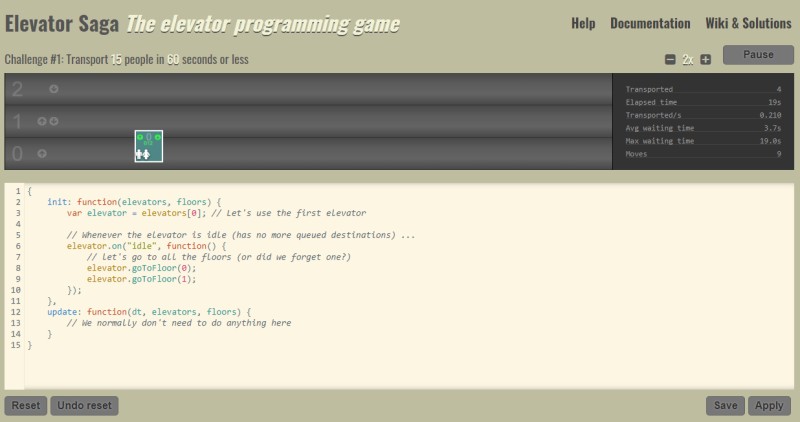
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
Here are 357 public repositories matching this topic...
Freecol / freecol.
FreeCol: FreeCol is a turn-based strategy game based on the old game Colonization, and similar to Civilization. The objective of the game is to create an independent nation.
- Updated Apr 18, 2024
mingli1 / Unlucky
Java LibGDX Android 2D RPG Game
- Updated Oct 10, 2023
mykola-dev / Tetris
The well known brick game written with Kotlin Multiplatform and Jetpack Compose Multiplatform.
- Updated Sep 16, 2022
hdescottes / GdxGame
Turn-based RPG game using Java and libGDX
- Updated Mar 23, 2024
Sesu8642 / FeudalTactics
Strategy game with countless unique and challenging levels.
- Updated Apr 8, 2024
OSSpk / Minesweeper-Desktop-Game
💣 An object-oriented clone of the famous Windows game Minesweeper made in Java-Swing Framework following the Model View Controller (MVC) Architecture. Its a stand-alone desktop game which also provides save and load game functionalities.
- Updated Oct 29, 2023
arminkz / PlantsVsZombies
My own clone of Plants Vs. Zombies game using Java/Swing
- Updated Jun 8, 2022
TheDudeFromCI / WraithEngine
A free, open source, Java game engine library built on top of LWJGL. Designed to act as a use-exactly-what-you-need, no-assumptions framework, WraithEngine is versatile enough to act as a library within other tools or projects, or a full fledged standalone game engine.
- Updated Jan 9, 2022
Rohitjoshi9023 / KBC--Kaun-Banega-Crorepati
It is Core Java based Game based on Indian television game show having best animation as possible in Core java 5000+ lines
- Updated Oct 21, 2022
2003scape / rsc-client
🎮 runescape classic web client
- Updated Jul 18, 2023
kishanrajput23 / Java-Tutorials
Compilation of all study materials related to code with harry java course with assignment solutions
- Updated Aug 18, 2023
ashish2199 / Aidos
A beginner friendly project with aim of creating our own version of Bomberman.
- Updated Oct 24, 2020
mtala3t / Super-Mario-Java-2D-Game
It is a simple Java 2D game like super mario.
- Updated Jun 1, 2018
mynttt / gnuman
Satirical Pacman Clone with Map Editor using JavaFX
- Updated Sep 21, 2022
arminkz / Pacman
A simple java game using swing also with Map Editor and Map Compiler
- Updated Oct 20, 2019
raydac / battleships-resurrection
Attempt to restore game-play of old demo-game Battleships written for E3 2001
- Updated Mar 24, 2024
rafiulgits / SFC-The-Game
A game, developing for create awareness about cancer
- Updated Dec 16, 2023

thederickff / GreatSpace
👾 Space Invaders Game Style
- Updated Oct 14, 2021
Vrekt / OasisGame
A multiplayer RPG built with LibGDX and Netty.
- Updated Apr 16, 2024
onurozuduru / java-shooter-game-project
A simple Java game project for programming class.
- Updated Apr 25, 2016
Improve this page
Add a description, image, and links to the java-game topic page so that developers can more easily learn about it.
Curate this topic
Add this topic to your repo
To associate your repository with the java-game topic, visit your repo's landing page and select "manage topics."
Advertisement
Supported by
Taylor Swift’s ‘Poets’ Arrives With a Promotional Blitz (and a Second LP)
The pop superstar’s latest album was preceded by a satellite radio channel, a word game, a return to TikTok and an actual library. For her fans, more is always welcome.
- Share full article

By Ben Sisario
Taylor Swift was already the most ubiquitous pop star in the galaxy, her presence dominating the music charts, the concert calendar, the Super Bowl, the Grammys.
Then it came time for her to promote a new album.
In the days leading up to the release of “The Tortured Poets Department” on Friday, Swift became all but inescapable, online and seemingly everywhere else. Her lyrics were the basis for an Apple Music word game . A Spotify-sponsored, Swift-branded “ library installation ,” in muted pink and gray, popped up in a shopping complex in Los Angeles. In Chicago, a QR code painted on a brick wall directed fans to another Easter egg on YouTube. Videos on Swift’s social media accounts, showing antique typewriters and globes with pins, were dissected for clues about her music. SiriusXM added a Swift radio station; of course it’s called Channel 13 (Taylor’s Version).
About the only thing Swift didn’t do was an interview with a journalist.
At this stage in Swift’s career, an album release is more than just a moment to sell music; it’s all but a given that “The Tortured Poets Department” will open with gigantic sales numbers, many of them for “ghost white,” “phantom clear” and other collector-ready vinyl variants . More than that, the album’s arrival is a test of the celebrity-industrial complex overall, with tech platforms and media outlets racing to capture whatever piece of the fan frenzy they can get.
Threads, the newish social media platform from Meta, primed Swifties for their idol’s arrival there, and offered fans who shared Swift’s first Threads post a custom badge. Swift stunned the music industry last week by breaking ranks with her record label, Universal, and returning her music to TikTok, which Universal and other industry groups have said pays far too little in royalties. Overnight, TikTok unveiled “The Ultimate Taylor Swift In-App Experience,” offering fans digital goodies like a “Tortured Poets-inspired animation” on their feed.
Before the album’s release on Friday, Swift revealed that a music video — for “Fortnight,” the first single, featuring Post Malone — would arrive on Friday at 8 p.m. Eastern time. At 2 a.m., she had another surprise: 15 more songs. “I’d written so much tortured poetry in the past 2 years and wanted to share it all with you,” she wrote in a social media post , bringing “The Anthology” edition of the album to 31 tracks.
“The Tortured Poets Department,” which Swift, 34, announced in a Grammy acceptance speech in February — she had the Instagram post ready to go — lands as Swift’s profile continues to rise to ever-higher levels of cultural saturation.
Her Eras Tour , begun last year, has been a global phenomenon, crashing Ticketmaster and lifting local economies ; by some estimates, it might bring in as much as $2 billion in ticket sales — by far a new record — before it ends later this year. Swift’s romance with the Kansas City Chiefs tight end Travis Kelce has been breathlessly tracked from its first flirtations last summer to their smooch on the Super Bowl field in February. The mere thought that Swift might endorse a presidential candidate this year sent conspiracy-minded politicos reeling .
“The Tortured Poets Department” — don’t even ask about the missing apostrophe — arrived accompanied by a poem written by Stevie Nicks that begins, “He was in love with her/Or at least she thought so.” That establishes what many fans correctly anticipated as the album’s theme of heartbreak and relationship rot, Swift’s signature topic. “I love you/It’s ruining my life,” she sings on “Fortnight.”
Fans were especially primed for the fifth track, “So Long, London,” given that (1) Swift has said she often sequences her most vulnerable and emotionally intense songs fifth on an LP, and (2) the title suggested it may be about Joe Alwyn, the English actor who was Swift’s boyfriend for about six years, reportedly until early 2023 . Indeed, “So Long” is an epic breakup tune, with lines like “You left me at the house by the heath” and “I’m pissed off you let me give you all that youth for free.” Tracks from the album leaked on Wednesday, and fans have also interpreted some songs as being about Matty Healy , the frontman of the band the 1975, whom Swift was briefly linked to last year.
The album’s title song starts with a classic Swift detail of a memento from a lost love: “You left your typewriter at my apartment/Straight from the tortured poets department.” It also name-drops Dylan Thomas, Patti Smith and, somewhat surprisingly given that company, Charlie Puth, the singer-songwriter who crooned the hook on Wiz Khalifa’s “See You Again,” a No. 1 hit in 2015. (Swift has praised Wiz Khalifa and that song in the past.)
Other big moments include “Florida!!!,” featuring Florence Welch of Florence + the Machine, in which Swift declares — after seven big percussive bangs — that the state “is one hell of a drug.” Jack Antonoff and Aaron Dessner, the producers and songwriters who have been Swift’s primary collaborators in recent years, both worked on “Tortured Poets,” bringing their signature mix of moody, pulsating electronic tracks and delicate acoustic moments, like a bare piano on “Loml” (as in “love of my life”).
As the ninth LP Swift has released in five years, “Tortured Poets” is the latest entry in a remarkable creative streak. That includes five new studio albums and four rerecordings of her old music — each of which sailed to No. 1. When Swift played SoFi Stadium near Los Angeles in August, she spoke from the stage about her recording spurt, saying that the forced break from touring during the Covid-19 pandemic had spurred her to connect with fans by releasing more music.
“And so I decided, in order to keep that connection going,” she said , “if I couldn’t play live shows with you, I was going to make and release as many albums as humanly possible.”
That was two albums ago.
Ben Sisario covers the music industry. He has been writing for The Times since 1998. More about Ben Sisario
Inside the World of Taylor Swift
A Triumph at the Grammys: Taylor Swift made history by winning her fourth album of the year at the 2024 edition of the awards, an event that saw women take many of the top awards .
‘The T ortured Poets Department’: Poets reacted to Swift’s new album name , weighing in on the pertinent question: What do the tortured poets think ?
In the Public Eye: The budding romance between Swift and the football player Travis Kelce created a monocultural vortex that reached its apex at the Super Bowl in Las Vegas. Ahead of kickoff, we revisited some key moments in their relationship .
Politics (Taylor’s Version): After months of anticipation, Swift made her first foray into the 2024 election for Super Tuesday with a bipartisan message on Instagram . The singer, who some believe has enough influence to affect the result of the election , has yet to endorse a presidential candidate.
Conspiracy Theories: In recent months, conspiracy theories about Swift and her relationship with Kelce have proliferated , largely driven by supporters of former President Donald Trump . The pop star's fans are shaking them off .
- CBSSports.com
- Fanatics Sportsbook
- CBS Sports Home
- Champions League
- Motor Sports
- High School
- Horse Racing
Men's Brackets
Women's Brackets
Fantasy Baseball
Fantasy football, football pick'em, college pick'em, fantasy basketball, fantasy hockey, franchise games, 24/7 sports news network.
- CBS Sports Golazo Network
- PGA Tour on CBS
- UEFA Champions League
- UEFA Europa League
- Italian Serie A
- Watch CBS Sports Network
- TV Shows & Listings
The Early Edge
A Daily SportsLine Betting Podcast
With the First Pick
NFL Draft is coming up!
- Podcasts Home
- The First Cut Golf
- Beyond the Arc
- Eye On College Basketball
- NFL Pick Six
- Cover 3 College Football
- Fantasy Football Today
- My Teams Organize / See All Teams Help Account Settings Log Out
2024 RBC Heritage live stream, TV schedule, how to watch online, channel, tee times, radio, golf coverage
There was no rest for the weary with a signature event on tap one week after the masters.
Players have made the short trip from Augusta, Georgia, to Hilton Head Island, South Carolina, for the 2024 RBC Heritage. In total, 69 golfers teed it up on Harbour Town Golf Links as yet another signature event on the PGA Tour's playing calendar rolls on.
Unlike the Genesis Invitational or Arnold Palmer Invitational, the RBC Heritage does not feature a 36-hole cut, meaning the entirety of the field will be around for the weekend. That is music to the ears to those who missed out on the weekend action at the Masters, including the likes of Jordan Spieth, Justin Thomas, Wyndham Clark and Brian Harman.
Spieth, the 2022 champion, looks to kickstart his season after a disappointing showing at Augusta National. He is no stranger to bouncing back at the RBC Heritage, following a missed cut at the year's first major championship with a win a week later in Hilton Head. He's not in contention to win this tournament, but he's certainly playing better than he did last week.
This tournament, like the rest on the PGA Tour these days, goes through Scottie Scheffler. The world No. 1 aims to collect his fourth trophy in his last five tournaments, and he holds the 54-hole lead entering the final day of play. Fresh off slipping on his second green jacket, Scheffler has widened the gap between him and the rest of the world and shows no signs of slowing down. Collin Morikawa and Ludvig Åberg -- just as they did at the Masters -- are breathing down Scheffler's neck on Sunday.
All times Eastern; streaming start times approximated
Round 4 - Sunday
Round starts: 7:15 a.m.
PGA Tour Live: 7:15 a.m. - 6 p.m. -- PGA Tour Live
Early TV coverage: 1-3 p.m. on Golf Channel, fubo (Try for free) Live streaming: 1-3 p.m. on Peacock
Live TV coverage: 3-6 p.m. on CBS Live simulcast: 3-6 p.m. on CBSSports.com and the CBS Sports App
Radio: 1-6 p.m. -- PGA Tour Radio
Our Latest Golf Stories
2024 PGA Championship odds, picks, best bets, field
Cbs sports staff • 5 min read.
Four tied at RBC Heritage as Scheffler makes his move
Kyle porter • 3 min read.
Nelly Korda goes for record fifth straight win
Prize, money purse breakdown for 2024 RBC Heritage
Kyle porter • 1 min read, poston leads rbc heritage, but åberg on his heels, kyle porter • 4 min read.
Is a grand slam in play for Scottie Scheffler?
Share video.
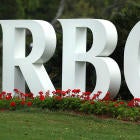
2024 RBC Heritage TV schedule, coverage guide
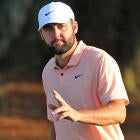
Grand slam in play for Scheffler?
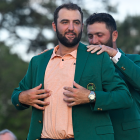
Faith, focus make Scottie unshakable
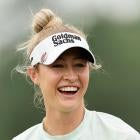
Nelly Korda goes for record fifth straight LPGA win
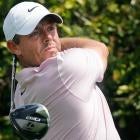
Rory McIlroy emphatically shuts down LIV Golf rumors
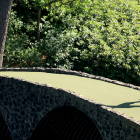
Åberg's trajectory among nine Masters final thoughts
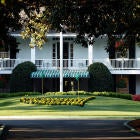
2024 Masters prize money, $20M payout breakdown
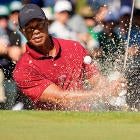
Tiger ends 100th Masters with worst major score
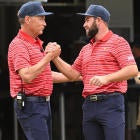
Davis Love III enthused about golf's young stars
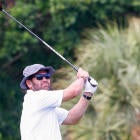
Johnny Damon: How I started loving golf
Filter Results
- Play in browser
- $15 or less
- Last 7 days
- Last 30 days
- Educational
- Interactive Fiction
- Role Playing
- Visual Novel
- Xbox controller
- Gamepad (any)
- Touchscreen
- Voice control
- Oculus Rift
- Leap Motion
- NeuroSky Mindwave
- Accelerometer
- OSVR (Open-Source Virtual Reality)
- Google Daydream VR
- Google Cardboard VR
- Playstation controller
- MIDI controller
- Oculus Quest
- Windows Mixed Reality
- Valve Index
- A few seconds
- A few minutes
- About a half-hour
- About an hour
- A few hours
- Days or more
- Local multiplayer
- Server-based networked multiplayer
- Ad-hoc networked multiplayer
- Color-blind friendly
- Configurable controls
- High-contrast
- Interactive tutorial
- Blind friendly
- Downloadable
- With Steam keys
- In game jams
- Not in game jams
Top free Java Games Games Tools Game assets Comics Books Physical games Albums & soundtracks Game mods Everything else (81 results)
- New & Popular
- Top sellers
- Most Recent
Explore Java games on itch.io · Upload your Java games to itch.io to have them show up here.
New itch.io is now on YouTube!
Subscribe for game recommendations, clips, and more
- FanNation FanNation FanNation
- Swimsuit SI Swimsuit SI Swimsuit
- Sportsbook SI Sportsbook SI Sportsbook
- Tickets SI Tickets SI Tickets
- Shop SI Shop SI Shop
- Free Agency
- What's on TV
© David Kirouac-USA TODAY Sports
Largest Crowd in Women's Hockey History Watches PWHL Game at Montreal's Bell Centre
- Author: Patrick Andres
Even in an era of sustained growth for women's sports around the world, 2024 has stood out.
The NCAA women's tournament rode a compelling cast of characters—led by Iowa guard Caitlin Clark—into the monoculture, and its championship outdrew the men's national title game . The NWSL is in year one of a monster new TV contract.
Because of this, the success of the Professional Women's Hockey League has flown under the radar a bit. But what encouraging success it has been.
On Saturday, 21,105 fans came out to watch Montreal lose 3–2 in overtime to Toronto at the Bell Centre in Montreal. That is the largest crowd ever to see a women's hockey game, breaking a record set Feb. 16... when the two teams played in Toronto.
Toronto forward and Wisconsin product Sarah Nurse, a 2022 Olympic gold medalist for Canada, scored two goals—including the winner 13 seconds into overtime.
Founded in 2023, the PWHL is a six-team circuit with squads in Boston, Minnesota, Montreal, New York, Ottawa and Toronto.
Latest News
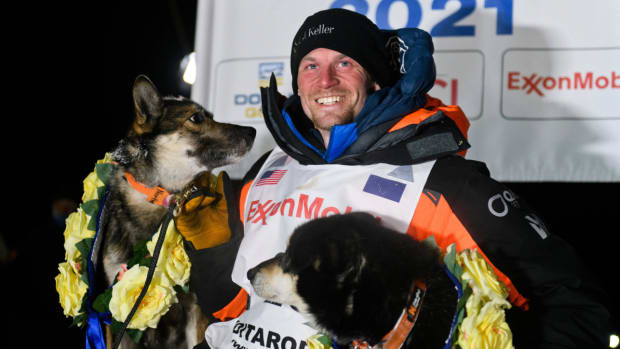
Iditarod Musher Kills, Guts Moose Who Injured Sled Dog During Race
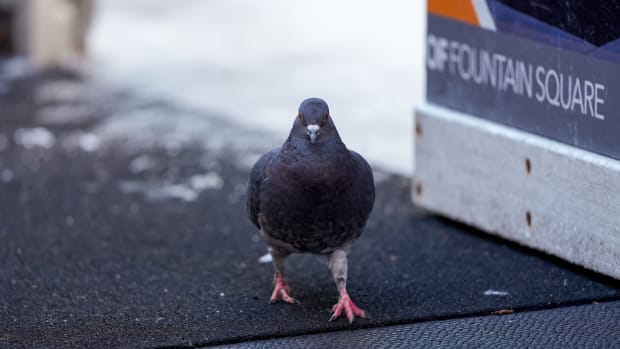
Taiwanese Racing Pigeon Beats Allegations of Spying for China, Becomes Global Celebrity
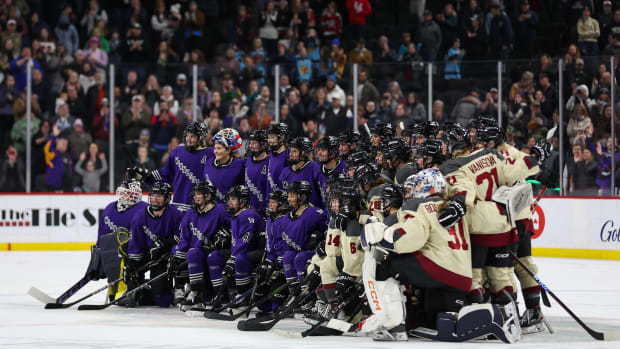
PWHL Sets Pro Women’s Hockey Attendance Record With Minnesota vs. Montreal Game
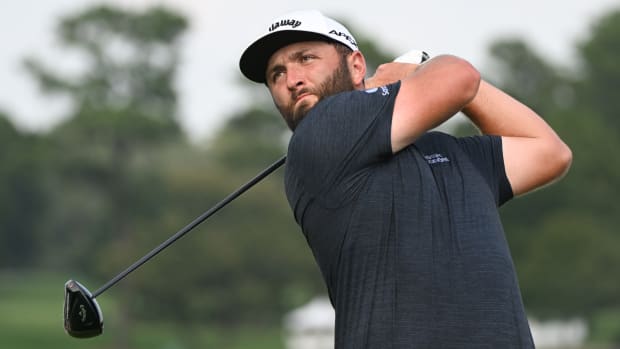
SI:AM | Jon Rahm’s Defection Throws a Wrench in Saudi–PGA Tour Agreement
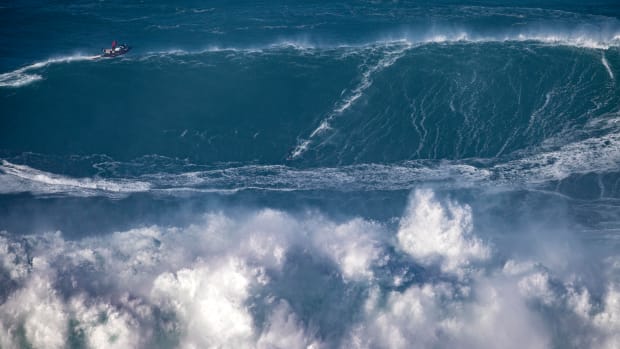
Come Hell or High Water, Surfing Nazare’s 100-Foot Waves Is a Laureano Family Affair
Advertisement
Miami heat at boston celtics game 1 odds, picks and predictions, share this article.
The Miami Heat visit the Boston Celtics Sunday in Game 1 of a best-of-7 Eastern Conference, 1st-round series. Tip from TD Garden is scheduled for 1 p.m. ET (ABC). Let’s analyze BetMGM Sportsbook’s lines around the Heat vs. Celtics odds and make our expert NBA picks and predictions .
Season series: Celtics won 3-0
Despite missing leading scorer F Jimmy Butler , who suffered an MCL tear in the previous game, the Heat took down the Chicago Bulls 112-91 Friday in a play-in game — and covered as 2-point home favorites — to earn the right to play as the No. 8 seed in the East. G Tyler Herro scored a game-high 24 points with a team-high 10 rebounds for a double-double. Four Miami players scored in double figures, while the team shot 46.3% from the field in the win.
Boston ended its season with back-to-back wins after beating the Wizards 132-122 April 14 to push as a 10-point home favorite in a game that didn’t affect the standings. G Payton Pritchard scored a game-high 38 points while dishing out 12 assists — also a game-high — for a double-double. Five Celtics players scored in double figures, while the team shot 57.3% from the field in the win.
Heat at Celtics odds
Provided by BetMGM Sportsbook ; access USA TODAY Sports Scores and Sports Betting Odds hub for a full list. Lines last updated at 2:58 a.m. ET.
- Moneyline (ML) : Heat +675 (bet $100 to win $675) | Celtics -1100 (bet $1100 to win $100)
- Against the spread (ATS) : Heat +13.5 (-110) | Celtics -13.5 (-110)
- Over/Under (O/U) : 208.5 (O: -115 | U: -108)
Heat at Celtics key injuries
- F Jimmy Butler (knee) out
- F Kevin Love (ankle) available
- G Josh Richardson (shoulder) out
- F Duncan Robinson (back) questionable
- G Terry Rozier (neck) out
- C Luke Kornet (calf) out
For most recent updates: Official NBA injury report .
Play our free daily Pick’em Challenge and win! Play now !
Heat at Celtics picks and predictions
Celtics 115, Heat 104
There is no value on the Celtics (-1100), who are 37-4 at home this season.
Against the spread
LEAN HEAT +13.5 (-110).
Miami covered the spread in 2 of the 3 matchups against Boston this season. The Heat are 7-3 against the spread (ATS) in their last 10 games including 6-2 ATS in their last 8. Boston is 0-1-2 ATS in its last 3 games as a 9-point or more favorite. While Miami is without Butler, 13.5 points is too large of a spread to back Boston.
BET OVER 208.5 (-115).
Miami has hit the Over in 8 of its last 10 games, while Boston has hit the Over in 7 of its last 10.
Miami has hit the Over in 3 of its last 4 and has scored 112 or more in 3 of those 4 games. It has scored 109 or more in 7 of its last 10.
Boston has hit the Over in each of its last 3 and has scored 131 or more in back-to-back games. It has scored 109 or more in 7 of its last 10.
For more sports betting picks and tips , check out SportsbookWire.com and BetFTW .
Follow @seth_orlemann on Twitter/X . Follow SportsbookWire on Twitter/X and like us on Facebook .
Access more NBA coverage: HoopsHype | Bulls Wire | Celtics Wire | Nets Wire | Rockets Wire | Sixers Wire | Spurs Wire | Thunder Wire | Warriors Wire | LeBron Wire | Rookie Wire | List Wire
Like this article?
Sign up for our newsletter to get updates to your inbox, and also receive offers from us, our affiliates and partners. By signing up you agree to our Privacy Policy
More NBA Picks and Predictions
Fanatics sportsbook promo - bet & get up to $1000 in bonus bets for nba playoffs, nhl odds, dallas mavericks at la clippers game 1 odds, picks and predictions, nba betting promos & bonuses | best nba playoff betting sites for first-round games.
Gannett may earn revenue from sports betting operators for audience referrals to betting services. Sports betting operators have no influence over nor are any such revenues in any way dependent on or linked to the newsrooms or news coverage. Terms apply, see operator site for Terms and Conditions. If you or someone you know has a gambling problem, help is available. Call the National Council on Problem Gambling 24/7 at 1-800-GAMBLER (NJ, OH), 1-800-522-4700 (CO), 1-800-BETS-OFF (IA), 1-800-9-WITH-IT (IN). Must be 21 or older to gamble. Sports betting and gambling are not legal in all locations. Be sure to comply with laws applicable where you reside.

New Orleans Pelicans at Oklahoma City Thunder Game 1 odds, picks and predictions

Fanatics Sportsbook Massachusetts Promo Delivers $50 No-Deposit Bonus for Celtics, Bruins

Indiana Pacers at Milwaukee Bucks Game 1 odds, picks and predictions
Sports betting resources.
Sports Scores and Odds Hub Sports Betting Legality Map Parlay Calculator
Most Popular
Los angeles lakers at denver nuggets game 1 odds, picks and predictions, phoenix suns at minnesota timberwolves game 1 odds, picks and predictions, philadelphia 76ers at new york knicks game 1 odds, picks and predictions, toronto maple leafs at boston bruins game 1 odds, picks and predictions.
Please enter an email address.
Thanks for signing up.
Please check your email for a confirmation.
Something went wrong.
- Bahasa Indonesia
- Bahasa Melayu
JAVA GAMES GENRE DANCE
Android Games >
Guitar Rock Tour
Description, guitar rock tour java game, ratings & reviews (0), review summary.
There are currently no reviews for this game
Be the first to review this game
Register Register a PHONEKY account to post reviews with your name, upload and store your favourite mobile apps, games, ringtones & wallpapers.
Register or Sign in to PHONEKY
- GUITAR ROCK TOUR
You might also like:
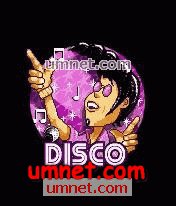
Guitar Hero III Mobile: Legends of Rock
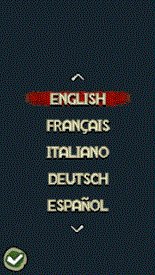
Guitar Hero: Warriors Of Rock Mobile
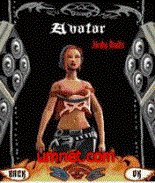
Guitar Hero: World Tour Mobile
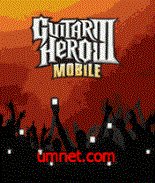
Guitar Hero III: Backstage Pass
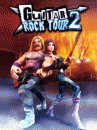
Guitar Rock Tour 2
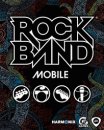
Rock Band Mobile
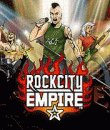
Rock City Empire
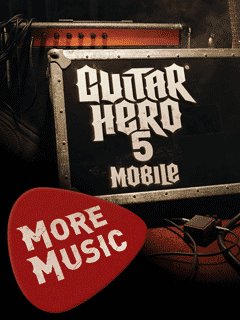
Guitar Hero 5 Mobile: More Music
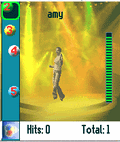
Dance Off Disco
Miami nights: singles in the city, mtv cribs: dj mansion.
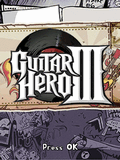
Guitar Hero III Mobile: Song Pack 1
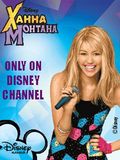
Hannah Montana: Secret Star
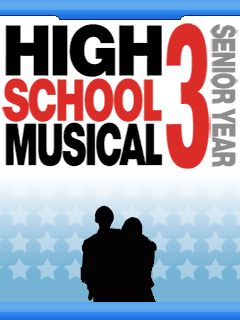
High School Musical 3: Senior Year
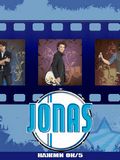
Hip Hop All Star
Guitar hero world tour: backstage pass.
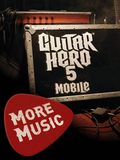
Mobile Air Guitar
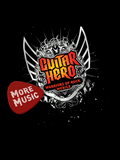
Guitar Hero: Warriors Of Rock Mobile More Music
Rock band reloaded.
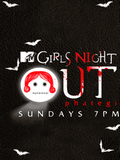
MTV Girls Night Out
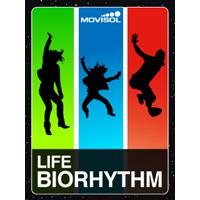
Life Biorhythm
Other versions:.
- NEW 4.1 360x640 1.0 MB 27K
- 4.5 360x640 1.05 MB 974 (Landscape / Touch / Multilanguage)
- NEW 1.0 352x416 506 KB 27K
- NEW 4.4 240x320 612 KB 27K
- 4.2 240x320 644 KB 3K (Touch)
- 4.4 240x320 644 KB 1K (Touch)
- 4.2 240x320 644 KB 1K (Touch / Es)
- 4.4 240x320 585 KB 974 (Touch)
- 4.4 240x320 644 KB 957 (Touch)
- 4.4 240x320 690 KB 723 (Spanish)
- 3.6 240x320 613 KB 496
- 3.0 240x320 644 KB 435
- 2.4 240x320 613 KB 416 (Multilanguage)
- NEW 4.1 208x208 278 KB 27K
- NEW 4.2 176x220 272 KB 27K
- NEW 4.2 128x160 435 KB 27K
- NEW 3.4 128x128 113 KB 27K
JAVA GAMES JAVA APPS ANDROID GAMES HTML5 GAMES SYMBIAN GAMES

PHONEKY: JAVA GAMES & APPS!
Download for Free!
Download your favorite Java games for free on PHONEKY!

Play Minecraft Games with Game Pass

ALSO AVAILABLE ON:
Minecraft is available to play on the following platforms:

*Mac and Linux are compatible with Java Edition only.

Minecraft 1.20.5 Release Candidate 2
A Minecraft Java Release Candidate
We're honestly starting to lose count at this point. Anyways! Here's 1.20.5 Release Candidate 2 coming in clutch with some bona fide bug fixes to round off the week.
Happy weekend mining!
Fixed bugs in 1.20.5 Release Candidate 2
- MC-270862 - Firework Star item displays have inconsistent color updates
- MC-270902 - Game unpauses when credits are played via pause menu
- MC-270916 - Villagers can sell experimental enchantments without the 1.21 experiment enabled
Get the Release Candidate
Release Candidates are available for Minecraft: Java Edition. To install the Release Candidate, open up the Minecraft Launcher and enable snapshots in the "Installations" tab.
Testing versions can corrupt your world, so please backup and/or run them in a different folder from your main worlds.
Cross-platform server jar:
- Minecraft server jar
Report bugs here:
- Minecraft issue tracker !
Want to give feedback?
- For any feedback and suggestions on our upcoming 1.21 features, head over to the dedicated Feedback site category . You can also leave any other feedback on the Feedback site . If you're feeling chatty, join us over at the official Minecraft Discord .

SHARE THIS STORY
Community creations.
Discover the best add-ons, mods, and more being built by the incredible Minecraft community!
Block...Block...Block...

IMAGES
VIDEO
COMMENTS
Backtracking Algorithm for Knight's tour . Following is the Backtracking algorithm for Knight's tour problem. If all squares are visited print the solution Else a) Add one of the next moves to solution vector and recursively check if this move leads to a solution. (A Knight can make maximum eight moves. We choose one of the 8 moves in this step).
Publisher: Nokia.Platform: Java Mobile.=====Nokia - Java Games OST Playlist: https://youtube.com/playlist?list=PLwlJ97GcHiDxz9a...
The Knight's Tour Problem in Java. The Knight's Tour Problem is a classic problem in computer science, mathematics, and chess. The Knight's Tour Problem involves finding a series of moves that a knight on a chessboard can make in order to visit every square only once. We will be developing a Java program that utilizes backtracking to solve this ...
Publisher: Gameloft.Release: 2008.Platform: Java Mobile.- Playlist:[00:00] - 01. Alive (Stephan Endemann / Dragan Hecimovic) - Main Theme.[03:08] - 02. Cry ...
The tutorial uses Java Swing to create the games. E-book. A unique e-book Java 2D games programming is available on ZetCode. The e-book is in PDF format and has 115 pages. Related tutorials. You might also want to look at the Java tutorial, Displaying image in Java tutorial, the Java Swing tutorial, the JavaFX tutorial, or the Java 2D tutorial.
Publisher: Gameloft.Release: 2007.Platform: Java Mobile.- Playlist:[00:00] - 01. Banquet (Kele Okereke, Russell, Lissack, Gordon Moakes & Matt Tong) - Main ...
Download Guitar Hero: World Tour Mobile game for mobiles - one of the best Java games! At PHONEKY Free Java Games Market, you can download mobile games for any phone absolutely free of charge. Nice graphics and addictive gameplay will keep you entertained for a very long time. At PHONEKY, you will find many other games and apps of different ...
If you prefer a more brain-twistery sort of game that requires problem- and puzzle-solving skills, you should check out Codingame. Codingame offers multiple challenges and brain teasers that you have to solve to proceed. You'll need to write code to get through the puzzles, and you can use one of over 25 supported languages, including Java.
Step-by-step Instructions to Create a New Java Project: Open your Java Integrated Development Environment (IDE). Create a new Java project by selecting "File" -> "New" -> "Java Project". Name your project and select the desired location to save it. Click "Finish" to create the project.
1. An old heuristic which works very well for knight's tour (even on paper) is always jump to the MOST restricted square, that is a place where the knight has the least moves. If there are more than one square with same restrictions then choose one of them at random.
Java is a widely used game development programming language because of its versatility. It is one of the go-to options for developers to create apps and games on mobile and desktop. In fact, some of the top mobile games are Java-developed. Games like Minecraft and Asphalt 6 are a testament to its potential for impressive gameplay, graphics, and ...
CodeMonkey. 4. Codingame. Codingame is another popular web platform for developers to practice their coding skills by solving increasingly difficult puzzles. Codingame supports over 25 programming languages, so you won't be limited just to Java, which is also on the list of course.
Golf Tour Java Game. Golf Tour 3D is a full-featured 3D golf simulation featuring and entertaining single mode. Golf Tour 3D delivers all the elements of a golf game you would expect including a customizable set of golf clubs, a range of match and stroke play competitions, male and female golfer graphics and a range of golfer abilities. Info Info.
Download DJ Mix Tour game for mobiles - one of the best Java games! At PHONEKY Free Java Games Market, you can download mobile games for any phone absolutely free of charge. Nice graphics and addictive gameplay will keep you entertained for a very long time. At PHONEKY, you will find many other games and apps of different genres, from adventure ...
Add this topic to your repo. To associate your repository with the java-game topic, visit your repo's landing page and select "manage topics." GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
Get the Release Candidate. Release Candidates are available for Minecraft: Java Edition. To install the Release Candidate, open up the Minecraft Launcher and enable snapshots in the "Installations" tab. Testing versions can corrupt your world, so please backup and/or run them in a different folder from your main worlds. Cross-platform server jar:
Overnight, TikTok unveiled "The Ultimate Taylor Swift In-App Experience," offering fans digital goodies like a "Tortured Poets-inspired animation" on their feed. Before the album's ...
The Colorado Avalanche (50-25-7) hit the road to face the Winnipeg Jets (52-24-6) in Game 1 of their 1st-round series at Canada Life Centre. Puck drop is set for 7 p.m. ET (ESPN2). Below, we analyze BetMGM Sportsbook's lines around the Avalanche vs. Jets odds, and make our expert NHL picks and predictions.. The Avs are in the unusual spot of 3rd place in the Central Division as they ...
Want more? Explore the library at https://www.codecourse.com/lessonsOfficial sitehttps://www.codecourse.comTwitterhttps://twitter.com/teamcodecourse
This tournament, like the rest on the PGA Tour these days, goes through Scottie Scheffler. The world No. 1 aims to collect his fourth trophy in his last five tournaments, and he holds the 54-hole ...
Download 4x4 Extreme Rally: World Tour game for mobiles - one of the best Java games! At PHONEKY Free Java Games Market, you can download mobile games for any phone absolutely free of charge. Nice graphics and addictive gameplay will keep you entertained for a very long time. At PHONEKY, you will find many other games and apps of different ...
Find Java games like Dungeon Of Keys, The Path to Power (demo), blasterma, Overkill!, Granny Online on itch.io, the indie game hosting marketplace itch.io Browse Games Game Jams Upload Game Developer Logs Community
On Saturday, 21,105 fans came out to watch Montreal lose 3-2 in overtime to Toronto at the Bell Centre in Montreal. That is the largest crowd ever to see a women's hockey game, breaking a record ...
The Miami Heat visit the Boston Celtics Sunday in Game 1 of a best-of-7 Eastern Conference, 1st-round series. Tip from TD Garden is scheduled for 1 p.m. ET (ABC). Let's analyze BetMGM Sportsbook's lines around the Heat vs. Celtics odds and make our expert NBA picks and predictions.. Season series: Celtics won 3-0. Despite missing leading scorer F Jimmy Butler, who suffered an MCL tear in ...
The Series of Guitar Rock Tour Video Game for Java MobileList :01.Guitar Rock Tour02.Guitar Rock Tour 2You can tell if I miss one of the games in this videoE...
In Java, variables begin with lowercase letters and follow camelCase, i.e. go, look, etc. Things beginning with uppercase letters include things like class names, i.e. Adventure1, as you already have. It's good to get into this habit now, it makes code easier to read for everybody involved, and can help minimize confusion.
Download Guitar Rock Tour game for mobiles - one of the best Java games! At PHONEKY Free Java Games Market, you can download mobile games for any phone absolutely free of charge. Nice graphics and addictive gameplay will keep you entertained for a very long time. At PHONEKY, you will find many other games and apps of different genres, from ...
Pre-Releases are available for Minecraft: Java Edition. To install the Pre-Release, open up the Minecraft Launcher and enable snapshots in the "Installations" tab. Testing versions can corrupt your world, so please backup and/or run them in a different folder from your main worlds. Cross-platform server jar: Minecraft server jar. Report bugs here:
Get the Release Candidate. Release Candidates are available for Minecraft: Java Edition. To install the Release Candidate, open up the Minecraft Launcher and enable snapshots in the "Installations" tab. Testing versions can corrupt your world, so please backup and/or run them in a different folder from your main worlds. Cross-platform server jar:
1. I'm developing a 2D Brick Breaker style game in Java and want to apply some real time physics to it, especially to the paddle/ball interections. Nothing too complex; just applying some of the physics principles, like: friction to transfer horizontal movement from the curved, hemisferic shaped paddle to the ball in order to change its ...