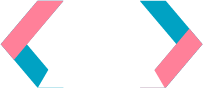

Detect Whether a Device is iOS or Not Using JavaScript
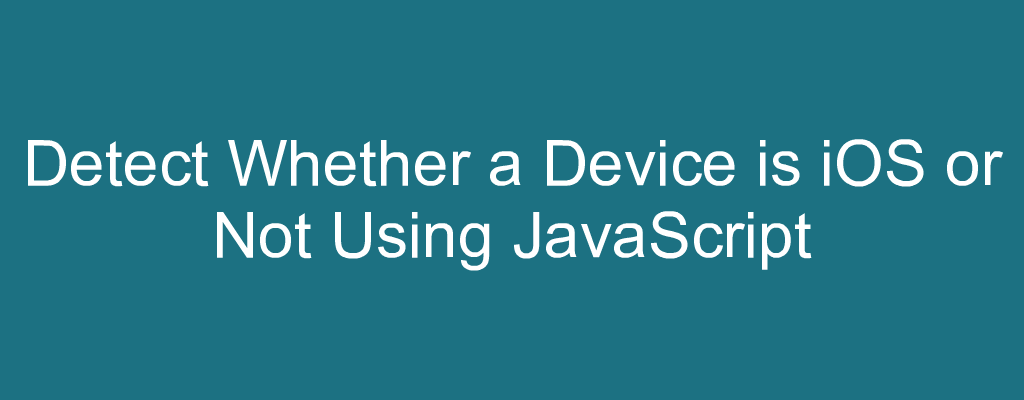
JavaScript is a high-level, interpreted programming language that is widely used for developing dynamic and interactive web applications. Its versatility and ease of use have made it one of the most popular programming languages in the world. In this tutorial, we’ll explore how to use JavaScript to detect whether a device is running iOS or not.
Knowing the type of device your users are accessing your web application from is crucial as a software developer. This information can be used to provide a better user experience or to customize the layout and functionality of your web application. In this section, we’ll explore three different methods for determining if a device is running iOS using JavaScript:
User-Agent Detection
Navigator.platform detection, feature detection.
Each method has its own pros and cons, and it’s important for us to choose the right one for our specific use case. We’ll go over each method in detail, explaining how it works and providing code examples to help us implement it in our own projects.
One of the methods we can use to detect whether a device is running iOS or not is User-Agent Detection. This method involves examining the user-agent string of the device to determine the operating system and browser being used.
We can use the JavaScript navigator.userAgent property to retrieve the user-agent string and then check if it contains the word “iPhone”, “iPad”, or “iPod”. This method is simple to implement and can provide quick results, but it’s important to keep in mind that the user-agent string can be easily altered, making it not the most reliable method for detecting iOS devices.
Here’s an example of how to use the User Agent string to detect if a device is running iOS:
In the above code snippet, we are using the test() method of a regular expression to check if the string stored in the navigator.userAgent property contains any of the substrings “iPad”, “iPhone”, or “iPod”. The regular expression is surrounded by forward slashes (/) and is enclosed within the test() method.
If the test returns true, which means that the navigator.userAgent string contains one of the substrings, then we know that the device is running iOS and we print “This is an iOS device.” to the console and vice-versa.
Let’s say, for example, we run this code on an iPhone. The output would be:
And if we run this code on a device that is not running iOS, the output would be:
We can also determine if a device is running on iOS by checking the navigator.platform property. This property gives us a string value that represents the platform on which our browser is running. By evaluating this property, we can find out if our device is an iOS device or not. Essentially, we just have to check if the navigator.platform is equal to ‘iPad’, ‘iPhone’, or ‘iPod’, and if it is, then we know our device is an iOS device.
Here’s how we can use the navigator.platform property to detect if a device is running iOS:
In the above code snippet, we use of the navigator.platform property to detect if a device is running iOS or not. As we know, the navigator.platform property returns a string representing the platform on which the browser is running.
In this code, we’re checking if the navigator.platform is equal to ‘iPad’, ‘iPhone’ or ‘iPod’. If it is, we log the message “This is an iOS device.” to the console. Otherwise, we log the message “This is not an iOS device!” to the console.
It’s important to note that this method is not foolproof, as some non-iOS devices can have similar platform strings. However, this method is widely used and considered reliable in most cases.
Feature Detection is another method to determine if a device is running iOS or not. This method involves checking the availability of specific features that are unique to iOS devices. It involves checking for touch events, max touch points and other iOS specific functionalities to determine the device type.
For instance, the MaxTouchPoints property is used to determine the number of touchpoints supported by a device. If a device supports more than one touch point, it’s likely not running iOS. On the other hand, the ‘ontouchstart’ in window check is used to detect if a device has the ability to detect touch events. If this check returns true, it means the device is running iOS.
Let’s see how we can use feature detection to find out if the device is an iOS device or not.
The above code checks three specific properties: ontouchstart, navigator.MaxTouchPoints , and navigator.msMaxTouchPoints . If any of these properties exist in the current device, the code logs “This is an iOS device.” to the console. Otherwise, it logs “This is not an iOS device!”.
By checking these properties, we’re essentially checking if the device is touch-enabled, which is a common characteristic of iOS devices. However, it’s important to note that this method may not always be 100% accurate, as some non-iOS devices may also have touch capabilities. But overall, it’s a good starting point for feature detection in determining the type of device being used.
In this tutorial, we delved into the different ways to figure out if a device is running on iOS or not. We walked through the User-Agent detection method and talked about how it uses the navigator.userAgent property to identify the device type. Then, we went over the Navigator.platform detection method and how it checks the navigator.platform property to determine if the device is iOS.
We also touched upon the Feature Detection method, which involves checking for the presence of certain features that are only found on iOS devices. To wrap up, we provided examples of code to help illustrate how each method works.
How to detect iPad or iPhone web view via JavaScript?
- Post author By John Au-Yeung
- Post date May 21, 2022
- No Comments on How to detect iPad or iPhone web view via JavaScript?

Sometimes, we want to detect iPad or iPhone web view via JavaScript.
In this article, we’ll look at how to detect iPad or iPhone web view via JavaScript.
To detect iPad or iPhone web view via JavaScript, we check window.navigator.userAgent and window.navigator.standalone .
For instance, we write
to get the user agent with window.navigator.userAgent
And window.navigator.standalone being false means that the page is opened in a web view in an app.
Then we check if the user agent is safari and ios with test .
We then check if the page is opened in ios . If it is then ios is true .
And then in the inner if block, we check for combinations of standalone and safari to see if it’s opened in a Safari web view.
If the page is opened in a web view, then !standalone && !safari is true .
Related Posts
Sometimes, we want to detect iPad Pro as iPad with JavaScript. In this article, we'll…
Sometimes, we want to detect Android phone via JavaScript. In this article, we'll look at…
Sometimes, we want to listen for scroll event for iPhone or iPad with JavaScript. In…
By John Au-Yeung
Web developer specializing in React, Vue, and front end development.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.


Member-only story
Essential JavaScript Functions for Detecting User’s Device Characteristics
Learn how to detect ios, android, fullscreen mode, firefox, safari, and more with javascript..

David Dal Busco
Time and again, I’ve encountered the need to implement features in my web-based projects that specifically target certain types of devices. Each time, I find myself falling back on the same set of utilities I’ve been using for years. It’s high time I shared these invaluable tools in a blog post.
In this article, you’ll gain access to a curated collection of JavaScript functions for identifying iOS and Android devices, detecting fullscreen mode, distinguishing Firefox from Safari, and more.
It’s important to be aware that some of these methods are based on parsing the navigator user-agent string, which might see changes in the future as Google plans to reduce the information provided by this string.
Let’s start with the detection of a mobile device. I determine if a user is using my applications in a browser-based environment that might be a mobile device by testing whether the user is using a pointing device with limited accuracy, such as a touch screen, and which is not a precise device such as a mouse.
The matchMedia interface returns an object that can be used to determine if the document or web page matches a specific media query. To test the pointing device, I utilize the any-pointer CSS media feature.
Identifying an iPad device involves inspecting the user-agent, but it also necessitates combining this data with the previous mobile detection function. This is because Safari presents a string similar to that of desktop or laptop devices.
On older versions of iPadOS, the user-agent used to include the indentation; therefore, I also check if the string might contain this information.
Note that the i in the regex showcased in this article stands for insensitive meaning it performs a test without considering case sensitivity.
I combine iPhone and iPod into a single function because their user experience is similar for my projects. Both can be tested by querying the user-agent, which contains their indication.
I assume you already know the answer: to detect if a device is running iOS, we can simply use the two previous functions.
Similar to iPhones, detecting Android devices is straightforward because the necessary information is provided in the user-agent string.
isAndroidTablet
According to my tests, an Android tablet is an Android device, which is strangely not identified by the keyword mobile in its user-agent string.
Once, I also had to determine if the user’s device was held in portrait mode. Instead of comparing screen dimensions, I utilized the matchMedia interface to determine the orientation of the device.
isLandscape
Similarly, we can implement the opposite of the previous function to determine if the device’s orientation is in landscape mode.
isFullscreen
To determine if a browser is used in fullscreen mode, I typically use the document fullscreenElement property, but I also check vendor-specific properties that are supposed to reflect the same information.
While the navigator vendor property is marked as deprecated by MDN, I still rely on it to check if a browser is Safari.
You might argue that this would also return true on an iPhone when, for example, Chrome is used. To that, I would offer a friendly reminder that any browser running on an iPhone or iPad is, unfortunately, still Safari due to Apple’s vendor lockdown monopoly.
Knowing if an application is running in a Firefox browser can be determined by checking the user-agent string.
Finally, if your application focuses on internationalization, this is how I determine if the content should be read from right to left. I set the dir attribute at the top level of the document.
Thank you for reading! For more exciting coding content, please consider following me on Twitter / X . 🤓
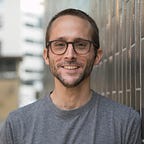
Written by David Dal Busco
Freelancer by day | Creator of Juno.build by night
More from David Dal Busco and ITNEXT
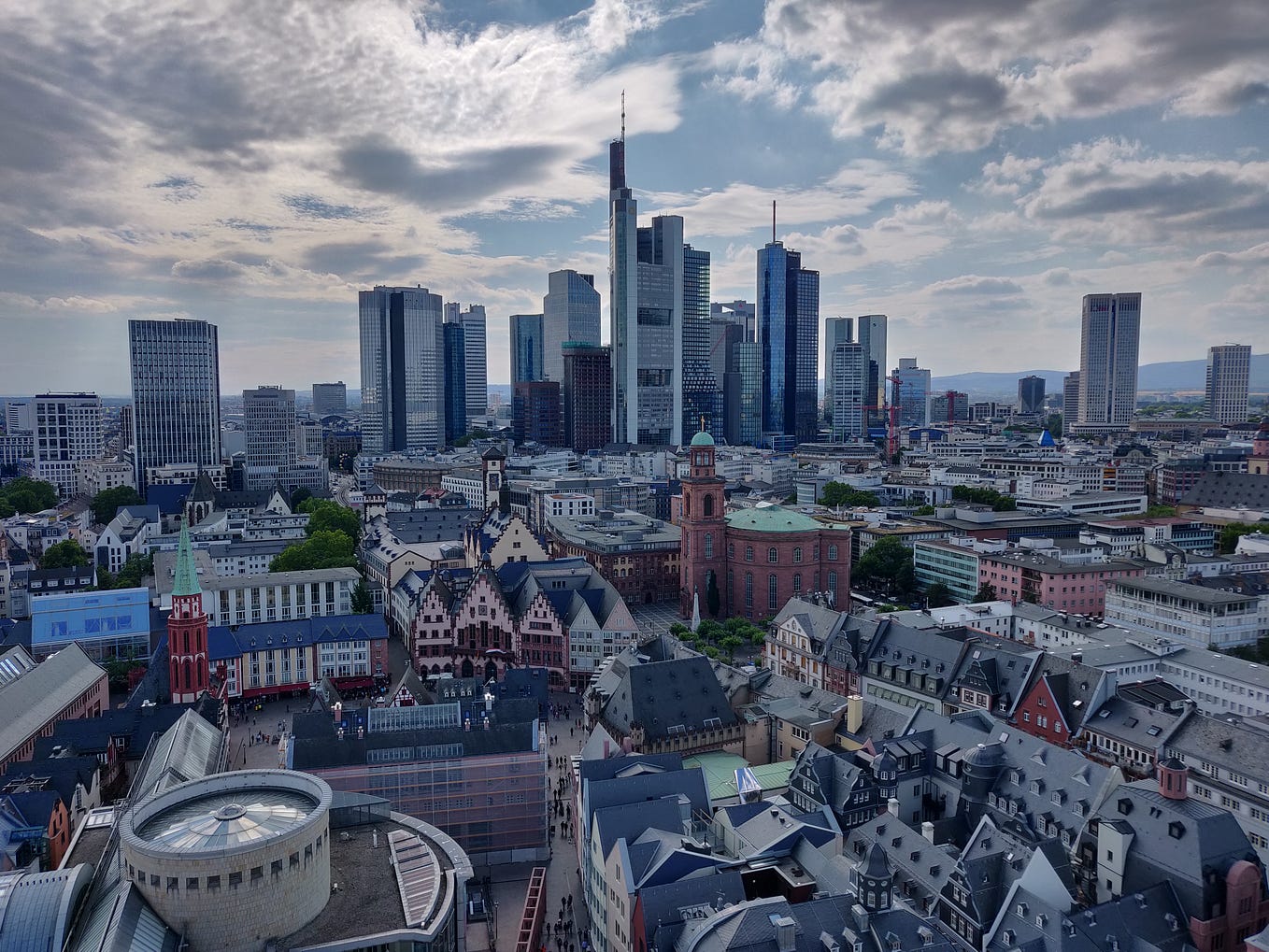
Take photo and access the picture library in your PWA (without plugins)
How to access camera and photo library in a progressive web app using web technologies and no plugins.
![javascript detect ipad safari Benchmark results of Kubernetes network plugins (CNI) over 40Gbit/s network [2024]](https://miro.medium.com/v2/resize:fit:1358/1*JoN-fag4InHrYL2mR6pX-g.png)
Alexis Ducastel
Benchmark results of Kubernetes network plugins (CNI) over 40Gbit/s network [2024]
This article is a new run of my previous benchmark (2020, 2019 and 2018), now running kubernetes 1.26 and ubuntu 22.04 with cni version….

Daily bit(e) of C++ | Optimizing code to run 87x faster
Daily bit(e) of c++ #474, optimizing c++ code to run 87x faster for the one billion row challenge (1brc)..
Geek Culture
SvelteKit Web Worker
How to create and communicate with a web worker in sveltekit, recommended from medium.
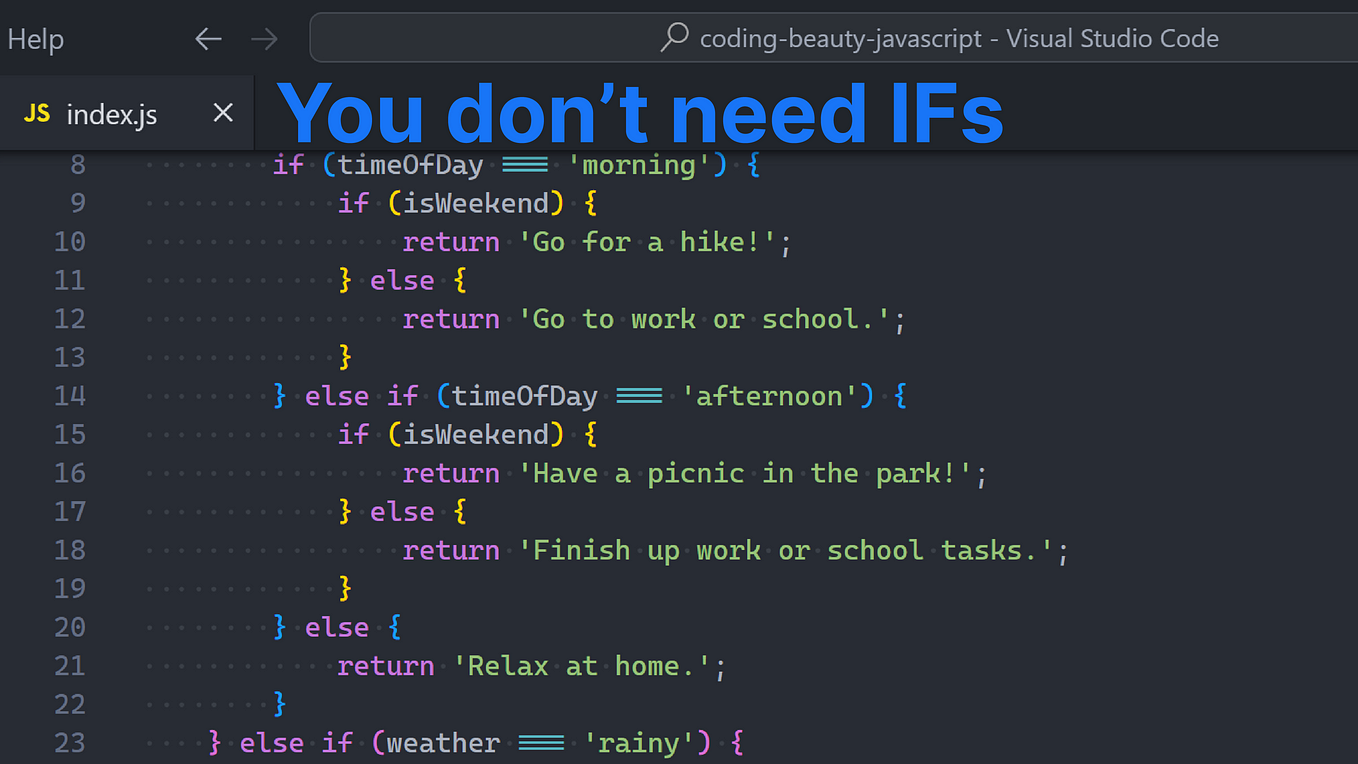
Coding Beauty
You don’t actually NEED if statements (ever)
Powerful💪 if upgrades to completely transform your javascript code.
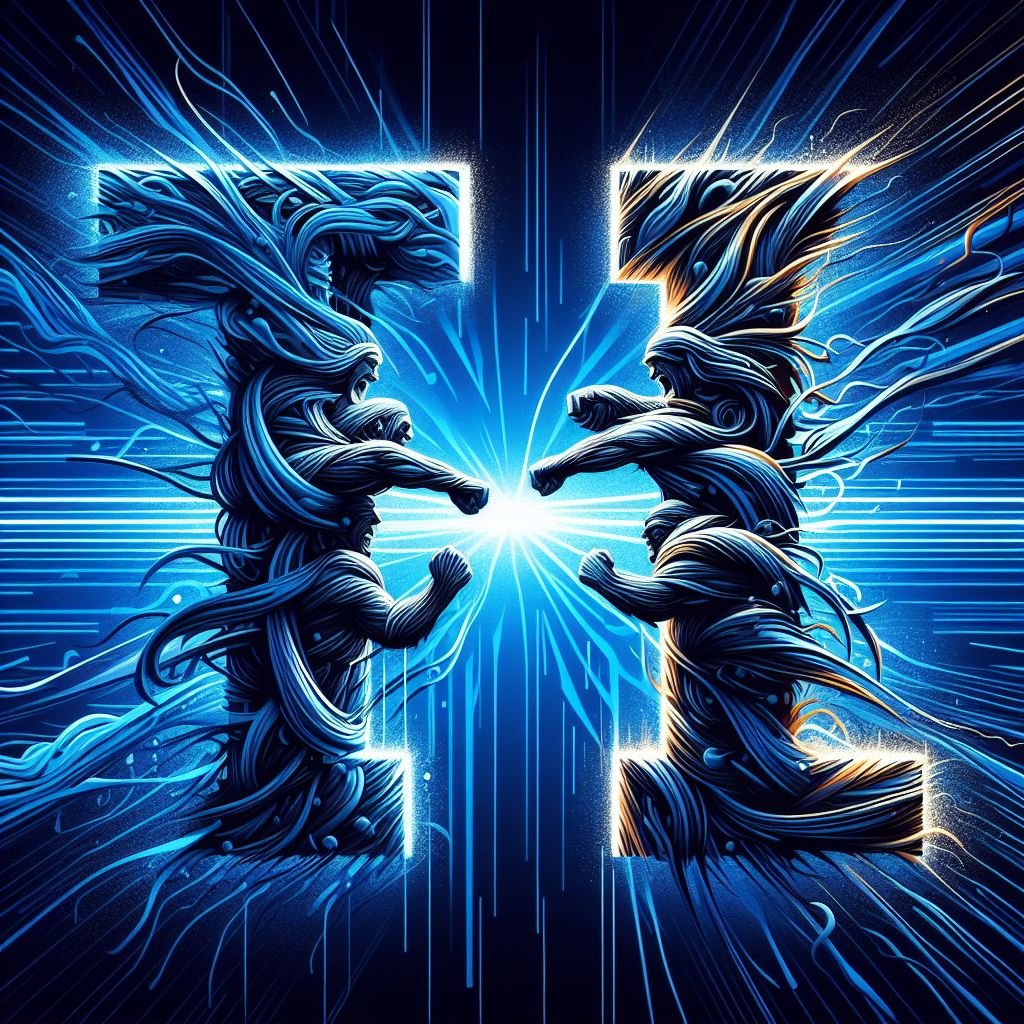
Daniel Craciun
Level Up Coding
Stop Using TypeScript Interfaces
Why you should use types instead.

General Coding Knowledge
Coding & Development

Stories to Help You Grow as a Software Developer

ChatGPT prompts
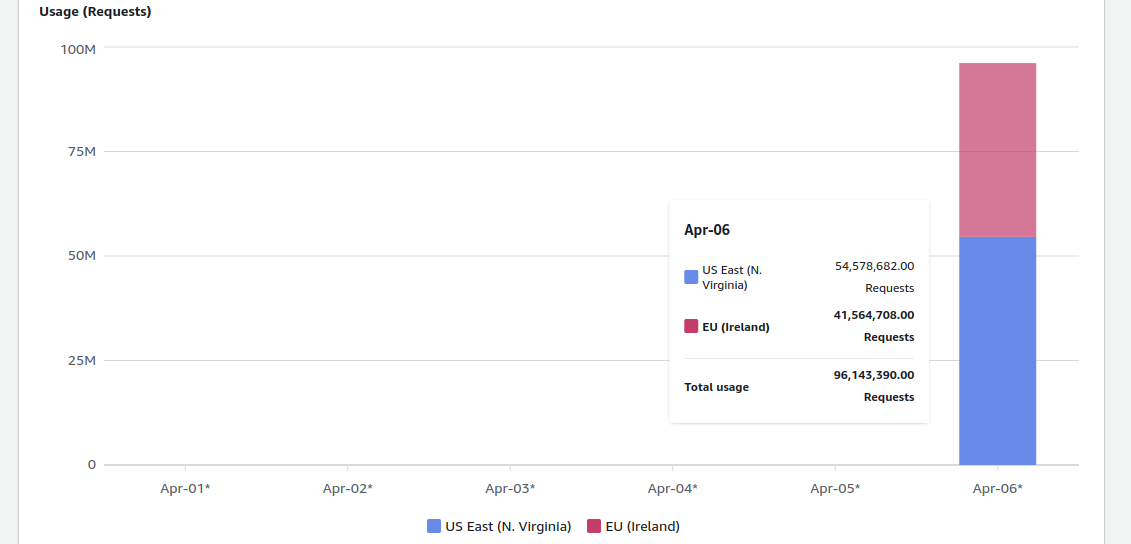
Maciej Pocwierz
How an empty S3 bucket can make your AWS bill explode
Imagine you create an empty, private aws s3 bucket in a region of your preference. what will your aws bill be the next morning.
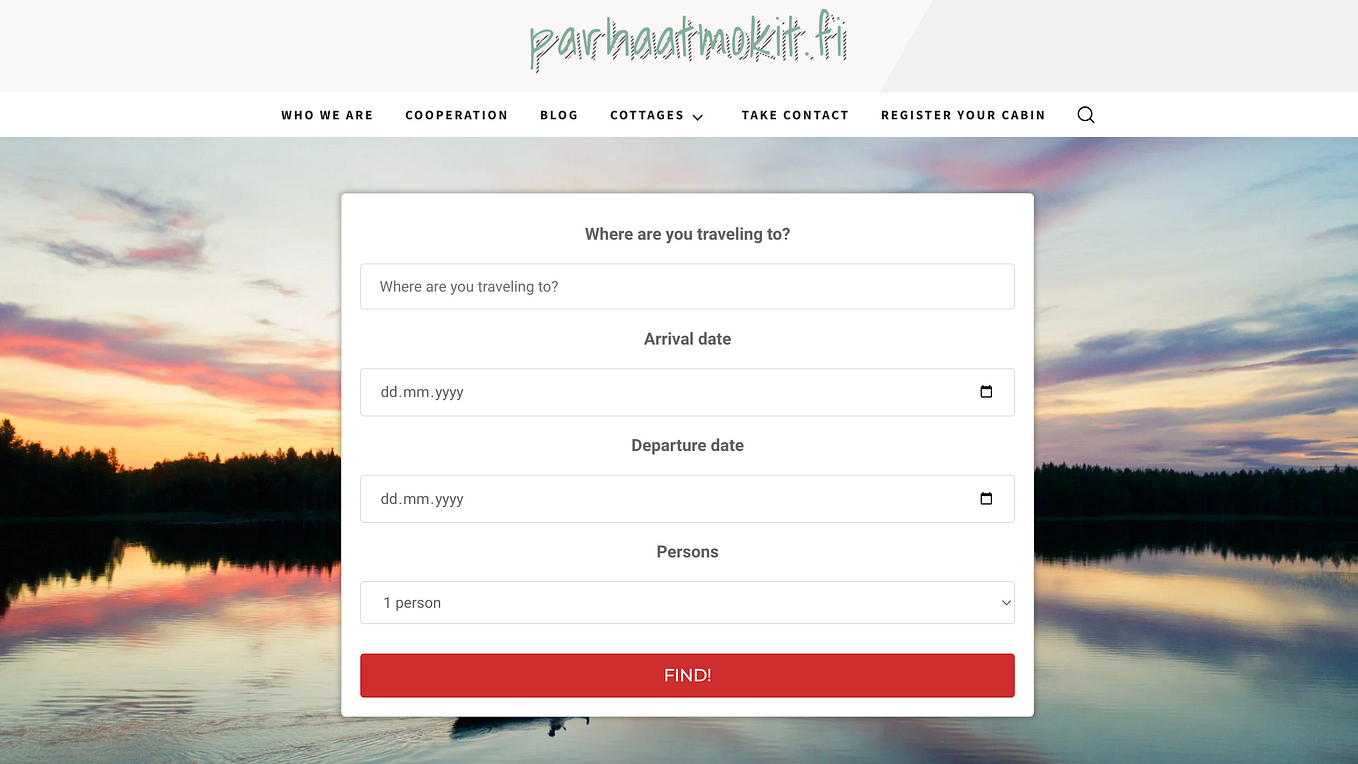
Artturi Jalli
I Built an App in 6 Hours that Makes $1,500/Mo
Copy my strategy.
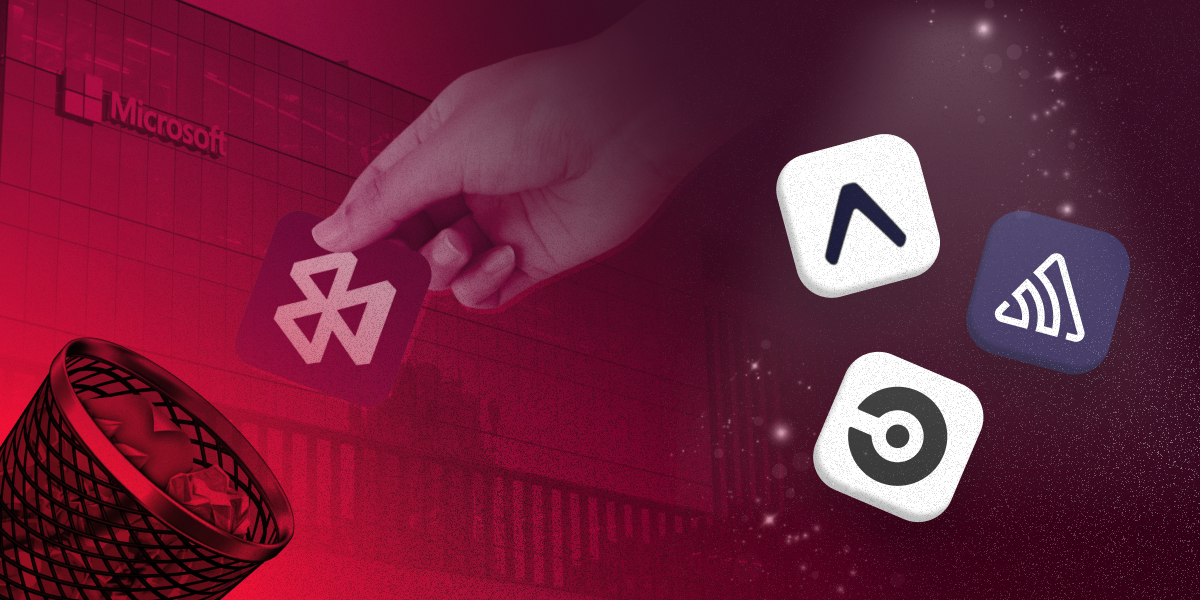
Jamon Holmgren
Microsoft is retiring App Center: Here’s what React Native developers should use instead
Big bummer, react native developers: microsoft announced they are shutting down visual studio app center on march 31, 2025..
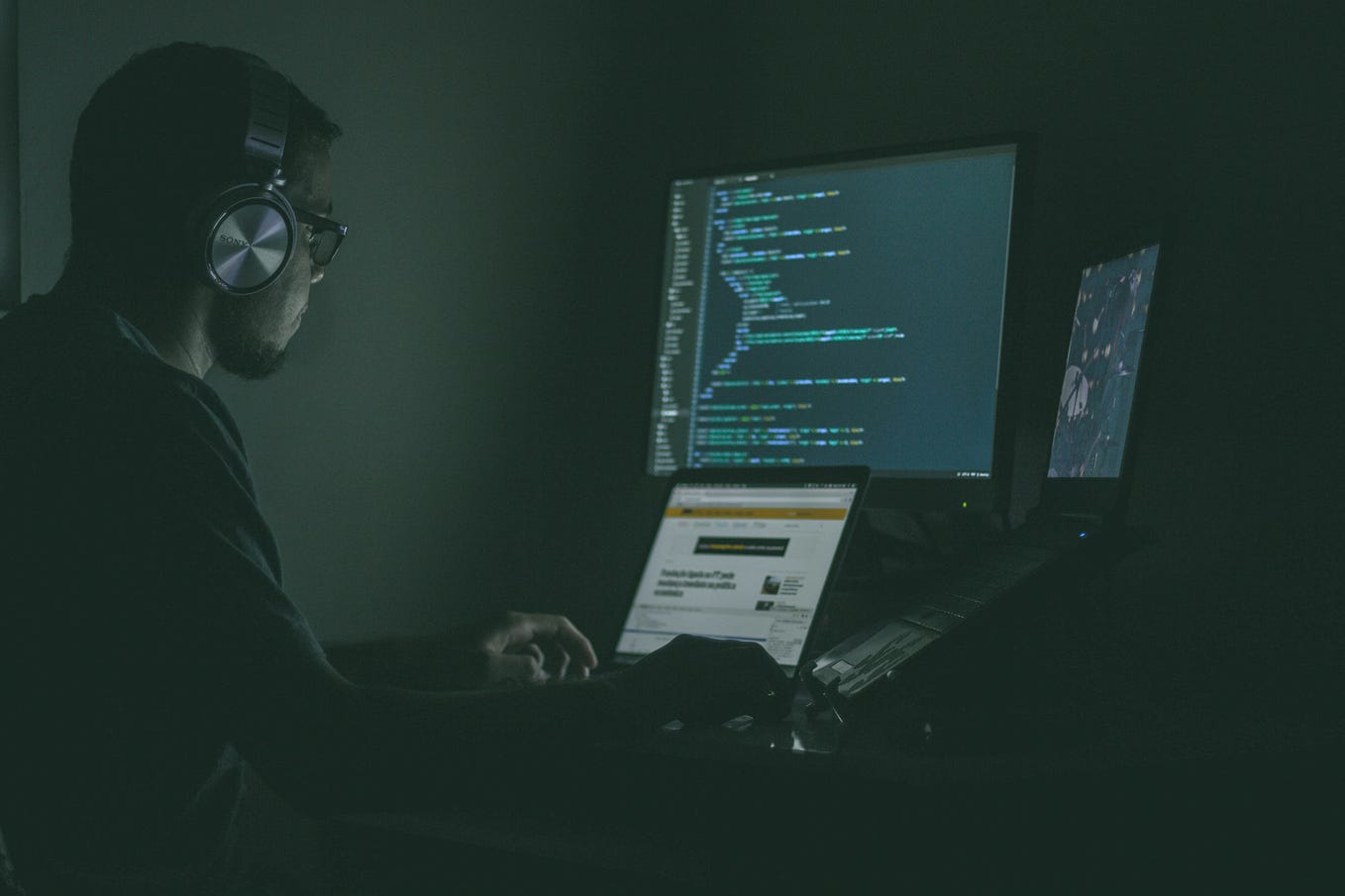
Mate Marschalko
28 JavaScript One-Liners every Senior Developer Needs to Know
Learn how to implement complex logic with beautifully short and efficient next-level javascript syntax..
Text to speech
Check for Mobile iOS Device in JavaScript
September 28, 2021 - 1 minute read
Here’s how you can check whether someone on your website or app is using a mobile iOS device in JavaScript:
A call to the ios() function will return true if the user is using an iPhone, iPad, or iPod. Otherwise, it will return false .
Link to this section Checking for iPhone usage
You could also check for a single device category. The below function will only return true for iPhone users:
Similarly, the iphone() function will return true if the user is using an iPhone, and it will otherwise return false.
Link to this section Conclusion
I’ve tried to make this snippet as precise as possible, but users can still fake their user agent header and so on.
Anyway, I hope you found this useful!
PROWARE tech
Javascript: check for mobile device.
Mobile devices have limited resources so here is a good way to check if a web application is running on a mobile device and then use it to modify the application to react differently based on the platform.
Since iPadOS version 15, the Safari User Agent on the iPad Pro reports as being on the desktop, so always check the navigator.maxTouchPoints to know if it is a tablet or not.
Example usage (click the JS icon to run):
Here is another example that returns more information about the device:
JavaScript: About
The story of JavaScript.
JavaScript: Add Line Numbers to Preformatted Text
Automatically add line numbers to the HTML PRE element.
JavaScript: AJAX Library
A simple AJAX library for FormData and REST API.
JavaScript: AJAX Library for React
A simple AJAX library compatible with React.
JavaScript: AJAX Tutorial
A quick guide to Asynchronous JavaScript And XML (AJAX).
JavaScript: Analog Clock
Scalable analog clock made with HTML, CSS and JavaScript - no images required.
JavaScript: Animate-on-Scroll
Apply a CSS class to an element when it is scrolled into view to cause it to animate.
JavaScript: Auto-hide Text Sections That Are Too Long
Use HTML, CSS and JavaScript to better format a page by hiding large sections of text with a fade to a "read more" link that the user can choose to expand the text with. This is often used on user submitted comments.
JavaScript: Browser Window Utility
Find the true browser window size even if it has a scrollbar displayed.
JavaScript: Browser/Client Detection
Detect the browser and client that the end user is using.
How to determine if the browser is on a mobile device like Apple iPhone, Apple iPod, Google Android or Apple iPad including the iPad Pro with iPadOS version 15.x and 16.x or later.
JavaScript: Classes
Several ways to create classes.
JavaScript: Contact Form Creation Example
How to create a contact form using JavaScript createElement and appendChild procedures.
JavaScript: Cookie Utility
A utility to create, access and delete cookies from the browser.
JavaScript: Copy Text to Clipboard
How to copy text to the clipboard with Chrome, Edge, Firefox and Internet Explorer.
JavaScript: Determine HTML Element Position
Determine where an HTML object is on the screen.
JavaScript: Determine If an Element Has a Class
Determine if an HTML element has a class assigned to it.
JavaScript: Download Images with Progress Indicator Bar
How to download images to the browser while showing the user a percent completion of the download while they wait.
JavaScript: ES5 Array Methods
All the EcmaScript 5 Array Method Functions.
JavaScript: Event Utility
A utility for handling events in the browser.
JavaScript: How to Create WordPress Slugs
Create slugs with only standard characters for use in a URL or other purpose.
JavaScript: Is a Number a Power of Two (2)
How to check or determine if a number has a base of 2 without using modulo operations for maximum performance.
JavaScript: Lazy Load Images
Lazy load images; also works to know if an element has scrolled into view.
JavaScript: LERP - Linear Interpolation Function
A useful function for easing the transition between two values over time (examples included).
JavaScript: Make a Scroll Tracker for a Website
Use JavaScript, CSS and HTML to make a bar move across the screen of a website page to show the user where they are scrolled to.
JavaScript: Parallax Effect
Shift on x-axis and y-axis (horizontal and vertical) parallax effect library.
JavaScript: Parse Internet Links in Plain Text
Use Regex to parse Internet links in plain text and convert to HTML anchor elements.
JavaScript: Position HTML Element
Position a Speech Bubble (DIV Element) with object.getBoundingClientRect().
JavaScript: React without JSX
Learn React without using the large JSX framework.
JavaScript: Remove SCRIPT Elements from HTML
Use regular expressions to remove SCRIPT elements from HTML text.
JavaScript: REST API Tutorial
Understand how the REST API works with examples.
JavaScript: Reveal and Hide Password in HTML Textbox
Show or hide the password in HTML textboxes.
JavaScript: Slide-on-Scroll
Add a sliding effect to the page as it is scrolled.
JavaScript: Stop Developer Tools and the Inspect Element Option
How to disable F12 and Ctrl+Shift+I for developer tools and the inspect element context menu option on all browsers.
JavaScript: Tips and Tricks
Useful tips and tricks for the budding JavaScript programmer.
JavaScript: URI/URL Parser
Parse a URI/URL location; separate the parameters, hashtag; automatically generate arrays for multiple valued parameters.
JavaScript: Use FileReader to Read the Contents of Files
How to use the FileReaader to access file contents in an Internet web browser.
JavaScript: Validate Credit Card Number
Use the Luhn Check to validate credit card numbers.
JavaScript: Validate Email Address
How to validate email addresses by checking their format using client-side code.

JavaScript: Whole Website Example
An idea for a whole website using mostly HTML/CSS with just a little JavaScript.
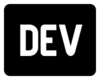
DEV Community

Posted on Jun 30, 2020
A simple way to detect if browser is on a mobile device with Javascript
Sometimes we need a little Javascript code snippet to detect if user use mobile device, the simplest way is detecting its browser user agent.
We use the regular expression test to detect if browser is a mobile device like:
demo is on codepen:
https://codepen.io/timhuang/pen/MWKEZMJ
https://stackoverflow.com/questions/3514784/what-is-the-best-way-to-detect-a-mobile-device
Top comments (33)
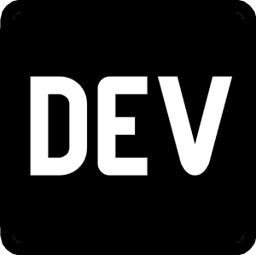
Templates let you quickly answer FAQs or store snippets for re-use.

- Joined Nov 27, 2020
Hey, this is very very unreliable. UserAgent can be changed and as far as I know, iPads want to be treated as desktops now and thus send the same UA in Safari as the Desktop Safari. Mozilla is of a similar opinion: developer.mozilla.org/en-US/docs/W... Hope that helps :-)

- Location Florence, KY
- Work Lifelong Student
- Joined Sep 9, 2020
I didn't read your link, if you provided a solution using that link, my apologies. Otherwise, do you have a better solution? I'm thinking you do ;-)
That link shows that using UserAgent is unreliable. If you trust my comment you don't need to read the source :-)
In one of our projects, we have a workaround that checks for screen size, pixel density, touch and other features only available on mobile devices. It seems to work reliably, but it is an Angular module, that I can't share at the moment. Unfortunately, we don't have a plain JS solution yet. That's also the reason why I did search for a simpler way again (for a non-angular project) and found your solution. At first, I was amazed, but then I realized that we have tried this approach in the past and it was not reliable enough for what we needed. So I just wanted to leave this comment here, so people know about it. For this project, I'm back to simple breakpoints considering the width of the screen.

- Joined Feb 26, 2021
"Browser identification based on detecting the user agent string is unreliable and is not recommended, as the user agent string is user configurable."
If we require that users don't modify their UserAgent in order to use our website then the UserAgent is perfectly reliable!

- Location Mexico
- Work Software producto manager
- Joined Apr 7, 2021
I agree with @bechtold , using the user agent is a bad idea, there are multiple features to consider, I recommend this article on how to use media queries in JavaScript, I think it is a bester practice.
"using the user agent is a bad idea, there are multiple features to consider"
Would you elaborate on why using the user agent is a bad idea? (Also, I'm not sure if you meant features or some other word.)

- Location Florianópolis - SC / Brazil
- Work Newbie Web Developer at Freelancer
- Joined Jan 4, 2020
@jeancarlo13 the problem with using media queries in JS is that it causes layout shift (FOUC) and it's a problem mainly on above-the-fold UI. Otherwise it's a good approach.

- Location Dubai
- Work Mr at mfc
- Joined Aug 28, 2019
You can use JavaScript window.matchMedia() method to detect a mobile device based on the CSS media query.
if (window.matchMedia("(max-width: 767px)").matches) { // The viewport is less than 768 pixels wide document.write("This is a mobile device."); }
You may also use navigator.userAgentData.mobile .
const isMobile = navigator.userAgentData.mobile;
Another approach would be a responsive media query. You could presume that a mobile phone has a screen size greater than x and less than y. For example:
@media only screen and (min-width: 320px) and (max-width: 600px) {}
net-informations.com/js/iq/default...

- Joined Sep 6, 2023
Unfortunately navigator.userAgentData.mobile appears to be not widely supported yet
caniuse.com/?search=userAgentData caniuse.com/?search=mobile

- Location Taipei, TW
- Work Developer at Deep Blue
- Joined Jun 11, 2020
Thanks for sharing!

- Location GLASGOW
- Work Self employed at Glasgow
- Joined Dec 30, 2019
Thank you for this, I have ended up placing it in a view class as a getter function
Honestly thanks for this eloquent regex test.
thanks for your improvement! :)

- Location Zürich
- Joined Feb 4, 2020
Make it shorter like this:

- Location Kyiv, Ukraine
- Joined Jun 10, 2020
Wow, really short! Thanks for your improvement.

- Location Kazakhstan, Almaty
- Joined Nov 24, 2021
Alternatively, you can use
navigator.mediaDevices.enumerateDevices().then(md => { console.log(md) }); and use field
MediaDeviceInfo.kind Read only Returns an enumerated value that is either "videoinput", "audioinput" or "audiooutput".
MediaDeviceInfo.groupId Read only Returns a DOMString that is a group identifier. Two devices have the same group identifier if they belong to the same physical device — for example a monitor with both a built-in camera and a microphone.
That is, if several "videoinput" and their groupId are the same, most likely this is a mobile device, since there are more than one laptop and monitor with two cameras and more.
That function suported all desktop and mobile browsers except IE.
Great! An alternative solution. Thanks!

- Joined May 23, 2020
'ontouchstart' in window => true for mobile devices => false for desktop environment

- Location London, UK & Madrid, Spain
- Work Front-end web engineer at ING Bank
- Joined Sep 17, 2019
Some desktops have touchscreen devices, this is not reliable
I think you've taken the question too literally. The main issue behind the question is for developers to distinguish whether their JavaScript code needs to handle touch events vs click events, and not to figure out if someone can literally lug their device around in a mobile manner. Determining if touch events are in the window object is a simple way to determine this. Cheers!

- Joined Aug 31, 2023
I think you've taken the question too literally. The main issue behind the question is for developers to distinguish whether their JavaScript code needs to handle touch events vs click events
No, this is very often not true.
In my case, I'm interested in this right now because I need to default the UI rendering of a webpage to relate better to our mobile app when the user is on mobile.
If the user lands on a marketing page, for example, we want to frontload the marketing copy that talks about the mobile features of our product suite. That's not relevant to someone running Windows on a touchscreen laptop.
User Agent detection is not a recommended technique for modern web apps. There is a JavaScript API built-in for detecting media. The JavaScript window.matchMedia() method returns a MediaQueryList object representing the results of the specified CSS media query string. You can use this method to detect a mobile device based on the CSS media query.
$(document).ready(function(){ let isMobileDevice = window.matchMedia("only screen and (max-width: 760px)").matches; if(isMobileDevice){ // The viewport is less than 768 pixels wide //Conditional script here } else{ //The viewport is greater than 700 pixels wide alert("This is not a mobile device."); } });
You can use above script to do show/hide elements depending on the screen size.
net-informations.com/js/progs/mobi...

- Location Bangladesh
- Work Junior Software Developer
- Joined Oct 21, 2020
This is working fine! Thank you.
Great! I hope this could help who need to detect if browser on a mobile device. Thanks for your visiting.

- Joined May 1, 2022
it's better to use 'navigator.userAgentData.mobile' because it only returns true or false

- Joined May 18, 2022
unfortunately this property is not supported all browsers developer.mozilla.org/en-US/docs/W...

- Education Yes 9 classes and college full.
- Work Backend nodejs and php
- Joined Apr 3, 2019
an interesting solution for new browsers, and most importantly fast: stackoverflow.com/questions/783868... ?
a pity that display media-queries are not available for very ancient devices and browsers (hello Safari)
for server side detect device in production, i use packet npmjs.com/package/node-device-dete... (I'm the author, maybe I'm PR late)
I have made some improvements to the speed of detecting devices and clients, if you also use the library, I recommend updating the dependency to the latest version.
Great! Thank you!

- Joined Apr 11, 2021
It worked very well for me. Thanks, @timhuang I adapted the modification proposed by @drgaud

- Joined Jan 3, 2021
hello, thank man, it helped me a lot. working like a charm.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

Kickstart your development with Bun
AgentQuack - Apr 4
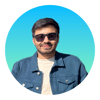
A Powerful Password Generator NPM Package
abhilaksh-arora - Apr 7
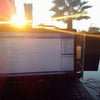
Embark on a UI Odyssey: 5 Spectacular Libraries to Explore
Bojan Jagetic - Apr 16

How to install the latest LTS version of "NodeJS" in "LINUX"?
Roshan Khetpal - Apr 7
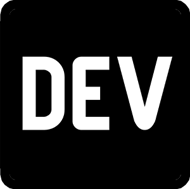
We're a place where coders share, stay up-to-date and grow their careers.
- Main Content
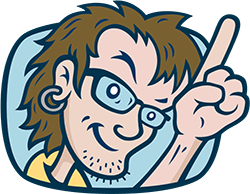
- JavaScript Promises
- ES6 Features
iPad Detection Using JavaScript or PHP
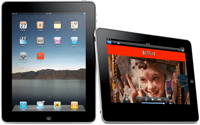
The hottest device out there right now seems to be the iPad. iPad this, iPad that, iPod your mom. I'm underwhelmed with the device but that doesn't mean I shouldn't try to account for such devices on the websites I create. In Apple's developer tip sheet they provide the iPad's user agent string:
Given that string we can create a few code snippets to determine if the user is being a smug, iPad-using bastard.
The JavaScript
A quick String.match regular expression test can check for the presence of "iPad" in the user agent string.
This time we look for the position of "iPad" in the user agent string.
The .htaccess
Using some logic from Drew Douglass' excellent mobile redirection post , we can redirect users to a mobile version of your website if you so desire.
So what would you the above tests for? You may want to redirect iPad users to a different version of your website. You may want to implement different styles to your standard website if your user is surfing on an iPad.
Recent Features

Create a Sheen Logo Effect with CSS
I was inspired when I first saw Addy Osmani's original ShineTime blog post . The hover sheen effect is simple but awesome. When I started my blog redesign, I really wanted to use a sheen effect with my logo. Using two HTML elements and...
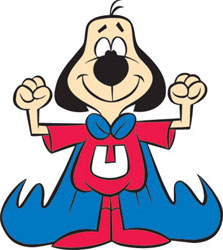
Conquering Impostor Syndrome
Two years ago I documented my struggles with Imposter Syndrome and the response was immense. I received messages of support and commiseration from new web developers, veteran engineers, and even persons of all experience levels in other professions. I've even caught myself reading the post...
Incredible Demos
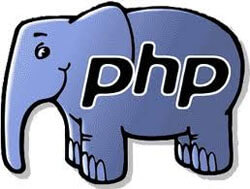
PHP Woot Checker – Tech, Wine, and Shirt Woot
If you haven't heard of Woot.com, you've been living under a rock. For those who have been under the proverbial rock, here's the plot: Every day, Woot sells one product. Once the item is sold out, no more items are available for purchase. You don't know how many...
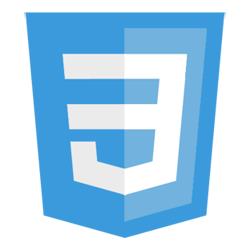
Using CSS attr and content for Tooltips
I've found myself in love with CSS content and attr ; I've recently written about how you can use the property and expression on a basic level , how you can implement CSS counters , and use for the sake of localization . I wanted to share...
Nice tutorial. Did you get an iPad? I also created a similar tutorial http://cardonadesigns.com/wordpress/2010/04/04/using-php-to-detect-the-ipad-user-agent/
I just wrote a similar post, and referred your post.
As I described the technique in the post, it’s better to apply different CSS to iPad visitors rather than redirecting them to a special version of your website (when it meets your requirements). This way all you need to do is manage different CSS files.
http://jquery-howto.blogspot.com/2010/10/javascript-to-detect-ipad-visitors.html
I respectfully disagree. Especially if your site has flash, more than a one column layout, or really bandwidth intensive content.
Standard web and mobile web are completely different beasts. Simple css tweaks may not be enough.
I applaud the idea of keeping maintenance easy but I think anyone who is even thinking of doing a mobile site, will have to prioritize which content to show.
The cruddy thing about the iPad user agent string is that it has the word “mobile” in it. So if your detection script is looking for “mobile” already, it may also be sending iPad users to your iphone/mobile optimized site. Apple offered up a way to test though -> http://developer.apple.com/safari/library/technotes/tn2010/tn2262.html
Yes but you could employ logic in both cases which does and does not find the string “iPad”.
Great! Now I can redirect/block iPad users!
@Rob: That’s what I was thinking! Thanks David.
I just use this code:
What if I need a different landing site for the ipad, I try this and it doesn´t work
if((navigator.userAgent.match(/iPhone/i)) || (navigator.userAgent.match(/iPod/i))) { location.replace(“iphone/index.html”); } if((navigator.userAgent.match(/iPad/i))) { location.replace(“ipad/index.html”); } else { location.replace(“index_frames.html”); }
Maybe try location.href I’ve never used location.replace.
@Connor Crosby: that is in a javascript
Another, and often better option is media queries in CSS3
http://www.w3.org/TR/css3-mediaqueries/
You can deliver CSS based on device characteristics, such as viewport width, and orientation (so you can have different CSS for portrait and landscape)
http://developer.apple.com/safari/library/documentation/AppleApplications/Reference/SafariWebContent/OptimizingforSafarioniPhone/OptimizingforSafarioniPhone.html
Let them eat Flash
Welcome to the old web !
“Site optimised for IE 5.5”
But where is the code to make the iPad melt once detected? :p
@Noetic: you could start with js “while……..” =x
Very nice tutorial, tnx man!
I was a little stuck. I’m looking to do something similar, but really just target the viewport for the iPhone/iPod Touch and iPad. Here’s what I came up with so far but doesn’t seem to work. Any suggestions?
Easy to do redirecting in PHP:
If you just want to redirect iPhone users:
If you also want to bounce iPad users, use this instead:
Here’s what the session variable “fullsite” is for:
I always use a link in the mobile site that would allow the user to go to the full site if he wishes; the link goes to a page I call “fullsite.php,” and which includes this code:
This sets the session variable and then sends them back to the full site’s home page, and prevents the PHP from bouncing them continually back into the mobile site.
Note that the session variable will stay set until they close their browser.
Hey ! Thanks for the turorial ! I’m a newbie, and I wanna do something I guess is easy, but can’t find a solution. What I wanna do is to display a certain element (a div) if the user is on his computer, but display another one instead if he is on his iPad.
What should I write, and where ????
Please help me !
This post is featured on 40 iPad tools, tips for designer
Perfect! thank you David!
Hi! Thanks for the article.
I wrote a free open source set of APIs for web designers to detect mobile visitors. The APIs are very light weight, easy to implement, and easily customized. They’re available for JavaScript, PHP, Java, and ASP.NET. Check out http://www.mobileesp.org
Nice article, thanks.
Also nice site and article on iPad site dev Garmahis. Particularly enjoyed the link to page flipping versus scrolling. :)
Thank you very much for this page! I probably owe you a cut of the small fee I earned last night solving someone’s emergency iPod detection crisis. And you’re funny! (lots of people can code. Funny, not so much.)
Did you know that its not possible to copypaste you hillarious quotes when using An ipad to reading you site?
“determine if the user is being a smug, iPad-using bastard” Genius!
Hate the bastards myself. It’s the iPhone all over again: “You didn’t invent it, you bought it, anyone can buy a bloody iPad”
Sorry for posting again in such quick succession, but I’ve just found a very natty PHP class that handles all browser user agents, including the iPad. Thought you might be interested: http://www.phpclasses.org/package/6369-PHP-Detect-the-type-of-browser-accessing-the-site.html Promise I’m not spamming!
Nice Article. The regexp works great for single device detections. If you’re after detecting device classes like Tablets or other mobile browsers check out http://www.handsetdetection.com (disclaimer : I work there).
Cheers Richard
Awesome tutorial! Saved me for iPad in my use
Nice way to check, but I am waiting to buy iPad.
thank you for info
As of iOS 3.2 (which is iPad-only), the user agent string no longer contains ‘iPad’. Rather, the device identifies itself as ‘iPhone’, so the above solution does not work. The way I’m detecting iPads in JavaScript–at least until I come across a better way–is with the following expression:
/iPad/i.test(ua) || /iPhone OS 3_1_2/i.test(ua) || /iPhone OS 3_2_2/i.test(ua)
Sorry, the ‘ua’ in that line of code should be navigator.userAgent
When is/was iOS 3.2 released? I just tested on my iPad, which has its iOS up to date, and the user agent included “iPad”.
http://davidwalsh.name/dw-content/detect-ipad-new.php
I’m seeing iPad in the UA for Safari on iPad as well. I think the case I was seeing was an embedded UIWebView inside an iPad app. Most web developers won’t have to worry about that case, I suppose, but it is pretty vital for native app developers using web views.
Awesome, thanks Andrew. I updated my article. Cheers!
Laughed my ass off on the ‘iPad this, iPad that, iPad your mom’. hahaha! Made my day!!!
To those having issues like “too many redirections” there’s a solution, here’s the link of StackOverflow where this guy helped me figure out a easy solution to this!
http://stackoverflow.com/questions/5131158/ipad-iphone-browser-sniffer-with-mod-rewrite-and-redirection-too-many-redirecti
Hey, becareful with this line:
PHP’s strpos() can return 0 , meaning that the $needle is at position 0 (zero) of the string. So if you do like that, $isiPad can return FALSE where the correct value is TRUE .
To resolve that, do this:
That is true in general, but in the case of the user-agent string for iPad it never puts iPad at the start of the string…
Palomita para tí, awesome tutorial, simply! efficient! Thanks a lot.
Thanks for the code snippets David…! Thanks for Andrew too.
Surely there must be a better way than using userAgent… At the end of March 2011, there are literally HUNDREDS of possible values for the userAgent, all of which have to be manually mapped as “true” or “false” as to whether they are mobile devices. In the coming months and years, that number could easily become THOUSANDS!!! This is a regression to web-dev practices of the 1990s. As developers, we cannot rely on such tactics… Simply including such a file means users have to download SEVERAL THOUSAND ADDITIONAL BYTES just to determine if they are mobile or not. Maybe we should be identifying screen resolutions or something “simpler”…
@Blunt Brother – someone already commented on this – see CSS3 media queries. I agree with you by the way – user agent sniffing everything is a big regression. We’d just about, almost FINALLY got Microsoft to fix IE and the desktop browser space is better than it’s ever been for web developers, so now everyone wants to bend over for mobile devices? Insane.
How about for ipad 2?
Awesome post that I was looking for. Although a suggestion : the code with black background is hard to read i had to copy it and read it .
Thanks again
Anyone here had experience detecting iPad then providing a specific version of a video within the page to only thos ipad users, allowing for stack overflow to take care of other safari, ff, and ie users. The reason i need to do this is to overcome ipad 2’s gamma problem when playing video. I need to provide a gamma adjusted version of the video just to ipad users (preferably just ipad2 but i dont think the user agent string is that specific?,
Ideally just JS, and Im no JS wizard.
Any help would be greatly appreciated.
Just wanted to share this, since it pertains to the topic.
Our hero image once clicked opens a pop-up/shadowbox image viewer (imaging provided by Adobe Scene 7) on our company website, but (non-Flash-i)Pads don’t dig it. We use Miva Merchant as a part of our backend. The following code, using Miva Script, allows us to detect iPads and reveal a compatible viewer alternately:
-------- MOBILE USER AGENT --------
-------- MOBILE S7 VIEWER --------
-------- MOBILE ENLARGE VIEWS BUTTON --------
-------- NORMAL USER AGENT --------
-------- PRODUCT IMAGE --------
-------- ENLARGE VIEWS BUTTON --------
Essentially, if the site detects a mobile device, show the alternative viewer. Else, reveal the normal one.
Thanks for the insight thus far.
A fourth method are ESI tags like:
http://esi-examples.akamai.com/viewsource/ad.html
This is required when using a server side templating system that renders first before JavaScript
the php code is not working anymore.. :( help anyone?
This post is missing what’s by far the best check for an iPad (or iPhone) in JavaScript:
navigator.platform === 'iPad' || navigator.platform === 'iPhone'
The best part is that the platform property can’t be easily spoofed. Some Android devices, for example, will allow users to change the user agent string to match that of an iPad, but changing it won’t affect the value of navigator.platform.
You can do it in PHP:
http://sjevsejev.blogspot.com/2012/05/php-mobile-detect-class-ipad-iphone.html
I advise you check out http://wurfl.io/
In a nutshell, if you import a tiny JS file:
you will be left with a JSON object that looks like:
(that’s assuming you are using a Nexus 7, of course) and you will be able to do things like:
if(WURFL.form_factor == “Tablet”){ //dostuff(); }
The service also goes out of its way to detect specific iPad models.
I know this is an old post but it turns up near the top of a google search for php detecting ipad or something like that. Anyway I just wanted to note that your casting the result of strpos to a boolean is a bad idea. This is because strpos can return a 0 position which simply means the string you are looking for is at the beginning of the string you are searching. When you then cast this to a boolean you get false, implying that the string didn’t contain the search term when in fact it did. The best practice use of strpos to check for the presence of a search term would in fact be
This works because a treble character equality (or inequality) test checks both the type and the value.
navigator.userAgent.match(/iPad/i) not work with Ipad Pro
Since iOS 13 the iPad sends out the exact same User Agent string as desktop Safari, so one has to use hacky client-side solutions to determine if it’s an iPad – the old solutions just apply until and including iOS 12!
Wrap your code in <pre class="{language}"></pre> tags, link to a GitHub gist, JSFiddle fiddle, or CodePen pen to embed!
How to Enable JavaScript on Apple Safari (iPad, iPhone iOS)
Are you having a hard time in trying to turn on JavaScript on your iPad or Apple iPhone device?
JavaScript has evolved into an essential tool that makes the web what it is today. It controls the dynamic elements of web pages, and most websites will fail to run correctly if your Apple Safari browser does not have its JavaScript enabled. Although disabling JavaScript offers browsers faster loading of a website, you should know that it reduces the overall browsing experience on your iPad or iPhone device.
Be it an iPhone 11, iPhone 10 or MacOS device, the steps in this guide will help you learn the simple process and benefits of enabling the JavaScript feature on your Safari browser.
Instructions for Web Developers
You may want to consider linking to this site, to educate any script-disabled users on how to enable JavaScript in six most commonly used browsers. You are free to use the code below and modify it according to your needs.
On enablejavascript.io we optimize the script-disabled user experience as much as we can:
- The instructions for your browser are put at the top of the page
- All the images are inlined, full-size, for easy perusing
We want your visitors to have JavaScript enabled just as much as you do!
What Is JavaScript and Why Do I Need It?
JavaScript is a type of code used by web developers to install and establish interactive content on websites – in essence, it allows web pages to dynamically load and send content in the background without page loads on your Apple Safari browser. Generally, JavaScript is used by modern websites to provide various features such as display advertisements – the reason why a small subset of internet users want it disabled.
However, disabling the JavaScript feature on your Safari web browser is much more of a hassle than it seems. If you turn off JavaScript on your browser, many websites won’t function properly. In most cases, you will also be unable to enjoy certain functions or view content that you are accustomed to accessing on JavaScript-based websites.
How to Enable JavaScript on Safari
Here’s how to turn on JavaScript on Safari:
1. Select the “Safari” icon on your home screen.
2. Scroll down the Settings page to find the menu item labelled “Safari”, and then select it.
3. Scroll to the bottom of the Safari menu and choose “Advanced” – choosing this will reveal the advanced settings for your Safari browser.
4. On the Advanced menu, find the option for enabling or disabling “JavaScript” for your Safari browser. On finding the JavaScript option, you’ll see a button next to it.
5. Now slide this button to the right to turn on JavaScript on your Safari browser.
6. That’s it! You’ve just enabled JavaScript.
How to Disable JavaScript on Safari
Here’s how to turn off JavaScript on Safari:
1. Tap on the “Settings” icon on your home screen.
5. Now slide this button to the left to turn off JavaScript on your Safari browser.
6. That’s it! You’ve just disabled JavaScript.
How to Enable JavaScript on Mac
Follow the steps below to activate JavaScript in Safari on Mac:
1. Open the Safari application by clicking on an icon that appears like a compass in your bottom toolbar.
2. Click on the “Safari” menu at the top left corner of the browser.
3. Under the Safari menu, find and click on “Preferences”. This will open a pop-up menu.
4. Move your cursor over to the “Security” tab and select it.
5. Now check the box beside “Enable JavaScript” to turn on JavaScript. Make sure the box is checked.
6. You can now close the “Preferences” window to apply your settings.
7. That’s it! You’ve enabled JavaScript.
8. Restart your browser.
How to Disable JavaScript in Safari on Mac
Follow the steps below to disable JavaScript in Safari on Mac:
2. Click on “Safari” menu at the top left corner of the browser.
3. Under the Safari menu, find and click on “Preferences”. This will open a dropdown menu.
4. Move your cursor over to the “Security” tab and then click on it.
5. Now uncheck the box beside “Enable JavaScript” to disable JavaScript.
7. That’s it! You’ve disabled JavaScript.
8. Restart your browser.
Apple Safari comes built-in with a JavaScript engine that makes website elements interactive. And while it isn't actually necessary that you enable it to use your Safari browser, it's something that you'll perhaps want to do to enjoy a seamless browsing experience. Otherwise, many websites you visit will appear broken or won't even work.
JavaScript is enabled by default in Apple Safari, but you can verify if yours is active through the Safari tab. To do this, simply click on "Safari" at the top left of your screen to expand the Menu. Next, click on Preferences to reveal the Safari Preferences section. Now that you're in the Preferences section, find and click the "Security" tab to access the "Enable JavaScript" checkbox. If the checkbox has a tick symbol, it means JavaScript is active and working.
Millions of websites use JavaScript to display interactive elements, such as animations, special effects and more. If you browse them with JavaScript disabled in your Apple Safari, then you probably won't have the full experience that you normally would. Some JavaScript-based websites may appear dull or static, while others may not even work at all.
Olumide is a longtime writer who started his career as a digital marketer before transitioning into a copywriter almost eight years ago.
- – Google Chrome
- – Internet Explorer
- – Microsoft Edge
- – Mozilla Firefox
- – Apple Safari
How to enable JavaScript in your browser and why http://www.enablejavascript.io/
Test your JavaScript, CSS, HTML or CoffeeScript online with JSFiddle code editor.
Online javascript editor, testing javascript online, online ide, online code editor, html, css, coffeescript, scss online editor.
- Vote for features
Editor layout
Console in the editor (beta)
Clear console on run
Line numbers
Indent with tabs
Code hinting (autocomplete) (beta)
Auto-run code
Only auto-run code that validates
Auto-save code (bumps the version)
Auto-close HTML tags
Auto-close brackets
Live code validation
Highlight matching tags
Planned features by votes
- Publish fiddles as mini-websites
- Save fiddles as templates
- Download a zipped fiddle
Join the 4+ million users, and keep the JSFiddle dream alive.
All ads in the editor and listing pages are turned completely off.
Use pre-released features
You get to try and use features (like the Palette Color Generator) months before everyone else.
Fiddle groups
Sort and categorize your Fiddles into multiple groups.
Private groups and fiddles
You can make as many Private Fiddles, and Private Groups as you wish!
Debug your Fiddle with a minimal built-in JavaScript console.
Save anonymous (public) fiddle?
- Be sure not to include personal data - Do not include copyrighted material
Log in if you'd like to delete this fiddle in the future.
Fork anonymous (public) fiddle?
Embed snippet Prefer iframe? :
No autoresizing to fit the code
Render blocking of the parent page
Fiddle meta
http://stackoverflow.com/q/4460205/918414
Private fiddle PRO
Resources URL cdnjs 0
- Paste a direct CSS/JS URL
- Type a library name to fetch from CDNJS
Async requests
/echo simulates Async calls: JSON: /echo/json/ JSONP: //jsfiddle.net/echo/jsonp/ HTML: /echo/html/ XML: /echo/xml/
See docs for more info.
Other (links, license)
Created and maintained by Piotr and Oskar .
Hosted on DigitalOcean
All code belongs to the poster and no license is enforced. JSFiddle or its authors are not responsible or liable for any loss or damage of any kind during the usage of provided code.
Bug tracker About Docs Service status
Support the development of JSFiddle and get extra features ✌🏻
Lifestyle, adventure and backstage photographer based in warsaw, poland..
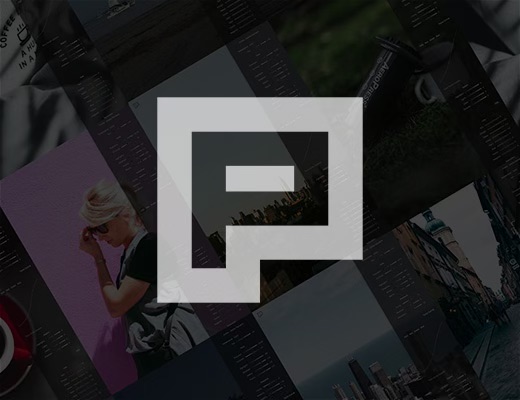
Exif Data Viewer: turn JPG files into Lightroom presets.
Frameworks & extensions, framework <script> attribute.
Normalized CSS
Keep JSFiddle running. Whitelist us in your content blocker.
We've been providing web developers, educators, students, companies with JSFiddle free for many years .
Your can help us in one of two ways:
- Whitelist JSFiddle in your content blocker
- Go PRO and get access to additional PRO features →
How to enable JavaScript on your iPad to make websites and videos work properly in Safari
- You should enable JavaScript on your iPad so that websites you access function properly.
- If JavaScript is not enabled in your browsers, some websites won't function or will function poorly, and features like animations and videos won't work, so it is important that you know how to turn it on.
- Visit Business Insider's homepage for more stories .
Most websites are programmed using JavaScript , which is essential for more advanced or complex operations and functions.
JavaScript can also be used to program, and there are a lot of resources for learning to use JavaScript for amateur programmers up to high-end web developers.
If JavaScript is not enabled in a browser, your iPad will inhibit functionality on some sites and prevent you from even accessing others.
That can also limit your ability to access certain features of websites. Some programs and applications from the web, which use JavaScript as well, will also have trouble working.
Check out the products mentioned in this article:
Ipad (64gb), available at best buy, from $799.99, how to enable javascript on your ipad.
1. Tap the "Settings" app.
2. Scroll down until you see "Safari," or any other web browser that you'd like to enable JavaScript in.
3. Tap on the "Safari" icon.
4. Scroll down and tap "Advanced," at the very bottom.
5. JavaScript should be one of the few items you see. Tap on the "JavaScript" toggle so that it turns green.
6. JavaScript is now enabled in Safari.
7. If you have other browsers you use, you will have to go back, select those in "Settings" and repeat the process for them as well.
Related coverage from How To Do Everything: Tech :
How to enable javascript in safari on a mac computer to prevent website errors, how to enable javascript on your iphone if it's disabled, to access most websites in a safari browser, how to delete an email account on your ipad in 4 simple steps, how to turn off autocorrect on your ipad in 4 simple steps.
Insider Inc. receives a commission when you buy through our links.
Watch: A professional race car driver turned the luxurious Porsche 911 into a rugged off-road sports car
- Main content
How To Enable Javascript On IPad Safari

- Software & Applications
- Browsers & Extensions
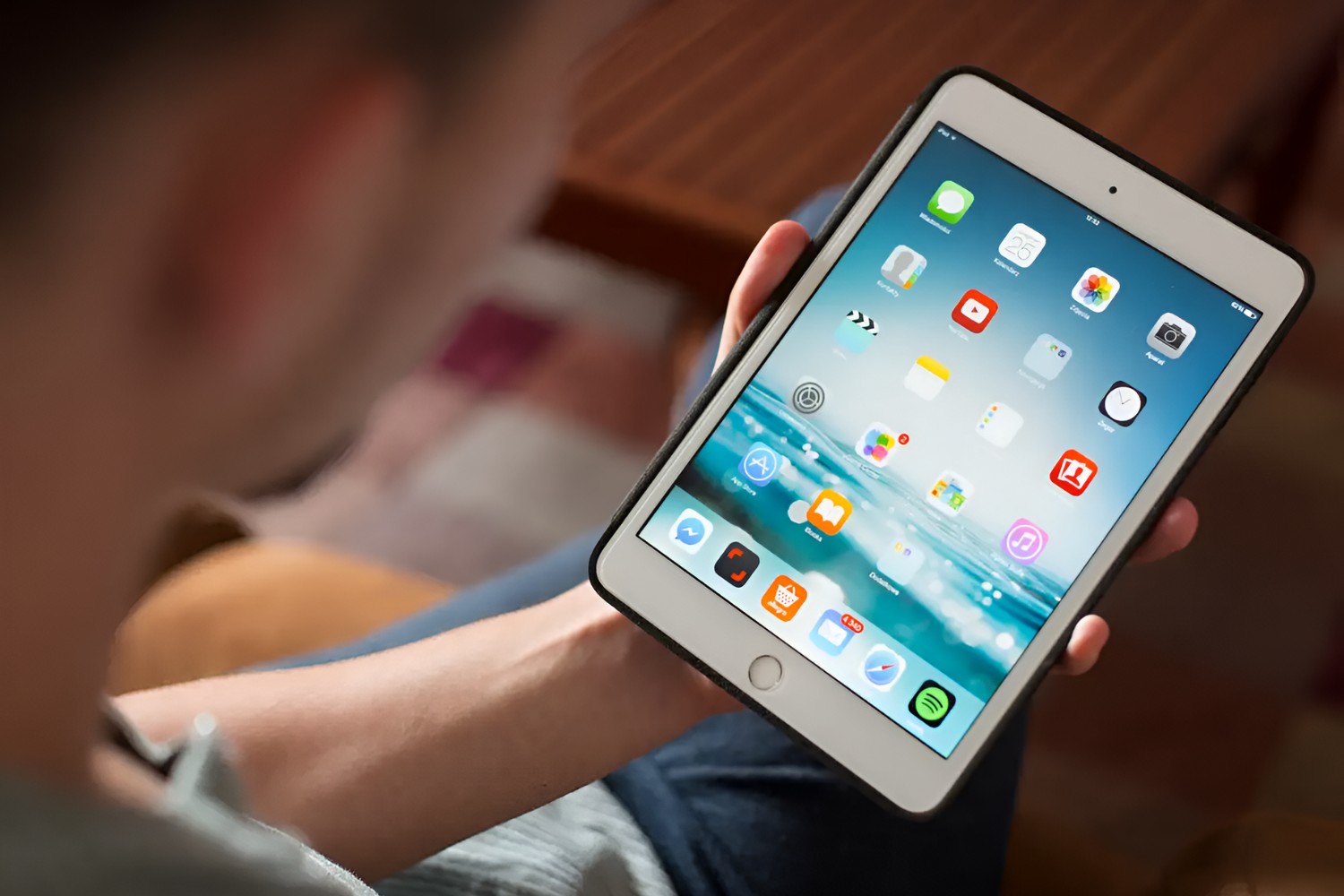
Introduction
Enabling JavaScript on your iPad's Safari browser can significantly enhance your browsing experience by allowing interactive and dynamic content to function seamlessly. JavaScript is a fundamental component of modern web browsing, enabling websites to deliver engaging features such as interactive forms, dynamic page updates, and responsive design elements. By enabling JavaScript on your iPad's Safari browser, you can ensure that you have access to the full range of features and functionality that websites offer, providing a more immersive and interactive online experience.
In this guide, you will learn how to enable JavaScript on your iPad's Safari browser in a few simple steps. Whether you're accessing educational resources, engaging with interactive web applications, or simply browsing the web for entertainment, having JavaScript enabled is essential for accessing the full range of features and content that websites have to offer.
By following the steps outlined in this guide, you will be able to seamlessly enable JavaScript on your iPad's Safari browser, empowering you to make the most of your browsing experience. Let's dive into the straightforward process of enabling JavaScript and ensure that you can enjoy the rich and interactive content available on the web.
Step 1: Open Safari Settings
To begin the process of enabling JavaScript on your iPad's Safari browser, you first need to access the Safari settings. This initial step is crucial as it provides you with access to the browser's configuration options, allowing you to customize various settings to suit your browsing preferences.
Launch the Settings App : Start by locating the Settings app on your iPad's home screen. The Settings app is represented by a gear icon and is an essential tool for customizing various aspects of your device's functionality.
Scroll and Locate Safari : Within the Settings app, scroll down the left-hand menu until you find the option for Safari. This will typically be represented by a compass icon, denoting the browser settings.
Tap on Safari : Once you have located the Safari option, tap on it to access the specific settings for the Safari browser.
By following these simple steps, you can easily access the Safari settings on your iPad, setting the stage for the subsequent steps in enabling JavaScript. With the Safari settings now accessible, you are ready to proceed to the next step and enable JavaScript to unlock the full potential of your browsing experience.
This initial step lays the foundation for customizing your Safari browser to align with your preferences and requirements. By accessing the Safari settings, you gain the ability to tailor the browsing experience to suit your individual needs, ensuring that you can make the most of the features and functionality available within the Safari browser.
With the Safari settings now within reach, you are well-positioned to move on to the next step and enable JavaScript, paving the way for a more interactive and dynamic browsing experience on your iPad.
Step 2: Enable JavaScript
Now that you have accessed the Safari settings on your iPad, the next crucial step is to enable JavaScript. JavaScript is a fundamental scripting language that enables dynamic and interactive content to function seamlessly within web pages. By enabling JavaScript in your Safari browser, you ensure that websites can deliver engaging features, interactive forms, dynamic page updates, and responsive design elements, providing you with a richer and more immersive browsing experience.
To enable JavaScript on your iPad's Safari browser, follow these simple steps:
Toggle JavaScript Setting : Within the Safari settings, locate the "JavaScript" option. This setting allows you to control whether JavaScript is enabled or disabled in the Safari browser.
Enable JavaScript : Tap on the toggle switch next to the "JavaScript" option to enable JavaScript. When the toggle switch is in the "on" position, JavaScript is enabled, allowing websites to utilize its full range of interactive and dynamic features.
By following these straightforward steps, you can seamlessly enable JavaScript in your Safari browser, unlocking the full potential of web content and ensuring that you have access to the interactive elements that modern websites offer.
Enabling JavaScript empowers you to engage with a wide range of online content, from interactive forms and multimedia elements to dynamic page updates and responsive design features. With JavaScript enabled, you can fully immerse yourself in the interactive experiences that websites provide, enhancing your browsing journey on your iPad.
By taking this essential step to enable JavaScript, you are poised to make the most of the dynamic and interactive content available on the web, ensuring that your browsing experience is both engaging and seamless. With JavaScript now enabled in your Safari browser, you are ready to verify that it is functioning as intended, confirming that you can fully engage with the interactive elements that websites have to offer.
Step 3: Verify JavaScript is Enabled
After enabling JavaScript in your Safari browser, it's essential to verify that it is functioning as intended. This verification step ensures that JavaScript is indeed enabled and ready to empower you with the full range of interactive and dynamic features that websites offer. By confirming that JavaScript is enabled, you can proceed with confidence, knowing that you are poised to engage with the rich and immersive content available on the web.
To verify that JavaScript is enabled on your iPad's Safari browser, follow these simple steps:
Navigate to a JavaScript-Dependent Website : Open Safari and visit a website known for its interactive and dynamic content. This could be a site with interactive forms, multimedia elements, or dynamic page updates. By visiting such a website, you can test whether JavaScript is functioning as expected.
Interact with Website Features : Once you have accessed a JavaScript-dependent website, interact with its various features. This could involve filling out interactive forms, engaging with multimedia content, or navigating through dynamically updated page elements. By interacting with the website's features, you can confirm that JavaScript is indeed enabled and facilitating the interactive elements of the site.
Observe Responsive Design Elements : Pay attention to the responsiveness of the website's design elements. JavaScript plays a crucial role in enabling responsive design, allowing web pages to adapt to different screen sizes and orientations. By observing how the website's design elements respond to your interactions and device orientation, you can further verify that JavaScript is functioning optimally.
By following these steps, you can effectively verify that JavaScript is enabled and facilitating the interactive and dynamic features of the websites you visit. This verification process ensures that you can fully engage with the rich and immersive content available on the web, confident in the knowledge that JavaScript is empowering your browsing experience.
With JavaScript successfully verified as enabled, you can now enjoy a seamless and interactive browsing experience on your iPad's Safari browser. Whether you're accessing educational resources, engaging with interactive web applications, or simply exploring the diverse content available online, JavaScript's enabling ensures that you can make the most of the dynamic and interactive elements that modern websites offer.
In conclusion, enabling JavaScript on your iPad's Safari browser is a pivotal step in unlocking the full potential of your browsing experience. By following the simple and straightforward process outlined in this guide, you have empowered your Safari browser to seamlessly interact with the dynamic and interactive content that modern websites offer.
With JavaScript enabled, you can now engage with a diverse range of online content, from interactive forms and multimedia elements to dynamic page updates and responsive design features. This enhancement ensures that your browsing journey on your iPad is not only engaging but also seamless, allowing you to fully immerse yourself in the interactive experiences that websites provide.
The ability to enable JavaScript on your Safari browser opens the door to a myriad of possibilities. Whether you are accessing educational resources, engaging with interactive web applications, or simply exploring the diverse content available online, JavaScript's enabling ensures that you can make the most of the dynamic and interactive elements that modern websites offer.
Furthermore, by verifying that JavaScript is functioning as intended, you have confirmed that your Safari browser is fully equipped to deliver a rich and immersive browsing experience. The verification process ensures that you can confidently interact with the interactive and dynamic features of the websites you visit, knowing that JavaScript is facilitating a seamless and responsive browsing experience.
In essence, the process of enabling and verifying JavaScript on your iPad's Safari browser empowers you to embrace the full spectrum of web content, ensuring that you can engage with the interactive elements that websites have to offer. This fundamental enhancement elevates your browsing experience, allowing you to explore, learn, and interact with online content in a more immersive and dynamic manner.
By enabling JavaScript, you have positioned yourself to fully embrace the interactive and dynamic nature of the modern web, ensuring that your browsing experience on your iPad's Safari browser is both enriching and seamless. With JavaScript now enabled and verified, you are ready to embark on a captivating and interactive journey through the diverse landscape of online content.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Crowdfunding
- Cryptocurrency
- Digital Banking
- Digital Payments
- Investments
- Console Gaming
- Mobile Gaming
- VR/AR Gaming
- Gadget Usage
- Gaming Tips
- Online Safety
- Software Tutorials
- Tech Setup & Troubleshooting
- Buyer’s Guides
- Comparative Analysis
- Gadget Reviews
- Service Reviews
- Software Reviews
- Mobile Devices
- PCs & Laptops
- Smart Home Gadgets
- Content Creation Tools
- Digital Photography
- Video & Music Streaming
- Online Security
- Online Services
- Web Hosting
- WiFi & Ethernet
- Browsers & Extensions
- Communication Platforms
- Operating Systems
- Productivity Tools
- AI & Machine Learning
- Cybersecurity
- Emerging Tech
- IoT & Smart Devices
- Virtual & Augmented Reality
- Latest News
- AI Developments
- Fintech Updates
- Gaming News
- New Product Launches

Learn To Convert Scanned Documents Into Editable Text With OCR
Top mini split air conditioner for summer, related post, comfortable and luxurious family life | zero gravity massage chair, when are the halo awards 2024, what is the best halo hair extension, 5 best elegoo mars 3d printer for 2024, 11 amazing flashforge 3d printer creator pro for 2024, 5 amazing formlabs form 2 3d printer for 2024, related posts.
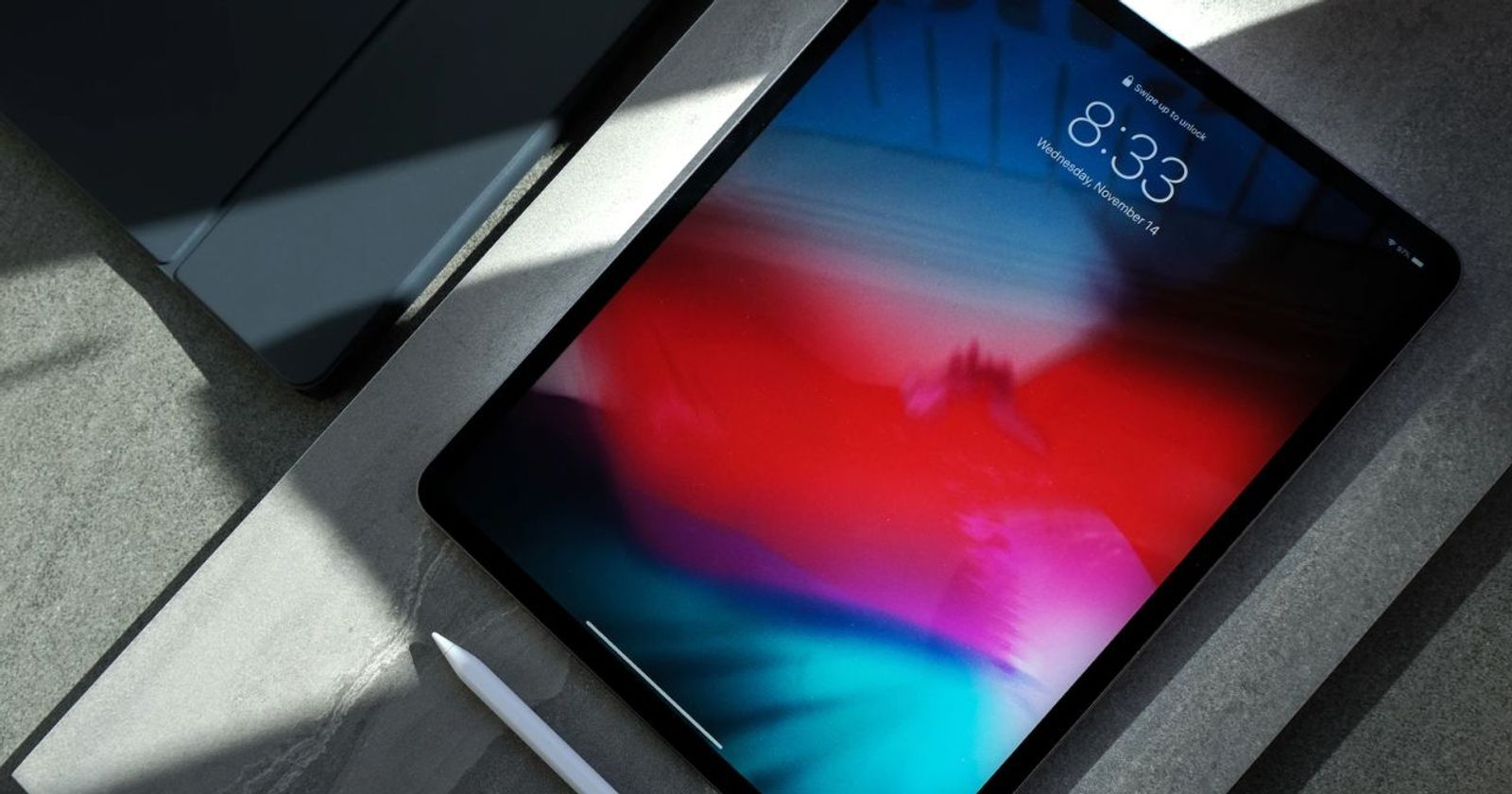
How To Inspect In Safari On IPad
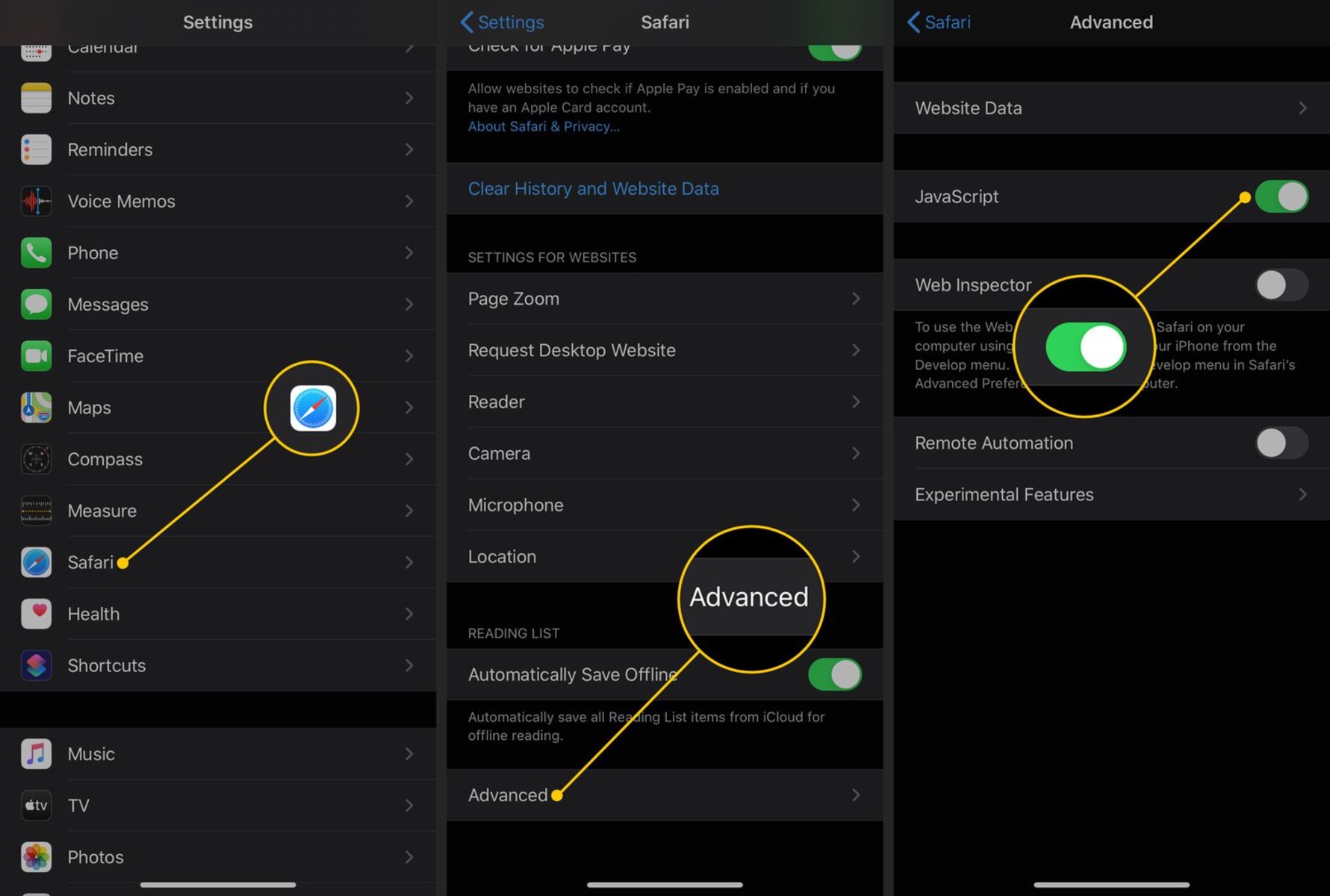
How To Disable Javascript On Safari
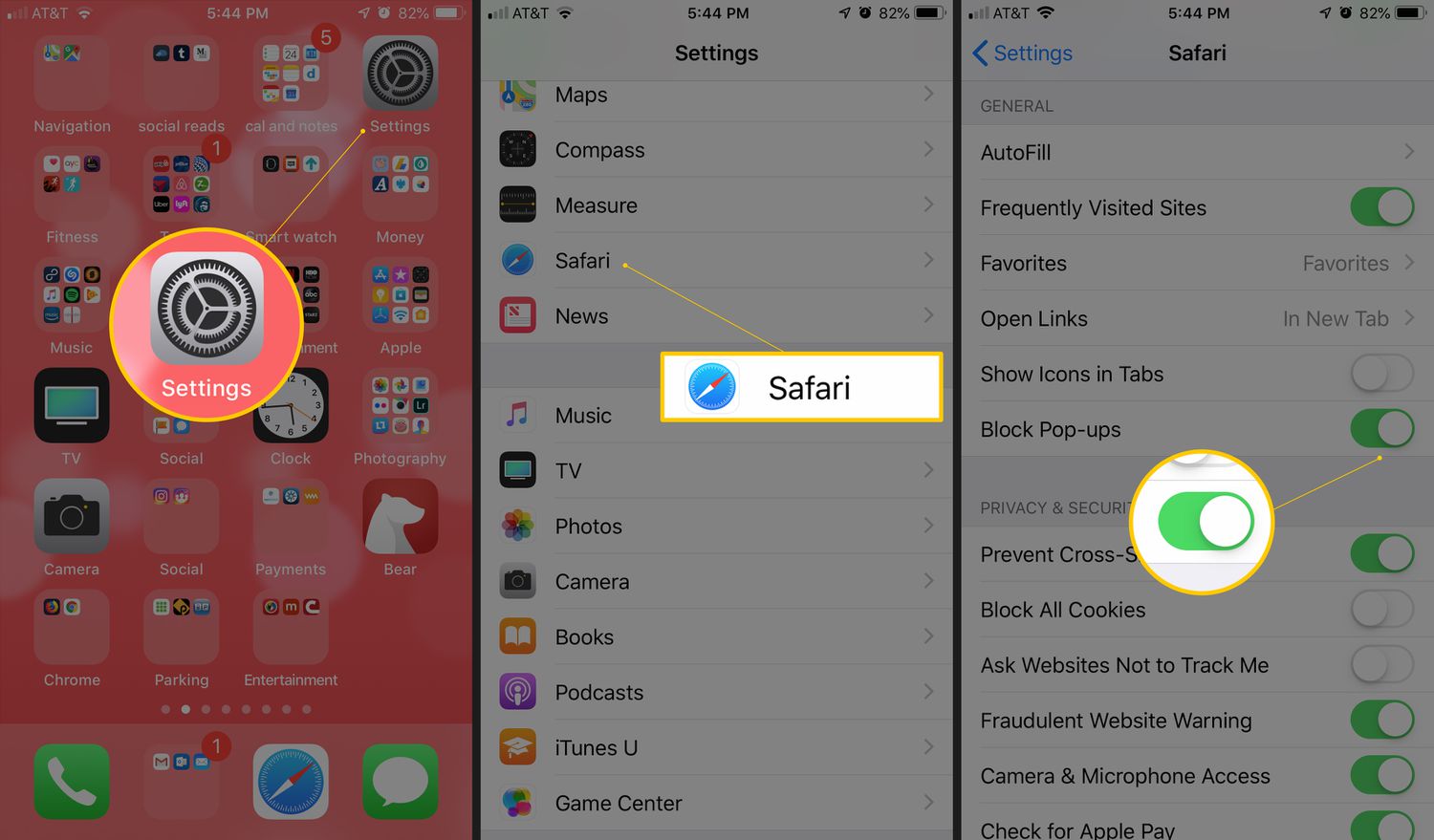
How To Stop Pop-Ups On IPad Safari
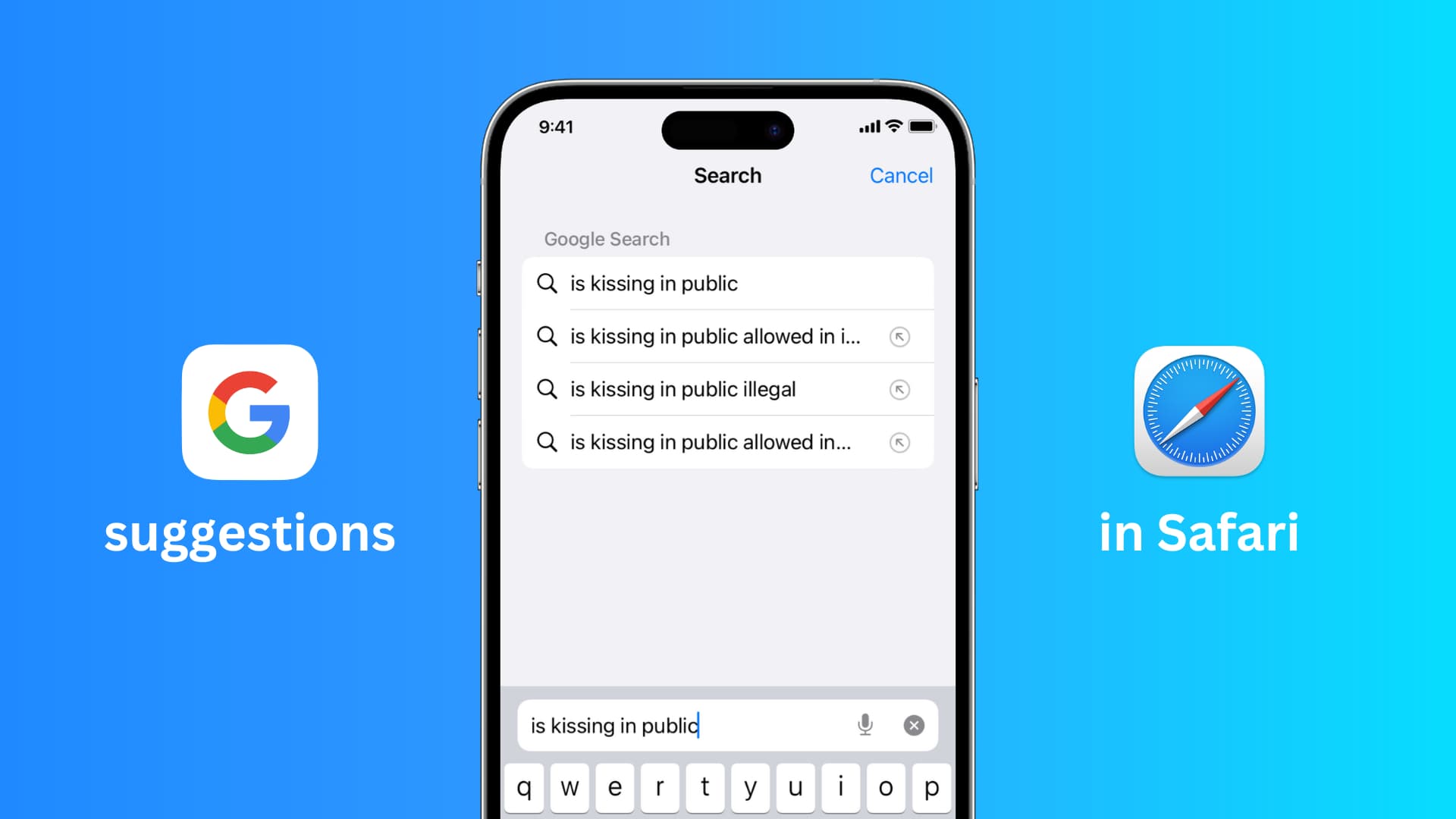
How To Keep Google Signed In On Safari
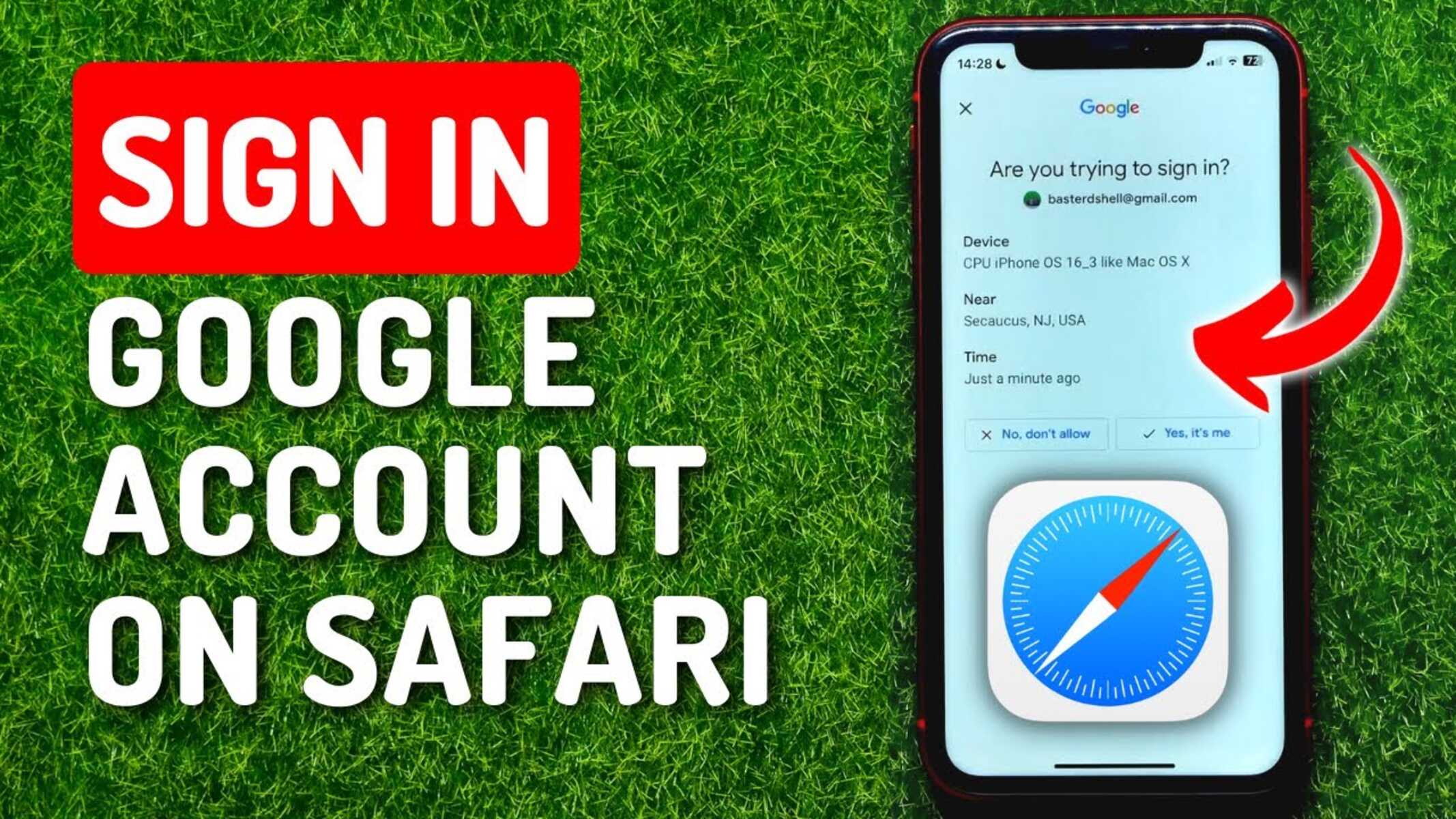
How To Stay Signed In To Google On Safari
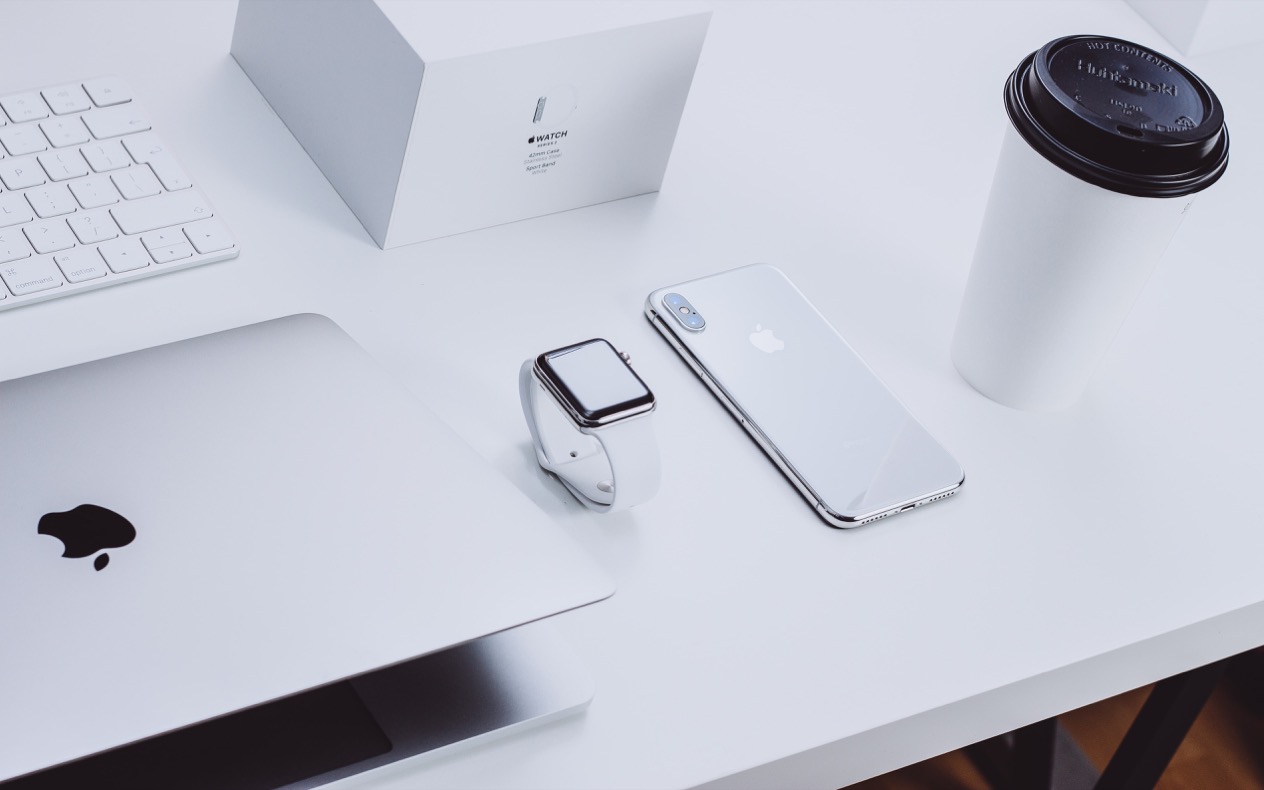
How To Open Developer Tools In Safari
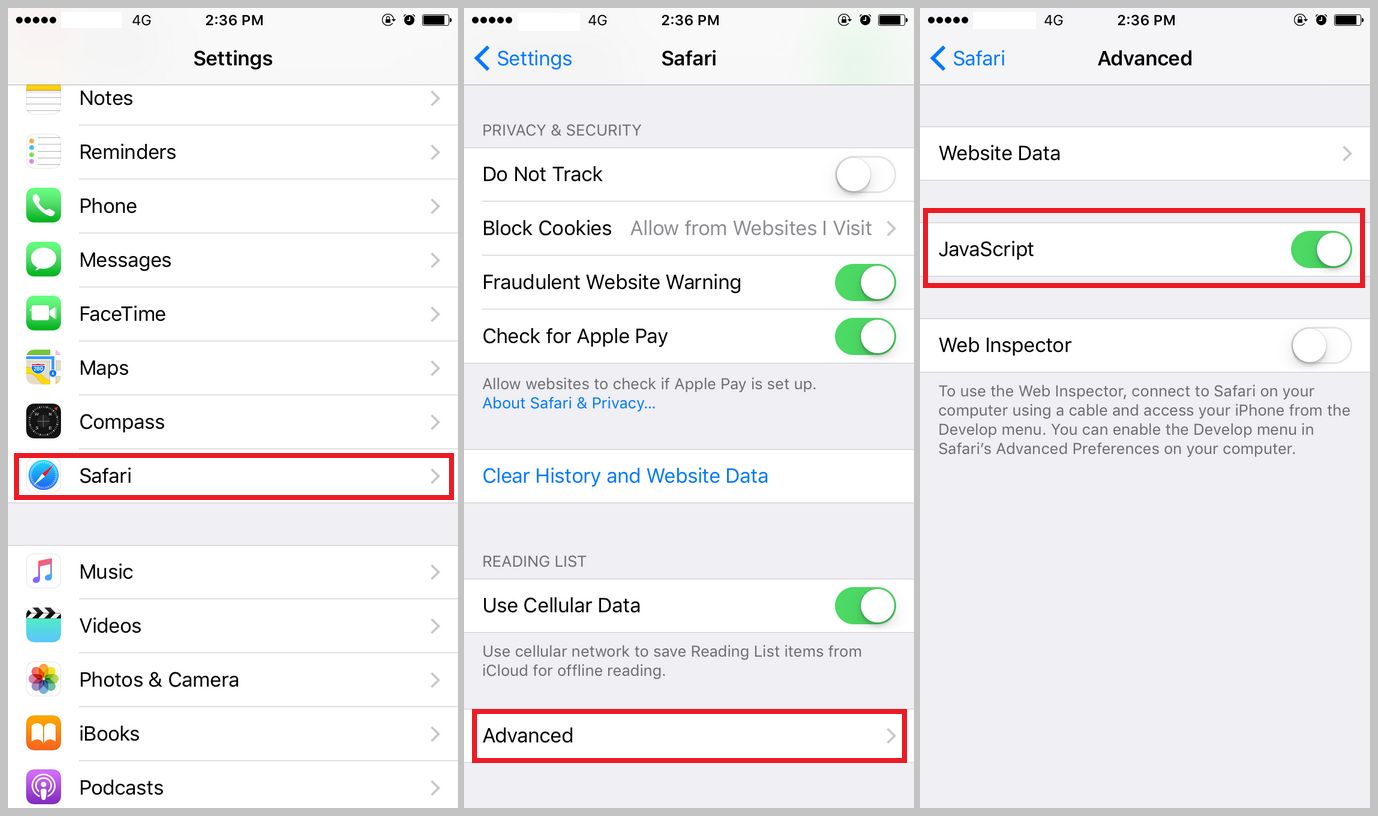
How To Enable Javascript For Safari
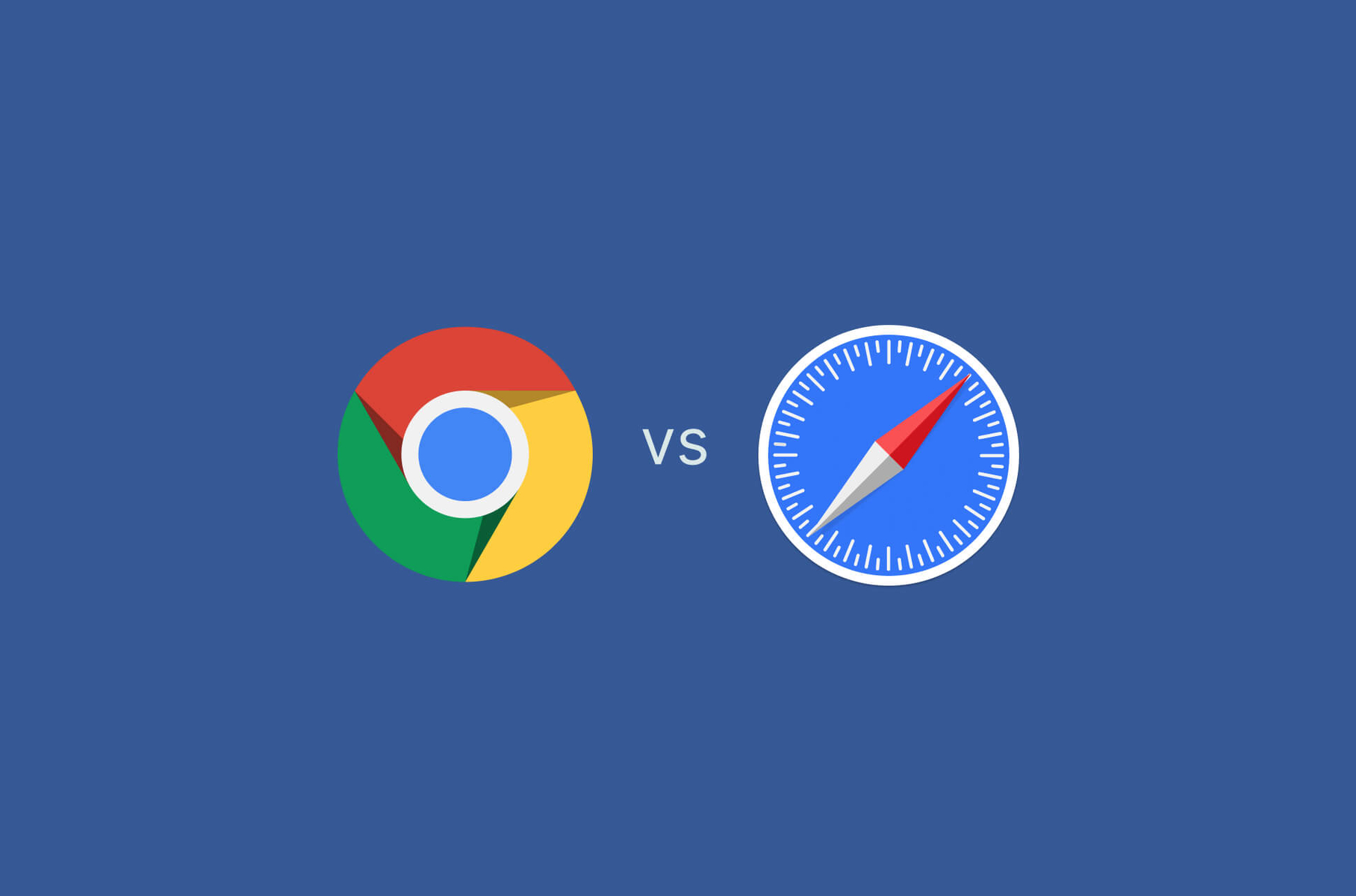
How Good Is The Safari Browser
Recent stories.
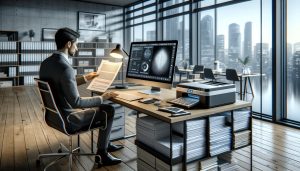
Fintechs and Traditional Banks: Navigating the Future of Financial Services
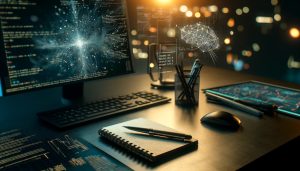
AI Writing: How It’s Changing the Way We Create Content
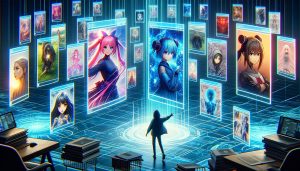
How to Find the Best Midjourney Alternative in 2024: A Guide to AI Anime Generators
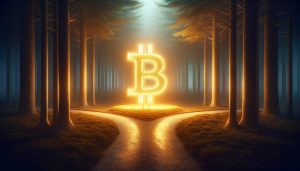
How to Know When it’s the Right Time to Buy Bitcoin
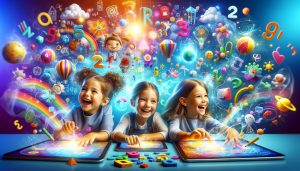
Unleashing Young Geniuses: How Lingokids Makes Learning a Blast!
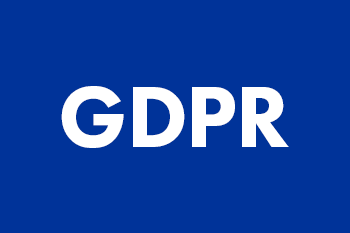
- Privacy Overview
- Strictly Necessary Cookies
This website uses cookies so that we can provide you with the best user experience possible. Cookie information is stored in your browser and performs functions such as recognising you when you return to our website and helping our team to understand which sections of the website you find most interesting and useful.
Strictly Necessary Cookie should be enabled at all times so that we can save your preferences for cookie settings.
If you disable this cookie, we will not be able to save your preferences. This means that every time you visit this website you will need to enable or disable cookies again.

iPhone user agent - Use JavaScript to detect the user agent
iPhone 'user agent' FAQ: I'm trying to optimize my HTML/web app for the iPhone; how do I detect an iPhone, iPod, or iPad browser in my web application?
This Apple page shows the iPhone, iPod, and iPad user agent strings, so you just need to write some JavaScript that properly parses those iPhone/iPad browser user agent strings.
Here's what the iPhone user agent string looks like for iOS 2:
That's actually one long string, but I wrapped it here to reduce scrolling.
As you can see, the string "iPhone" is contained in that user agent text, so a simple way of searching for the iPhone string in the user agent text with JavaScript looks like this:
That statement makes the variable isIphone true if the string "iPhone" is seen in the browser user agent text, or false if the text is not found.
Detecting iPhone, iPod, or iPad user agents
You can use just that one line if you're really only concerned about detecting the iPhone in your HTML application, or you can also perform other checks for the iPod and iPad to detect all iOS devices:
Now that you've properly detected the iPhone, iPod, or iPad devices in your web application using JavaScript, you can use the isIos variable (or the other JavaScript variables we created) in your JavaScript decision-making process.
iPhone/iPad user agent detecting - More information
For more information on detecting iPhone and iPad user agents, see this Apple documentation page . For a JavaScript library which was made for detecting Apple WebKit devices, see this link on detecting WebKit .
iPhone/iPad web app user agent detecting - Summary
I hope this tip on using JavaScript to detect iPod, iPhone, and iPad devices in your HTML/web apps has been helpful. When you're trying to optimize your web app to look as much as possible like a native iPhone app, you just need little tips like this to optimize the HTML (and JavaScript) in your web app.
Help keep this website running!
- iPhone and iPad JavaScript dialogs (alert, confirm, prompt)
- iPhone HTML apps - Status bar control (color control)
- iPhone Safari debugging console (iPhone/iPad web app debugging)
- Setting an iPhone HTML/web app icon | iPhone app home screen icon
- iPhone HTML apps - Using an app startup image (app splash screen)
books by alvin
- ZIO ZLayer: A simple “Hello, world” example (dependency injection, services)
- A dream vacation for the meditator in your life
- Al's Oasis
- Window of the Poet (painting)
- Shinzen Young on meditating in his daily life: arising, disappearing, and The Source, and love

Detect Ipad with javascript
How to detect if the device is iPad with javascript in the latest version the userAgent looks like this…
Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/16.0 Safari/605.1.15
I would suggest to use CSS for the themes/templates instead of using the jQuery but here it is
Thank you but I need to know if the device is ipad…
Can I ask why do you need to target iPad devices?
I am working on a Javascript project and I there is a bug specific only on Ipad and I have to write special code for it…

@FWDesign - what happens if people have disabled Javascript?
These links may help:
If you go on Google Search and type in “detect if device is ipad using javascript” then there are pages of possible answers to your question.
I tried the thing is that in the latest version IOS is showing a stupid thing when using userAgent, give it a try if you don’t believe me!
It’s because depending on the iPad, some of the ‘iPad’ may be registered as iOS instead. You need to check with the iPad that you’re having problem first - to make sure how the “userAgent” is registered, then you can target but…
If it doesn’t work on iPad, most likely it’s Safari issue, you should target Safari instead ( or ask the user to get the iPad updated, it’s probably not even iPad issue, it’s something else )
I don’t think is possible with the latest version since the userAgent is returning crap, as always Apple is doing the job right!
Install a Firefox or Opera on iPad and try again. Most likely, it’s browser issue and you won’t have the problem with the new browser.
Then you can change your strategy
I will try …
This is probably long since past being useful to you, but you might’ve gotten this userAgent result if you were testing this with XCode Simulator. Apparently the userAgent string being returned is for your Mac that you’re previewing Simulator on, not the Simulator itself.
Logging window.navigator.userAgent with Simulator results in:
Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_6) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/14.1 Safari/605.1.15
Logging the same call on an actual iPad gets me this:
Mozilla/5.0 (iPad; CPU OS 16_5 like Mac OS X) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/16.5 Mobile/15E148 Safari/604.1

IMAGES
VIDEO
COMMENTS
30. We were able to detect an iPad device using javascript like this: function isDeviceiPad(){. return navigator.platform.match(/iPad/i); } That worked perfectly in detecting iPad devices, but when we checked from an iPad Pro (10.5 inch), it does not detect that it is an iPad. To further investigate, we drilled down into the navigator object ...
One of the methods we can use to detect whether a device is running iOS or not is User-Agent Detection. This method involves examining the user-agent string of the device to determine the operating system and browser being used. We can use the JavaScript navigator.userAgent property to retrieve the user-agent string and then check if it ...
In this article, we'll look at how to detect iPad Pro as iPad with JavaScript. To detect iPad Pro as iPad with JavaScript, we can check various properties of navigator . For instance, we write:
If the page is opened in a web view, then !standalone && !safari is true. Conclusion. To detect iPad or iPhone web view via JavaScript, we check window.navigator.userAgent and window.navigator.standalone.
Essential JavaScript Functions for Detecting User's Device Characteristics. Learn how to detect iOS, Android, fullscreen mode, Firefox, Safari, and more with JavaScript. ... Chrome is used. To that, I would offer a friendly reminder that any browser running on an iPhone or iPad is, unfortunately, still Safari due to Apple's vendor lockdown ...
This JavaScript snippet determines whether the user is using an iPhone, iPad, or iPod. This JavaScript snippet determines whether the user is using an iPhone, iPad, or iPod. Skip to main content. natclark. Posts. Blog. Sharing what I'm creating and thinking as a solo maker. Tutorials. Brief software guides on blockchain, web, and SEO. Web 3.0.
JavaScript: Browser/Client Detection. Detect the browser and client that the end user is using. JavaScript: Check for Mobile Device. How to determine if the browser is on a mobile device like Apple iPhone, Apple iPod, Google Android or Apple iPad including the iPad Pro with iPadOS version 15.x and 16.x or later. JavaScript: Classes
User Agent detection is not a recommended technique for modern web apps. There is a JavaScript API built-in for detecting media. The JavaScript window.matchMedia() method returns a MediaQueryList object representing the results of the specified CSS media query string. You can use this method to detect a mobile device based on the CSS media query.
Anyone here had experience detecting iPad then providing a specific version of a video within the page to only thos ipad users, allowing for stack overflow to take care of other safari, ff, and ie users. The reason i need to do this is to overcome ipad 2's gamma problem when playing video.
This uses a combination of window.navigator.userAgent and window.navigator.standalone.It can distinguish between all four states relating to an iOS web app: safari (browser), standalone (fullscreen), uiwebview, and not iOS.
Enabling inspecting your device from a connected Mac. Before you can connect your device to a Mac to inspect it, you must allow the device to be inspected. Open the Settings app. Go to Safari. Scroll down to Advanced. Enable the Web Inspector toggle. Now, connect the device to your Mac using a cable. In Safari, the device will appear in the ...
Click on the "Safari" menu at the top left corner of the browser. 3. Under the Safari menu, find and click on "Preferences". This will open a pop-up menu. 4. Move your cursor over to the "Security" tab and select it. 5. Now check the box beside "Enable JavaScript" to turn on JavaScript.
Test your JavaScript, CSS, HTML or CoffeeScript online with JSFiddle code editor. Stackoverflow - detect ipad/iphone webview via javascript - JSFiddle - Code Playground JSFiddle
Scroll down until you see "Safari," or any other web browser that you'd like to enable JavaScript in. 3. Tap on the "Safari" icon. 4. Scroll down and tap "Advanced," at the very bottom. 5 ...
Returns a boolean indicating whether the browser is running in standalone mode. Available on Apple's iOS Safari only. When combined with navigator.platform === "MacIntel" iPad's are the only devices that define this property, therefore typeof navigator.standalone !== "undefined" filters out Macs running Safari (touchscreen or not).
To enable JavaScript on your iPad's Safari browser, follow these simple steps: Toggle JavaScript Setting: Within the Safari settings, locate the "JavaScript" option. This setting allows you to control whether JavaScript is enabled or disabled in the Safari browser. Enable JavaScript: Tap on the toggle switch next to the "JavaScript" option to ...
As you can see, the string "iPhone" is contained in that user agent text, so a simple way of searching for the iPhone string in the user agent text with JavaScript looks like this: var isIphone = navigator.userAgent.indexOf("iPhone") != -1 ; That statement makes the variable isIphone true if the string "iPhone" is seen in the browser user agent ...
Enabling Inspection. Across all platforms supporting WKWebView or JSContext, a new property is available called isInspectable ( inspectable in Objective-C). It defaults to false, and you can set it to true to opt-in to content being inspectable. This decision is made for each individual WKWebView and JSContext to prevent unintentionally making ...
Yes, same issue, user agent on iPad(iOS14) is useless when trying to detect mobile Safari browser. it is reported as mac os (not as ipad anymore) Safari. Same thing is happening with Firefox on iPad. I can only detect Chrome b/c it shows up as Mobile Chrome. -
It's because depending on the iPad, some of the 'iPad' may be registered as iOS instead. You need to check with the iPad that you're having problem first - to make sure how the "userAgent" is registered, then you can target but…. If it doesn't work on iPad, most likely it's Safari issue, you should target Safari instead ( or ...
However, as a matter of fact, browsers which match tokens like "iPad", "iOS", "iPhone" .etc don't mean they are mobile safari, maybe they are some other browsers for iOS. For example, UC and QQ browsers in China. All these browsers may use the core of safari and most behaviors are the same as mobile safari, but some not. It sucks. -